Interview Questions
- Home -
- Interview Questions
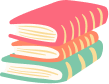
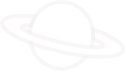

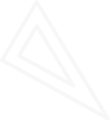
FullStack Java Developer Interview Questions And Answers
1. What is Java?
- • Developed by Sun Microsystems, which is currently owned by Oracle Corporation, Java is a high-level, object-oriented programming language.
2. What distinguishes JRE, JVM, and JDK from one another?
- • A software development kit called JDK (Java Development Kit) is used to create Java apps. The environment in which Java programmes execute is known as the JRE (Java Runtime Environment). The virtual machine used to run Java bytecode is called JVM (Java Virtual Machine).
3. In Java, what distinguishes an interface from an abstract class?
- • Interfaces are not allowed to contain method implementations or instance variables, whereas abstract classes are allowed to have both. Although a class can extend more than one abstract class, it can only implement one interface.
4. In Java exception handling, what does the "finally" section serve as?
- • Whether an exception is raised or not, the "finally" block is utilised to carry out crucial functionality like releasing locks and shutting down resources.
5. In Java, what is method overloading?
- • A class can have more than one method with the same name but distinct parameters thanks to method overloading.
6. In Java, what is method overriding?
- • When a subclass offers a unique implementation of a method that is already offered by its superclass, this is known as method overriding.
7. In Java, what is the distinction between equals() and ==?
- • To see if two references link to the same object, use the "==" operator. The equals() function determines whether two objects are equivalent conceptually based on their contents.
8. Describe Java's access modifiers.
- • There are four access modifiers available in Java: protected, private, default (no modifier), and public.
9. In Java, what is a constructor?
- • An unique technique for initialising things is called a constructor. It lack a return type and maintains the exact identical identity as the class.
10. In Java, what distinguishes static from non-static methods?
- • Static methods can be called without first constructing an instance of the class; they belong to the class rather than any particular instance. Non-static methods are restricted to calling on instances of the class and are associated with them.
11. What distinguishes an ArrayList from a LinkedList?
- • A double linked list is used by LinkedList to store elements, while a dynamic array is used by ArrayList. quick insertion and deletion are provided by LinkedList, whereas quick index access to elements is provided by ArrayList.
12. What is the Java "this" keyword used for?
- • The current instance of a class is referred to by the keyword "this". It provides access to the current object's methods and instance variables.
13. What is the Java "super" keyword used for?
- • The current object's superclass can be referred to using the "super" keyword. It provides access to constructors and superclass functions.
14. In Java, what is method chaining?
- • Programming strategy known as "method chaining," or "fluent interface," involves calling several methods one after the other within a single sentence, each of which returns an object belonging to the same class.
15. What is FullStack development?
- FullStack development is the process of working on the client-side (front end) and server-side (back end) of web applications.
16. What essential elements make up a Java FullStack application?
- • Technologies for the front end (HTML, CSS, JavaScript), the back end (Java, Spring framework), and the database (SQL or NoSQL databases).
17. Describe the MVC design and the Java implementation of it.
- • A software design pattern is called MVC (Model-View-Controller). Java normally uses the domain model or business logic to represent the model, the user interface serves as the view, and the controller mediates between the model and view by handling user input.
18. Describe the Spring Framework.
- • The Java framework Spring is used to create applications at the enterprise level. Complete infrastructure support is offered, including dependency injection and transaction management, and more.
19. In Spring, what does Dependency Injection (DI) mean?
- • One design pattern for handling object dependencies is dependency injection. DI in Spring is accomplished by inversion of control (IoC), in which dependencies are supplied to objects instead of being created by them.
20. Describe the distinction between Spring's constructor and setter injection.
- • Constructor injection employs constructor arguments, whereas setter injection uses setter methods to insert dependencies.
21. RESTful Web Services: What Is It?
- • Designing networked apps using the REST (Representational State Transfer) architectural style is ideal. RESTful web services are APIs that use HTTP methods for CRUD tasks and follow the REST principles.
22. How are RESTful services implemented in Java?
- • By designing controller classes with frameworks such as Spring Boot, you may quickly create RESTful services.
23. Describe hibernation.
- • Java objects can be mapped to database tables and database tables to Java objects using the Hibernate ORM (Object-Relational Mapping) framework.
24. Describe the distinction between Hibernate and JPA.
- • One Java ORM specification is JPA (Java Persistence API), and one implementation of this specification is Hibernate.
25. What benefits does Hibernate offer over JDBC?
- • Compared to JDBC, Hibernate offers simpler database operations, automated object-relational mapping, and higher-level abstractions.
26. What is JDBC?
- • JDBC (Java Database Connectivity) is an API for Java that enables SQL-based database interaction for Java programmes.
27. What is JUnit?
- • Write and execute repeatable tests with JUnit, a Java unit testing framework.
28. Describe the idea behind aspect-oriented programming, or AOP.
- • The AOP programming paradigm separates cross-cutting issues from the primary business logic in order to promote modularity.
29. Describe Java annotations.
- • Annotations offer programme metadata that the compiler or runtime can use for a variety of tasks, including configuration and documentation.
30. What is the Spring @Autowired annotation used for?
- • Spring automatically injects dependent beans into the application using @Autowired, which is used for automated dependency injection.
31. What does Spring MVC's @RequestMapping annotation serve to accomplish?
- • In Spring MVC controllers, @RequestMapping is used to map web requests to particular handler methods.
32. What is the use of Spring MVC's @PathVariable annotation?
- • @PathVariable is used to map values to method parameters in controllers by extracting them from the URI template.
33. What is CORS? How should a Spring Boot application handle CORS?
- • Browsers use a security feature called CORS (Cross-Origin Resource Sharing) to limit cross-origin HTTP queries. You can define a WebMvcConfigurer bean or use the @CrossOrigin annotation to configure CORS in Spring Boot.
34. What is JSON?
- • Structured data can be represented using a lightweight data-interchange format called JSON (JavaScript Object Notation). As an alternative to XML, it is frequently used for data transmission between a server and a web application.
35. What does Spring's @Transactional annotation serve as?
- • In Spring applications, @Transactional is used to define the boundaries of transactions, guaranteeing that a collection of operations is handled as a single transaction.
36. In the spring, what is a bean?
- • An object under the control of the Spring IoC container is called a bean in Spring. The container configures, instantiates, and assembles it.
37. In Java, what distinguishes an abstract class from an interface?
- • Java interfaces specify a contract that classes must adhere to, although abstract,classes can contain method implementations and cannot be instantiated directly.
38. Describe the idea of object-oriented programming's SOLID principles.
- • The term SOLID stands for Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. These are the five design principles. These guidelines are meant to improve the readability, adaptability, and maintainability of software designs.
39. What is the Java'static' keyword used for?
- • In Java, members (variables and methods) that belong to the class are declared using the'static' keyword, not instances of the class. Without launching an instance of the class, they can be reached by using the class name.
40. What is overloading a method?
- • A class can have numerous methods with the same name but distinct parameters thanks to Java's method overloading feature.
41. What is method overriding?
- • A Java subclass can give a particular implementation of a method that is already specified in its superclass by using method overriding.
42. In Java, what is a constructor?
- • When a class instance is formed in Java, a specific kind of method called a constructor is immediately invoked. The object state is initialised using it.
43. What is the Java 'final' keyword used for?
- • When used to classes, the 'final' keyword in Java is used to declare constants, prevent method overriding, and prevent subclassing.
44. What is a Java servlet?
- • A servlet is a class in Java that answers to HTTP requests and expands the functionality of servers.
45. What is a JSP?
- • JavaServer Pages, or JSP, is a technology that allows Java programmers to construct dynamic web pages. It permits the embedding of Java code and specific preset actions into HTML pages.
46. What is a web application session?
- • A web application session is a means of preserving state in between HTTP queries made by the same client. It enables the storing and retrieval of data across queries.
47. What is the Java equals() function used for?
- • In Java, you may compare the equality of two objects using the equals() function. Usually, classes override it to offer unique equality checks.
48. What is a pattern of design? Provide an illustration.
- • In software design, a design pattern is a generic, reusable answer to a frequently occurring issue within a specific environment. The Singleton pattern, which guarantees that a class has just one instance and offers a global point of access to it, is one example.
49. What distinguishes a Java Linked List from an Array List?
- • A double linked list is used to implement LinkedList, whereas a dynamic array is used to implement Array List. Quick random access is offered by Array Lists, whereas quick insertion and deletion are provided by Linked Lists.
50. What distinguishes an interface in Java from an abstract class?
- • An interface can only have abstract methods; an abstract class in Java can have both abstract and concrete methods. Although a class can extend more than one abstract class, it can only implement one interface.
51. What does the Java "volatile" keyword mean?
- • The "volatile" keyword in Java means that multiple threads will be modifying the value of the variable. It guarantees that modifications done by a single thread are observable by other threads.
52. Why is the Java "synchronised" keyword used?
- • The "synchronised" keyword is used to construct a vital part that is only accessible by one thread at a time in Java. It contributes to thread safety by blocking concurrent access to common resources.
53. What does Java's "transient" keyword mean?
- • The "transient" keyword in Java means that a variable shouldn't be serialised when the object that contains it is serialised.
54. What does a Java lambda expression mean?
- • A shorthand for an anonymous function in Java that may be passed around as an object and run at a later time is a lambda expression.
55. What is the objective of the Java Stream API?
- • The Java Stream API offers a fluid interface for handling object collections in a functional programming manner. It enables the processing of element streams through operations including filtering, mapping, and reduction.
56. What is the function of the "default" keyword in Java interfaces?
- • The "default" keyword in Java interfaces is used to specify a method's default implementation. It permits interfaces to change without interfering with already-implemented versions.
57. What does Java's "try-with-resources" statement mean?
- • In Java, the 'try-with-resources' statement is used to automatically close resources (like streams) that are used inside the try block. Even in the event of an exception, it makes sure that resources are appropriately closed.
58. What does Java's'super' keyword serve as?
- • The superclass of the current object is referred to in Java using the'super' keyword. It can be used to access superclass members and invoke constructors and functions of superclasses.
59. What is the Java "this" keyword used for?
- • The current object instance is referred to in Java using the 'this' keyword. It has the ability to call methods, retrieve instance variables, and send the current object as an argument.
60. What is a race condition? How do you prevent it in Java?
- • A race condition occurs when the outcome of a program depends on the timing or interleaving of multiple threads. Race conditions can be prevented in Java by using synchronization mechanisms like synchronized blocks, locks, or atomic operations to ensure that critical sections are executed atomically.
61. What is garbage collection in Java?
- • Garbage collection in Java is the process of automatically reclaiming memory occupied by objects that are no longer in use. It frees up memory and helps prevent memory leaks.
62. What do Java's "break" and "continue" statements accomplish?
- • In Java, the 'break' statement is used to prematurely end a loop or switch statement, but the 'continue' statement is utilised to skip the remainder of the current loop iteration and continue on to the next one.
63. What distinguishes an application server from a web server?
- • While an application server handles business logic, runs applications, and communicates with databases, a web server manages HTTP requests and responses and serves static content.
64. Describe the distinction between servlets' forward and redirect methods.
- • Redirecting is done on the client-side, instructing the client to submit a new request to a different URL, whereas forwarding is done on the server-side, moving the request to another internal servlet or resource.
65. What's a pattern of design? What are some popular design patterns that you may mention?
- • A design pattern is a repeatable fix for a typical software design issue. Design patterns like Singleton, Factory, Observer, Strategy, and MVC are frequently utilised.
66. What does Spring's @Component annotation serve to accomplish?
- • To enable automatic detection and configuration by Spring's component scanning system, a Java class can be marked as a bean using the @Component annotation.
67. What does Spring's @Repository annotation serve to accomplish?
- • In Spring, a class can be marked as acting as a repository by using the @Repository annotation; this is usually used for database activities. Better exception handling and translation are made possible by this specialisation of the @Component annotation.
68. What does Spring's @Service annotation mean?
- • Spring uses the @Service annotation to signify that a class offers a business service. It helps to organise and make the purpose of the annotated class clear. It is a specialisation of the @Component annotation.
69. What is Spring Boot?
- • Spring Boot is a prescriptive framework designed to make creating Spring applications easier. It reduces the requirement for manual setup by automatically configuring various components and providing default configurations.
70. Tell me about an ORM tool and why you use it.
- • Data from object-oriented programming languages is mapped to relational databases using an ORM (Object-Relational Mapping) tool. By offering an object-oriented interface and abstracting database interaction, it streamlines database operations.
71. What is JPA and how is Hibernate related to it?
- • A specification for relational data management in Java applications is called JPA (Java Persistence API). One implementation of the JPA specification that offers functionalities beyond those specified in the specification is called Hibernate.
72. What are the benefits of using JPA over JDBC for database access?
- • JPA provides a higher level of abstraction, automatic mapping between objects and database tables, support for object-oriented queries, and improved portability across different database systems compared to JDBC.
73. What is the purpose of the @Transactional annotation in Spring Data JPA?
- • The @Transactional annotation in Spring Data JPA is used to demarcate transaction boundaries for methods that interact with the database. It ensures that the operations within the annotated method are executed within a single transaction.
74. Describe aspect-oriented programming (AOP) and how it is used in the Spring framework.
- • The AOP programming paradigm enables the modularization of issues that cut across multiple domains, including security, transaction management, and logging. Applying aspects to target components in Spring allows you to decouple these concerns from the core application logic.
75. In Spring AOP, what is the function of the @Aspect annotation?
- • In Spring AOP, a class is defined as an aspect by using the @Aspect annotation, which captures cross-cutting concerns. It is used in conjunction with other annotations, such as @Before, @After, or @Around, to indicate where in the application guidance should be applied.
76. What does a Spring AOP pointcut mean?
- • A Spring AOP pointcut is an expression that indicates the application's join places where guidance ought to be applied. It lays forth the standards for choosing which techniques or classes the advise belongs in.
77. What is the JoinPoint object's function in Spring AOP?
- • In Spring AOP, a program's execution point—typically the execution of a method—is represented by the JoinPoint object. It offers metadata, including the method name, arguments, and target object, regarding the execution context.
78. What does a proxy object in Spring AOP mean?
- • A proxy object in Spring AOP is an object that is created dynamically and is used to intercept calls to methods on the destination object. It permits the application of recommendations and overlapping issues without changing the code of the target item.
79. In Java, what distinguishes compile-time from runtime polymorphism?
- • Method overloading and overriding allow for compile-time polymorphism, where the compiler uses the method signature to determine which method to call. On the other hand, runtime polymorphism is accomplished by method overriding and is determined at runtime by the object's true type.
80. Describe the idea behind Spring's autowiring technology.
- • Autowiring is a way to automatically insert dependencies into Spring beans. By enabling Spring to automatically detect and wire dependencies based on specific rules, like by type or by name, it removes the need for explicit bean configuration.
81. How many distinct kinds of autowiring modes does Spring support?
- • Spring supports 'no', 'byName', 'byType', 'constructor', and 'autodetect', among other variants. How Spring resolves dependencies and injects them into beans is determined by these modes.
82. What is the @Autowired annotation used for in Spring?
- • Spring uses the @Autowired annotation to automatically inject dependencies into Spring beans. It can be used in a Spring bean's fields, constructors, setter methods, or configuration methods.
83. What is the use of the @Qualifier annotation in Spring?
- • When more than one bean of the same type is declared inside the Spring application context, the @Qualifier annotation in Spring is used to distinguish between them. It usually works best when combined with @Autowired to indicate which bean to inject.
84. What is the Spring @Value annotation used for?
- • In Spring beans, values can be injected from property files, environment variables, or other sources using the @Value annotation. It permits the configuration of bean properties to be externalised.
85. In Spring MVC, what does the @RestController annotation mean?
- • In Spring MVC, the @RestController annotation combines the @Controller and @ResponseBody annotations for simplicity. It is employed in the development of RESTful web services that provide data in XML or JSON formats.
86. What distinguishes @RestController from @Controller in Spring MVC?
- • @RestController is used to define controllers that handle requests for RESTful web services and return data directly in the response body, whereas @Controller is used to define MVC controllers that handle web requests and return views.
87. How does Spring MVC's @ResponseBody annotation work?
- • Spring MVC uses the @ResponseBody annotation to specify that a controller method's return value should be serialised straight to the HTTP response body. It is usually used to build web services that use REST in conjunction with @RestController.
88. What is the use of Spring MVC's @RequestBody annotation?
- • In a controller method, the @RequestBody annotation is used to link the request body to a method parameter. It is employed to manage incoming data in JSON or XML formats, among others.
89. What is the purpose of Spring MVC's @PathVariable annotation?
- • In Spring MVC, values from the URI path are extracted and bound to method parameters in controller methods. It is frequently employed to extract dynamic URL segments.
90. What is the use of the @RequestParam annotation in Spring MVC?
- • In Spring MVC, the @RequestParam annotation is used to link method parameters in a controller method to query parameters that are extracted from the request URL. It is employed to manage incoming query strings or data from HTML forms.
91. What does Spring MVC's DispatcherServlet do?
- • In Spring MVC, the front controller that takes incoming HTTP requests and forwards them to the relevant controller for processing is called the DispatcherServlet. It serves as a Spring MVC application's entry point for managing web requests.
92. What is the purpose of the Spring MVC ViewResolver interface?
- • The ViewResolver interface is used to resolve the logical view names that controller functions return to the actual view implementations. Flexible mapping of view names to view templates, like JSP files or Thymeleaf templates, is made possible by it.
93. What is the use of the @ModelAttribute annotation in Spring MVC?
- • In Spring MVC, model attributes that are included in the model and supplied to the view for rendering are bound to method parameters using the @ModelAttribute annotation. It is frequently used to add more info to the screen or prepopulate form fields.
94. Describe Spring Security and its key features.
- • Spring Security is an adaptable and strong framework for access control and authentication for Java applications. Protection against typical security threats, session management, authorization (access control), and authentication (login/logout) are some of its primary features.
95. What is Cross-Site Request Forgery (CSRF) protection and how does Spring Security manage it?
- • CSRF protection is a security feature that stops unauthorised users from acting on behalf of authenticated users. By creating and validating CSRF tokens, which are included in forms and requests to confirm the legitimacy of the request, Spring Security offers protection against cross-site request fraud.
96. What is CORS and how is it handled by Spring Security?
- • Browsers use a security feature called CORS (Cross-Origin Resource Sharing) to limit cross-origin HTTP queries. By enabling the creation of CORS policies to regulate which origins and HTTP methods are permitted to access resources, Spring Security offers support for CORS...
97. What is the objective of Spring Boot Actuator?
- • Spring Boot Actuator is a collection of features and tools that are ready for production use and are supplied by Spring Boot to help with the management and monitoring of Spring applications. It has endpoints for metrics, environment data, health checks, and more.
98. What is CSRF protection, and how does Spring Security handle it?
- • CSRF (Cross-Site Request Forgery) protection is a security mechanism used to prevent unauthorized actions on behalf of authenticated users. Spring Security provides CSRF protection by generating and validating CSRF tokens, which are included in forms and requests to verify the authenticity of the request.
99. What is CORS, and how does Spring Security handle it?
- • CORS (Cross-Origin Resource Sharing) is a security feature implemented by browsers to restrict cross-origin HTTP requests. Spring Security provides CORS support by allowing configuration of CORS policies to control which origins are allowed to access resources and which HTTP methods are allowed..
100. What is the purpose of Spring Boot Actuator?
- • Spring Boot Actuator is a set of production-ready features and tools provided by Spring Boot for monitoring and managing Spring applications in production. It includes endpoints for health checks, metrics, environment information, and more.
FullStack Python Developer Interview Questions And Answers
1. What is Flask?
- • Flask is a Python web framework that is lightweight. It can be scaled up to complex applications and is made to be quick and simple to start using.
2. Describe the distinction between Django and Flask:
- • Django is a full-stack web framework, whereas Flask is a tiny web framework. While Django has more built-in features and conventions, Flask is more flexible and minimalist, giving developers more freedom to make decisions.
3. Describe Django:
- • Django is a high-level Python web framework that promotes efficient development and logical, clear design. The "batteries-included" concept is adhered to, and functionalities like routing, authentication, and an ORM are included.
4. What is Django ORM?
- • Django ORM, or Object-Relational Mapping, is a built-in Django functionality that enables programmers to use Python objects to communicate with databases. It facilitates database work by offering an abstraction layer over database tables and queries.
5. What is SQLAlchemy?
- • SQLAlchemy is an Object-Relational Mapper (ORM) and popular Python SQL toolkit that offers an expressive and versatile means of interacting with databases. It frequently works with Flask to do database operations.
6. What is Web Server Gateway Interface (WSGI)?
- • WSGI is a specification for a common interface that connects web servers to Python web applications or frameworks. Interoperability between various web servers and applications is made possible by it.
7. Why are virtual environments used in Python?
- • Python virtual environments are used to segregate environments for Python projects, enabling independent dependencies between projects without interfering with one another.
8. In Python, how do you set up a virtual environment?
- • The Python virtual environment can be established by utilising the venv package. Take `python -m venv myenv} as an example.
9. What is a pip?
- • The Python package installer is called pip. Installing and managing Python packages from the Python Package Index is done with it (PyPI).
10. How can I use pip to install a package?
- • `pip install package_name} is the command to use when installing a package using pip. Take `pip install flask` as an example.
11. Describe PEP 8.
- • The Python Enhancement Proposal, or PEP 8, offers recommendations for producing legible and consistent Python code. It addresses things like code structure, indentation, and naming conventions.
12. What does Python's `__init__.py` file serve as?
- • In Python, a package is defined in the `__init__}.py file. It may have initialization code that runs when the package is imported, or it may be empty.
13. What is a Python decorator?
- • A decorator is a function that accepts an input from another function and outputs a new, more functional version of that function. Decorators are frequently used to provide functions or methods—like logging, caching, or authentication—behavior.
14. What is a Python generator?
- • A generator in Python is a function that uses the `yield} keyword to produce a succession of values. Generators are useful for efficiently generating lengthy sequences of values without storing them in memory and allow for lazy evaluation.
15. What does Python's `with` statement serve as?
- • In Python, the `with` statement is used to make sure that certain resources, such file handles or databases, are correctly handled and cleaned up.
16. What does Python's `__name__} variable serve as?
- • The name of the current module is stored in a unique variable in Python called `__name__}. {__name__} is set to '__main__' when a Python script executes as the main programme.
17. Why does Python classes have the `__init__} method?
- • In Python classes, the __init__ method is a unique method known as the constructor. It is automatically invoked upon the creation of a new instance of the class and is used to initialise the state of the object.
18. What is the `super()` function in Python used for?
- • In Python, the `super()` function can be used to invoke superclass functions from within a subclass. Invoking the `__init__} method of the superclass is frequently utilised to initialise inherited attributes.
19. What does Python methods' `self` parameter serve as?
- • In Python methods, the `self` parameter refers to the current instance of the class. Within the class, it is used to access and change instance variables and methods.
20. What do Python's `*args` and `**kwargs` parameters serve to accomplish?
- • In Python, functions can take a variable number of positional and keyword arguments, respectively, using the `*args` and `**kwargs} parameters.
21. What is Flask-WTF?
- • Flask-WTF is an extension for Flask that offers Python form rendering and validation interaction with WTForms, a flexible framework. It makes handling forms and validating them in Flask applications easier.
22. What is Jinja2?
- • Jinja2 is a state-of-the-art, Python templating engine that is pleasant to designers. It has capabilities like template inheritance, macros, and filters and is used in Flask to generate dynamic HTML output.
23. What is a Flask blueprint?
- • A Flask blueprint is a modular method of grouping and organising similar routes, templates, and static files. In Flask apps, blueprints facilitate code organisation and reuse.
24. What is the aim of Flask-Migrate?
- • Alembic is a database migration tool for SQLAlchemy, and Flask-Migrate is an extension for Flask that offers integration with Alembic. It makes database schema modifications and version control easier and streamlines database schema migrations in Flask apps.
25. What does a Flask session mean?
- • A Flask session is a mechanism to save user-specific data over the course of several HTTP requests. It is frequently used for user authentication and user preference storage and enables data persistence across queries.
26. What is the goal of the Flask-RESTful extension?
- • Flask-RESTful is an extension that makes it easier to create RESTful APIs. It makes handling request parsing, serialising responses, and establishing API resources in Flask apps easier.
27. Describe Django REST Framework:
- • Django REST Framework is a robust and adaptable set of tools for creating Web APIs in the Django programming language. It offers a collection of reusable parts, including as views, authentication, permissions, serializers, and authentication.
28. What is the Django template language?
- • The Django template language is a markup language that is lightweight and is utilised in Django to construct dynamic HTML templates. It enables the creation of dynamic web pages through the addition of variables, control structures, and template inheritance.
29. What is a Django ModelForm?
- • A Django ModelForm is a helper class that takes an existing Django model and builds a form from it. For model instances, it streamlines the process of generating HTML forms by handling form validation and rendering automatically.
30. What is Django Admin?
- • Django Admin is a built-in Django tool that offers an online interface for Django model and data management. Based on the model definitions, it automatically creates an admin interface that makes CRUD operations on model objects simple.
31. What is a framework of hooks into Django's request/response processing called Django middleware?
- • It is a low-level, lightweight plugin system for Django applications that modify the request or response globally.
32. What does mean?
- • A Django context processor is a function that adds information to the context of each time a Django template renders. They serve the purpose of granting all templates in the application access to particular data.
33. What are Django signals?
- • Django signals are a way to let detached apps know when specific actions take place in other parts of the programme. They enable communication between various application components that are loosely connected.
34. For what purpose does Django employ its `reverse} function?
- • Django views' URLs are generated using the function, depending on their names and optional parameters. In Django apps, it enables uniform and DRY URL setup.
35. What is Django's ORM and how does it work?
- • Django's ORM (Object-Relational Mapper) is a powerful feature that allows developers to interact with databases using Python objects. It provides an abstraction layer over database tables and queries, allowing for easy database operations using Python syntax.
36. What is Django's QuerySet?
- • Django's QuerySet is a powerful API for querying the database and retrieving objects. It allows for chaining of query operations, such as filtering, ordering, and limiting, and provides lazy evaluation for efficient database access.
37. What is the purpose of the prefetch_related function in Django?
- • By prefetching and caching related objects in memory, Django's `prefetch_related` method helps to optimise database searches. It enhances performance by lowering the quantity of database queries needed to obtain related objects.
38. What is the purpose of the select_related function in Django?
- • To optimise database searches, Django's `select_related` method retrieves related items with just one JOIN transaction. It enhances performance by lowering the quantity of database queries needed to access linked objects.
39. What is the built-in authentication framework of Django?
- • It is a feature that comes pre-installed and offers user authentication and authorization features right out of the box. Support for groups, permissions, session management, and user authentication is included.
40. What is the function of the `settings.py` file in Django?
- • The `settings.py` file in Django is a configuration file that holds configurations and settings for Django projects. It contains configuration options for installed apps, middleware, static files, databases, and more.
41. What is the purpose of the middleware framework in Django?
- • Lightweight plugin system that adjusts the request or response globally in Django applications is the middleware framework provided by Django. It enables the application of cross-cutting considerations, such authentication, logging, or CORS, to all requests and answers.
42. Why is the INSTALLED_APPS property in Django used?
- • The list of strings that makes up Django's `INSTALLED_APPS` option contains the names of all Django apps that are enabled for the current Django project. It contains both bespoke applications created for the project and built-in Django applications.
43. Why are urlpatterns used in Django?
- • The list of URL patterns defined in a Django application's `urls.py` file is called `urlpatterns` in Django. Every pattern in a URL has a corresponding view function.
44. Describe Django's template language and its operation.
- • Django's template language is a markup language that is lightweight and is used to define dynamic HTML templates within the Django framework. It enables the creation of dynamic web pages through the addition of variables, control structures, and template inheritance. The Django template engine is used to render templates.
45. Describe and explain Django's Cross-Site Request Forgery (CSRF) protection.
- • This security feature guards against CSRF attacks by appending a special token to every form submission. To make sure the request came from the same location, it verifies the token on the server-side.
46. Describe and explain the operation of the Django migration framework.
- • Database schema migrations in Django applications are made possible via the built-in migration mechanism of Django. In order to maintain consistency between the database schema and the models, it automatically creates migration files based on model changes and applies them to the database.
47. What is the static files framework in Django and how does it function?
- • One feature that comes pre-installed in Django applications is the static files framework, which enables providing static files like CSS, JavaScript, and pictures. For arranging and providing static files from the `STATICFILES_DIRS} and `STATIC_URL` settings, it contains settings and conventions.
48. Describe and explain the operation of Django's built-in session framework.
- • This feature enables the saving of session data across HTTP requests. It supports custom session backends and has backends for many storage solutions, including file-based, database-backed, and in-memory sessions.
49. What is the caching framework in Django and how does it operate?
- • The caching framework in Django is an integrated feature that facilitates the caching of data in Django applications. It supports custom cache backends and has backends for several caching choices, including file-based, database-backed, and in-memory caching.
50. Describe and explain the operation of the Django middleware framework.
- • The middleware framework of Django is a set of lightweight plugins that alters the request or response globally in Django applications. It enables the application of cross-cutting considerations, such authentication, logging, or CORS, to all requests and answers.
51. Describe the Python Global Interpreter Lock (GIL):
- • A mutex that guards access to Python objects and stops multiple native threads from running Python bytecode at the same time is the GIL in Python. It may have an effect on how well multithreaded Python programmes run.
52. In Python, what are decorators? Give an illustration.
- • Python decorators are functions that alter the actions of other methods or functions. The @ symbol and the name of the decorator function are used to identify them. Def my_decorator(func): print("Something is happening before the function is called.") is an example of how to write a function.
- print("Something happening after the function is called.")
- wrapper @my_decorator in return
- print("Hello!") say_hello() def say_hello()
53. Describe how Python classes use the `__init__} method.
- • Python classes have a unique method called `__init__} that is used to initialize new instances of the class. It is used to build up the object's initial state and attributes and is called automatically upon the creation of a new instance.
54. In Python, what is monkey patching?
- • In Python, the term "monkey patching" describes the process of dynamically adding or changing code while it's running. Without changing the modules' or classes' original source code, developers can add functionality to them or change existing ones.
55. Why are `**kwargs} and `*args} used in Python function definitions?
- • In Python function declarations, positional arguments ({*args}) and keyword arguments ({**kwargs}) are used to accept a variable number of parameters. They make functions more adaptable and capable of taking varying quantities of arguments.
56. What does Python's `__name__} variable serve as?
- • The name of the current module is stored in a unique variable in Python called `__name__}. Upon importation
57. Describe the distinction between Python's `__str__} and `__repr__} methods.
- • To return a string representation of an object meant for end users, utilize Python's `__str__} function. For debugging purposes, the `__repr__} method is used to return a clear string representation of an object.
58. What does Python's `__doc__} attribute serve as?
- • In Python, the docstring (documentation string) of a function, module, class, or method is stored in the unique `__doc__} property. An object's {.__doc__} attribute can be used to retrieve it.
59. Describe how to utilize list comprehensions in Python.
- • List comprehensions in Python make it easy to generate lists by allowing you to iterate over an iterable and apply an expression to each element. These are made up of square brackets holding a phrase and a `for} clause, which may or may not be followed by more `for} or `if} clauses.
60. In Python classes, what is the use of the `__slots__} attribute?
- • In Python classes, the `__slots__} attribute is used to declare attributes explicitly that a class may have. By prohibiting the establishment of `__dict__} and `__weakref__} attributes for class instances, it enables memory optimization.
61. Describe the objective of Python's `property` function.
- • In Python, properties are defined on classes using the `property` function, which permits restricted access to instance attributes. For a property, it permits the definition of getter, setter, and deleter methods.
62. In Python, what is a context manager? Give an illustration.
- • In Python, an object used for resource management inside a context is called a context manager. It makes automatic resource allocation and release possible. Using the `with} statement as an example, we have: with open('file.txt', 'r') as f: data = f.read()
63. What is the Python logging module used for?
- • Python's `logging` module offers Python programs an adaptable and strong logging infrastructure. It enables the logging of messages at various severity levels (DEBUG, INFO, ERROR) to various destinations (files, streams, etc.).
64. Describe how Python iterators employ the `__next__} and `__iter__} methods.
- • To retrieve the subsequent item in an iterator, utilize Python's `__next__} function. The iterator object itself is returned via the `__iter__} method. When combined, they enable the `for} loop to iterate over custom objects.
65. In Python, what is a generator? Give an illustration.
- • In Python, a generator is a function that, rather of using the `return` keyword, yields an iterator (a generator iterator). It permits the lazy creation of a series of values. For instance:
- def my_generator(): produce 1 output Two-fold yield 3 gen = print(next(gen)) my_generator() # 1 print(next(gen)) is the output. #Result: 2
66. What in Python is a coroutine?
- • A Python coroutine is a function that uses the `await` keyword to halt and resume execution at specific moments. It can be scheduled concurrently with other coroutines and is usually used for asynchronous programming.
67. What does Python's `asyncio` module serve as?
- • Python's `asyncio` module supports event loop management and asynchronous input/output. It enables the use of coroutines and event loops to implement concurrent and asynchronous programming.
68. Describe how to utilize Python's `async` and `await` keywords.
- • To define asynchronous functions in Python, often known as coroutines, use the `async` keyword. To pause an asynchronous function's execution until a result is available, use the `await` keyword. When combined, they make Python asynchronous programming possible.
69. In Python, what is a lambda function? Give an illustration.
- • In Python, an anonymous function declared with the `lambda} keyword is called a lambda function. Usually, it is applied to brief, straightforward tasks that are performed just once. Add = lambda x, y: x + y is an example.
- print(add(2, 3)) # 5 70 is the output.
70.Describe the objective of Python's `map} function.
- • To apply a function to each item in an iterable and produce a new iterable with the results, utilize Python's `map` function. It is an inbuilt function that is frequently combined with other functions, such as lambda functions.
71. What is Python's `filter` function used for?
- • An iterable's elements can be filtered using Python's `filter` function according to a specified function (predicate). The elements for which the function returns {True} are contained in the iterator that is returned.
72. Describe the function of Python's `reduce` function.
- • To reduce an iterable to a single value, apply a function with two arguments cumulatively, working from left to right, on its items using Python's `reduce` function. In Python 3, it is a component of the `functools` module.
73. What is the function of the Python `collections` module?
- • Specialized container datatypes (e.g., {namedtuple}, `deque}, {Counter{, {OrderedDict}) are available in Python's `collections` module as substitutes for the built-in container types ({list}, `tuple{, `dict{). For particular use scenarios, it provides more capability and performance enhancements.
74. What is the function of the Python `itertools` module?
- • In Python, functions for constructing and manipulating iterators and iterables are available in the `itertools` package. Among other things, it has functions for creating permutations, combinations, and combinations with replacements.
75. What is the function of the Python `functools` module?
- • Python's `functools` module offers operations and higher-order functions on callable objects. It has several functions, including {partial}, `reduce}, {lru_cache}, and `total_ordering}.
76. What is the Python `os` module used for?
- • Python's `os` module has functions for working with files and directories, managing processes, and setting environment variables in order to communicate with the operating system. Platform-independent file and directory operations are supported.
77. What is the Python `sys` module used for?
- • Python's `sys` module gives you access to system-specific functions and parameters, including standard I/O streams, command-line arguments, and the version of the Python interpreter. Low-level system configuration and interactions are supported.
78. What is the Python `json` module used for?
- • JSON (JavaScript Object Notation) data encoding and decoding capabilities are available in the Python `json` module. It enables the conversion of Python objects into and out of the JSON format.
79. What is the function of the Python `datetime` module?
- • Classes like `datetime}, `date{, `time}, and `timedelta} are available for working with dates and times in Python through the `datetime` module. Date and time values can be formatted, manipulated, and mathematical operations performed on them.
80. What is the Python `re` module used for?
- • Python's `re` module offers functionality for regular expression manipulation. Regular expressions can be used to perform pattern matching, searching, and substitution operations on strings.
81. What is the Python `pickle` module used for?
- • Python's `pickle` module has functions for both serializing and deserializing objects in Python. It makes it possible to pickle Python objects by converting them to byte streams and to reconstitute Python objects from a byte stream (unpickling).
82. What does the `shutil` module in Python do?
- • The Python `shutil` module provides high-level file operations, such as copying, relocating, and erasing files and directories. It makes it possible to manipulate folders and files on any platform.
83. What does the `subprocess` module in Python do?
- The `subprocess` module in Python enables simpler the process to create new processes, connects to their input/output/error streams, and gets their return codes. It makes it possible to communicate with the system shell and run external commands.
84. What is the purpose of the `logging` module in Python?
- • The `logging` module in Python provides a flexible and powerful logging framework for Python applications. It allows for logging messages to different destinations (e.g., files, streams) at different levels of severity (e.g., DEBUG, INFO, ERROR).
85. What is the purpose of the `unittest` module in Python?
- • The `unittest` module in Python provides a framework for writing and running unit tests for Python code. It allows for the creation of test cases, test suites, and assertions to verify the correctness of code behavior.
86. Why is there a `mock` module in Python?
- • The `mock` module in Python allows you to define mock behavior and create mock objects for testing. To isolate and test particular code segments, it enables the simulation of objects and functions.
87. What is the function of the Python `asyncio` module?
- • The Python `asyncio` module supports event loop management and asynchronous I/O. It enables the use of coroutines and event loops to implement concurrent and asynchronous programming.
88. What is the function of the Python "typing" module?
- • The Python "typing" module supports type hints and type checking in Python code. To make code more readable and maintainable, it enables the annotation of variables, return types, and function signatures.
89. Why is the `requests` module in Python used?
- • The `requests` module in Python is used to facilitate HTTP requests and web API interaction. It enables handling of answers, submitting HTTP requests, and utilizing sessions and cookies.
90. What is the function of the Python `beautifulsoup4} module?
- • The module offers assistance with parsing XML and HTML texts. It enables data extraction, HTML and XML element manipulation, and document tree navigation and search.
91. Why is the `pandas` library in Python used?
- • The `pandas` library in Python is used to work with structured data (such as tabular data and time series data) by offering intuitive, high-performance data structures and data analysis capabilities. It makes data cleaning, analysis, and manipulation possible.
92. What is the Python `numpy` library used for?
- • Python's `numpy` library supports mathematical operations on arrays and matrices as well as numerical calculations. It makes random number generation, linear algebra operations, and mathematical function computing efficient..
93. What is the function of the Python `matplotlib` library?
- • The Python `matplotlib` library offers assistance in producing static, interactive, and animated visualizations. Plotting graphs, charts, histograms, and other visualizations is possible with it.
94. What is the rationale behind the Python `scikit-learn` library?
- • It offers assistance with machine learning algorithms, data pretreatment, and model assessment. Building and refining machine learning models for clustering, regression, classification, and other applications is made possible by it.
95. What does Python's `tensorflow` package serve as?
- • Deep learning model construction and training are supported by Python's `tensorflow` module. It enables the use of models for inference, automatic differentiation, and neural network construction and training.
96. What is the Python `keras` library used for?
- • Based on top of `tensorflow`, the Python `keras` library offers a high-level neural networks API. With an intuitive and flexible interface, it enables the construction and training of deep learning models.
97. What is the function of the Python "flask" library?
- • The Python "flask" library offers a simple web framework for creating web applications in Python. Routing, handling of requests, rendering of templates, and other web development tasks are supported.
98. Why is the `django` library in Python used?
- • The `django` library in Python offers a full-stack web framework for using Python to create web applications. It has functions like an administration interface, routing, routing tables, ORMs, and template rendering.
99. What is the aim of the Python `sqlalchemy` library?
- • The Python `sqlalchemy` library offers support for utilizing an ORM (Object-Relational Mapper) to work with SQL databases. It enables creating database models, running queries, and utilizing Python objects to communicate with databases.
100. What is the purpose of the `alembic` library in Python?
- • The `alembic` library in Python provides support for database migrations in Python applications using SQLAlchemy. It allows for defining and applying database schema changes in a version-controlled and automated manner.
Full Stack MEAN Developer Interview Questions And Answers
1. Explain the MEAN stack architecture and how each component (MongoDB, Express.js, Angular, Node.js) interacts with the others.
- • The MEAN stack consists of MongoDB (database), Express.js (back-end framework), Angular (front-end framework), and Node.js (runtime environment). MongoDB stores data, Express.js handles server-side logic and routing, Angular manages the client-side UI, and Node.js executes server-side JavaScript, facilitating seamless communication between the components via HTTP requests.
2. What is MongoDB? How does it differ from traditional relational databases?
- • MongoDB is a document-oriented, NoSQL database that stores information in documents that resemble JSON. Its schema-less architecture, which eliminates the need for complicated joins and specified schemas, allows for shorter development cycles, scalability, and flexible data structures compared to standard relational databases.
3. Describe the role of Mongoose in a MEAN stack application.
- • The Mongoose library for Node.js is an ODM (Object Data Modelling) tool for MongoDB. It improves productivity, data modelling, and consistency in a MEAN stack application by offering a defined schema, validation, middleware, and simple interaction with MongoDB.
4. What is Express.js? How does it handle routing in a Node.js application?
- • Express.js is a simple Node.js web application framework. Route definitions, HTTP methods, and middleware functions are used to handle routing. Node.js allows for organised routing and request processing through the use of routes, which are defined using `app.get()`, `app.post()`, etc.
5. How do you handle authentication and authorization in a MEAN stack application?
- • Authentication and authorization are commonly handled using middleware like Passport.js in Express.js. Passport.js provides authentication strategies (e.g., local, JWT) and authorization checks, ensuring secure access to routes based on user roles and permissions.
6. Explain the concept of middleware in Express.js and give examples of middleware functions.
- • Middleware in Express.js are functions that intercept and modify request and response objects. Examples include logging middleware (`morgan`), authentication middleware (e.g., Passport.js), error-handling middleware (`express-validator`), and custom middleware for specific application logic.
7. What is Angular? Describe the key features and advantages of using Angular in front-end development.
- • A front-end framework for creating dynamic web apps is called Angular. Two-way data binding, component-based architecture, dependency injection, routing, forms handling, and potent templating are some of the key characteristics that offer an organised and scalable front-end development method with increased developer productivity.
8. How does Angular handle data binding? Explain one-way and two-way data binding.
- • Angular supports both one-way (from component to view) and two-way (bidirectional between component and view) data binding. One-way binding uses interpolation (`{{ data }}`) or property binding (`[property]="data"`), while two-way binding uses `[(ngModel)]`, allowing data synchronization between component and view.
9. What are Angular directives? Give examples of built-in and custom directives.
- • Angular directives are markers on DOM elements that trigger specific behaviors or functionalities. Built-in directives include `ngIf`, `ngFor`, `ngClass`, etc., while custom directives can be created for reusable UI components or behaviors (e.g., `appHighlight`, `appDraggable`).
10. Describe the Angular component lifecycle hooks and their purposes.
- • Angular component lifecycle hooks include `ngOnInit`, `ngOnChanges`, `ngOnDestroy`, etc. They allow developers to tap into key lifecycle events of Angular components for initialization, data changes, cleanup, and integration with external services, providing control over component behavior.
11. What is dependency injection in Angular? How does it improve code maintainability and scalability?
- • Dependency injection (DI) in Angular is a design pattern for injecting dependencies into components, services, and modules. It improves code maintainability by promoting modular, testable, and reusable code, and scalability by facilitating the management of dependencies and their lifecycles.
12. Explain the role of Angular services and when to use them in a MEAN stack application.
- • Angular services are singleton objects used for encapsulating business logic, data fetching, and communication with external services. They promote code reuse, separation of concerns, and modular architecture in MEAN stack applications, making them ideal for shared functionalities and data management.
13. What is Node.js? What distinguishes it from more conventional server-side languages like PHP or Java?
- • Based on the V8 JavaScript engine seen in Chrome, Node.js is a JavaScript runtime environment. Unlike more traditional server-side languages like PHP or Java, it enables developers to run JavaScript on the server. Because Node.js is event-driven and single-threaded, it is incredibly effective at managing I/O-bound tasks.
14. Describe the event-driven, non-blocking architecture of Node.js and its advantages.
- • Node.js uses an event-driven, non-blocking I/O model, where I/O operations like file system access or network requests are handled asynchronously. This architecture allows Node.js to handle multiple concurrent requests efficiently without blocking the execution of other tasks, leading to improved performance and scalability.
15. How does Node.js handle asynchronous operations? Give examples of asynchronous programming patterns.
- • Node.js handles asynchronous operations using callbacks, promises, and async/await. Callbacks are traditional but can lead to callback hell. Promises provide a more structured approach for handling asynchronous operations, while async/await offers cleaner syntax for writing asynchronous code.
16. Explain the concept of streams in Node.js and how they can be used for efficient data processing.
- • Streams in Node.js are objects used for reading or writing data in chunks, which is especially useful for processing large datasets or handling I/O operations efficiently. Streams can be readable, writable, or duplex (both readable and writable), offering flexibility for data processing tasks.
17. What are RESTful APIs? How do you design and implement RESTful APIs in a MEAN stack application?
- • RESTful APIs follow a set of principles for designing scalable and interoperable web APIs using standard HTTP methods (GET, POST, PUT, DELETE). In a MEAN stack application, Express.js is commonly used to design and implement RESTful APIs for CRUD operations, data retrieval, and manipulation.
18. How do you handle database operations (CRUD) using Node.js and MongoDB in a MEAN stack application?
- • Database operations (CRUD - Create, Read, Update, Delete) using Node.js and MongoDB are performed using Mongoose ODM for MongoDB. Mongoose provides methods for creating models, querying data, updating documents, and deleting records in MongoDB from Node.js.
19. Describe the deployment process for a MEAN stack application, including server setup, database configuration, and front-end deployment.
- • The deployment process involves setting up a production server for Node.js (e.g., using Nginx or PM2), configuring MongoDB for production use, and deploying the front-end Angular application (built using Angular CLI) to a web server or cloud platform (e.g., AWS, Heroku).
20. How do you optimize the performance of a MEAN stack application, considering factors like code optimization, caching, and database indexing?
- • Performance optimization involves techniques like code minification, bundling, caching (e.g., Redis), database indexing, server-side rendering (SSR), lazy loading, AOT (Ahead-of-Time) compilation, and using CDNs for assets to improve load times, responsiveness, and scalability.
21. What is Angular CLI? How do you use it to generate components, services, and modules in an Angular application?
- • Angular CLI (Command Line Interface) is a powerful tool for scaffolding and managing Angular projects. It allows developers to generate components, services, modules, directives, pipes, and more using simple CLI commands like ng generate component, ng generate service, etc., streamlining the development process.
22. Explain the concept of routing in Angular and how to implement lazy loading for modules.
- • Routing in Angular allows navigation between different views (components) in a single-page application (SPA). Lazy loading is a technique where modules are loaded on-demand, improving initial load times and optimizing resource usage. In Angular, lazy loading is implemented by defining routes with loadChildren in the routing configuration.
23. What is NgRx? How does it facilitate state management in Angular applications?
- • NgRx is a state management library for Angular applications based on Redux principles. It provides tools like Actions, Reducers, Selectors, and Effects for managing application state in a predictable and centralized way, enhancing scalability, testability, and maintainability of Angular applications.
24. Describe the concept of server-side rendering (SSR) in Angular and its benefits.
- • Server-side rendering (SSR) in Angular involves rendering the initial HTML on the server before sending it to the client, improving page load times and SEO performance. SSR ensures faster perceived load times and better search engine indexing, enhancing user experience and discoverability of Angular applications.
25. What is TypeScript? How does it enhance the development experience in Angular applications?
- • TypeScript is a superset of JavaScript that enhances it with additional capabilities like enums, interfaces, and static typing. It offers greater IDE support, code readability and maintainability, type checking, and easier code navigation to improve the development experience with Angular applications.
26. Explain the concept of observables in Angular and how they are used for handling asynchronous data streams.
- • Observables in Angular are a powerful way to handle asynchronous data streams and events. They support features like cancellation, retrying, and error handling, making them ideal for handling HTTP requests, event handling, and data streaming in Angular applications with RxJS library integration.
27. How do you handle form validation in Angular, both template-driven and reactive forms?
- • Template-driven forms use directives like ngModel and template-driven validation attributes (e.g., required, minlength) for form validation. Reactive forms use form controls and validators from @angular/forms for validation logic in TypeScript files.
28. What are Angular pipes? Give examples of built-in and custom pipes and their use cases.
- • Angular pipes are used for data transformation in templates. Built-in pipes include DatePipe, UpperCasePipe, LowerCasePipe, etc. Custom pipes can be created for specific data transformations like formatting currency, filtering data, or sorting
29. Explain the concept of AOT (Ahead-of-Time) compilation in Angular and its advantages.
- • AOT compilation in Angular converts Angular templates and components into optimized JavaScript during build time, improving application performance, reducing bundle size, and catching template errors early in the development process.
30. How do you handle internationalization (i18n) and localization (l10n) in Angular applications?
- • Angular provides built-in support for internationalization (i18n) and localization (l10n) through the @angular/localize package. Developers can use the i18n attribute and localization files (*.xlf) for translating text and supporting multiple languages in Angular applications.
31. What is Angular Material? How do you integrate Angular Material components into an Angular application?
- • The UI component library for Angular applications is called Angular Material. To improve UI consistency and user experience, it offers pre-built, customisable components like buttons, forms, dialogues, etc. Installing the @angular/material package and importing the necessary modules will integrate Angular Material.
32. Describe the role of WebSockets in real-time communication and how to implement them in a MEAN stack application.
- • WebSockets enable full-duplex, real-time communication between a client and server over a single TCP connection. In a MEAN stack application, WebSockets can be implemented using libraries like socket.io for bidirectional communication, chat applications, real-time updates, etc.
33. What is JSON Web Token (JWT) authentication? How do you implement JWT authentication in a MEAN stack application?
- • Information can be safely transmitted between parties as a JSON object by using JSON Web Token (JWT) authentication. To ensure secure user authentication and authorization in a MEAN stack application, JWT authentication can be implemented utilising middleware such as jsonwebtoken in Node.js for token generation and verification.
34. Explain the concept of microservices architecture and how it can be applied in a MEAN stack environment.
- • Building apps as a group of discrete, autonomous services that interface with one another using APIs is known as microservices architecture. For scalability, flexibility, and easier maintenance, each component in a MEAN stack environment—MongoDB, Express.js, Angular, and Node.js—can be modularized into microservices.
35. How do you handle data caching in a MEAN stack application to improve performance?
- • Data caching in a MEAN stack application can be implemented using caching strategies like Redis or Memcached. Cached data can be stored temporarily in memory or disk to reduce database queries, improve response times, and enhance overall application performance.
36. Describe the role of Docker in containerizing and deploying MEAN stack applications.
- • Docker is used for containerizing MEAN stack applications, providing a lightweight, portable, and scalable environment for application deployment. Docker containers encapsulate application code, dependencies, and configurations, enabling consistent deployment across different environments.
37. What is serverless computing? How can you implement serverless functions in a MEAN stack application?
- • A cloud computing architecture known as "serverless computing" allows cloud providers to automatically scale resources in response to demand and manage infrastructure. Serverless functions (such as AWS Lambda and Azure Functions) can be used in a MEAN stack application to manage particular activities or endpoints without the need to manage server infrastructure, making scalable and reasonably priced solutions possible.
38. Explain the concept of progressive web apps (PWAs) and how to develop a PWA using the MEAN stack.
- • Progressive Web Apps (PWAs) are web applications that offer a native app-like experience with features like offline access, push notifications, and fast loading. To develop a PWA using the MEAN stack, you can leverage Angular's service workers for offline support, manifest files for app installation prompts, and Angular Universal for server-side rendering to improve performance and SEO.
39. How do you perform unit testing and end-to-end testing in a MEAN stack application?
- • Unit testing in a MEAN stack application involves testing individual components, services, and modules using testing frameworks like Jasmine, Mocha, or Jest. End-to-end testing simulates user interactions and application flows using tools like Protractor or Cypress to ensure functionality across the entire application.
40. What is MongoDB Atlas? How does it simplify database management and deployment in MEAN stack projects?
- • MongoDB Atlas is a cloud-based database service that simplifies database management and deployment for MongoDB in MEAN stack projects. It offers automated backups, scaling, monitoring, and security features, reducing operational overhead and ensuring high availability and performance.
41. Describe the concept of continuous integration (CI) and continuous deployment (CD) in the context of MEAN stack development.
- • Continuous Integration (CI) is the practice of automating code integration and testing in a shared repository, ensuring code quality and early issue detection. Continuous Deployment (CD) automates the deployment process, allowing for frequent, reliable releases of MEAN stack applications, enhancing development speed and reliability.
42. How do you handle security considerations such as XSS (Cross-Site Scripting) and CSRF (Cross-Site Request Forgery) in a MEAN stack application?
- • Security measures for XSS and CSRF in a MEAN stack application include input validation, output encoding, secure cookies, CSRF tokens, and using frameworks/libraries (e.g., Helmet.js, Angular's built-in protections) to mitigate these risks and ensure secure application behavior.
43. Explain the concept of GraphQL and how it differs from RESTful APIs in a MEAN stack environment.
- • GraphQL is a query language for APIs that allows clients to request specific data from the server, reducing over-fetching and under-fetching of data. Unlike RESTful APIs with predefined endpoints, GraphQL enables clients to shape data responses dynamically, enhancing flexibility and efficiency in data retrieval.
44. What are the advantages and disadvantages of using Angular Universal for server-side rendering?
- • Advantages of Angular Universal for server-side rendering include improved SEO, initial load time, and better user experience. Disadvantages may include increased complexity, configuration, and compatibility considerations with third-party libraries/extensions.
45. Describe the role of caching strategies like Redis or Memcached in a MEAN stack application.
- • Caching strategies like Redis or Memcached can be used to cache frequently accessed data, sessions, or API responses, reducing database queries and improving performance. They provide fast, in-memory storage for temporary data storage and retrieval.
46. How do you implement data pagination and sorting in a MEAN stack application with large datasets?
- • Data pagination and sorting in a MEAN stack application can be implemented using client-side pagination (e.g., Angular Material paginator) and sorting features, combined with server-side pagination and sorting APIs (e.g., MongoDB's `skip()` and `limit()` methods) for efficient data retrieval and display.
47. Explain the concept of micro frontends and how it can be implemented in a MEAN stack architecture.
- • Micro frontends is an architectural pattern where a front-end application is decomposed into smaller, independently deployable microservices or components. In a MEAN stack, micro frontends can be implemented using Angular modules, lazy loading, and component-based architecture for modular, scalable front-end development.
48. What are the best practices for securing MongoDB databases in production environments?
- • Best practices for securing MongoDB databases in production environments include enabling authentication, using strong passwords and role-based access control (RBAC), configuring network security (firewalls, SSL/TLS), enabling auditing/logging, and keeping the database and server software updated with security patches.
49. Describe the process of monitoring and logging in a MEAN stack application for performance optimization and debugging.
- • Monitoring and logging in a MEAN stack application involve using tools like Prometheus, Grafana, ELK stack (Elasticsearch, Logstash, Kibana), or cloud-based monitoring services (e.g., AWS CloudWatch) to track application performance, errors, and user interactions, allowing for proactive debugging, optimization, and troubleshooting.
50. How do you handle version control and collaboration using Git in a team-based MEAN stack project?
- • Micro frontends is an architectural pattern where a front-end application is decomposed into smaller, independently deployable microservices or components. In a MEAN stack, micro frontends can be implemented using Angular modules, lazy loading, and component-based architecture for modular, scalable front-end development.
Full Stack MERN Developer Interview Questions And Answers
1. What is the MERN stack?
- • The MERN stack is a group of web development tools based on JavaScript. It consists of Node.js, React.js (or React), Express.js, and MongoDB.
2. Describe MongoDB.
- • MongoDB is a document-oriented data model-based NoSQL database system. Data is kept in adaptable documents that resemble JSON.
3. Describe Express.js.
- • Express.js is a Node.js web application framework intended for creating APIs and online apps.
4. What is React.js?
- • React.js is a JavaScript library created by Facebook that is used to create user interfaces. It is employed in the development of interactive and dynamic web applications.
5. What is Node.js?
- • Based on the V8 JavaScript engine seen in Chrome, Node.js is a server-side JavaScript runtime environment. It enables JavaScript execution on the server for developers.
6. Describe the distinction between React and React.js.
- • React (or React 17 and above) is the most recent version of React that incorporates enhancements and updates to the library. React.js, usually referred to as React, is a JavaScript library for creating user interfaces.
7. What is npm?
- • A package manager for JavaScript programming languages is called npm (Node Package Manager). It is Node.js's default package manager.
8.What does a package.json file mean?
- • For Node.js projects, the package.json file contains metadata in JSON format. It contains scripts, other configuration data, dependencies, and metadata related to the project.
9. Describe Express.js's middleware concept.
- • Functions with access to the request object (req), response object (res), and the following middleware function in the Express.js
10. Describe Mongoose.
- • Mongoose is a Node.js and MongoDB object data modeling (ODM) module. It offers an application data modeling solution based on schemas.
11. What is create-react-app?
- • create-react-app is a command-line tool used to bootstrap React.js applications. It sets up a new React project with a predefined folder structure, configuration files, and development server.
12. What is JSX in React.js?
- • JSX (JavaScript XML) is a syntax extension for JavaScript used with React.js. It allows you to write HTML-like code within JavaScript, making it easier to write and understand React components.
13. Explain the purpose of npm start command.
- • npm start command is used to start a Node.js application. It executes the script specified in the "start" field of the package.json file.
14. What is Redux?
- • Redux is a predictable state container for JavaScript applications, commonly used with React.js for managing application state.
15. What is the React.js useEffect hook used for?
- • React.js's useEffect hook is used to implement side effects in function components. It lets you manually modify the DOM of a React component, fetch data, and create subscriptions.
16. Describe the intent of the npm install command.
- • The package.json file's dependencies are installed using the npm install command. Installing all of the requirements mentioned in the "dependencies" and "devDependencies" sections requires it to read the package.json file.
17. What is React.js routing?
- React.js routing provides a way to switch between several views or components in response to URL changes. It makes it possible for users to move about a React application.
18. Describe the function of React.js's useState hook.
- • seReact.js's state hook is used to give function components state management. In React function components, it facilitates the creation and modification of state variables.
19. What is a React.js application routing library?
- • React Router is a routing library. It enables you to specify paths and navigation in React applications using a declarative syntax.
20. RESTful API: What is it?
- • Representational State Transfer, or RESTful API, is an architectural paradigm used in networked application architecture. For data manipulation, it makes use of common HTTP methods like GET, POST, PUT, and DELETE.
21. How can I deal with mistakes in Node.js?
- • Try-catch blocks in Node.js or the `next} argument in Express.js can be used to transfer errors to the next middleware function in case of an error.
22. What is Redux Thunk?
- • Redux Thunk is a Redux middleware that enables you to create action creators that, rather than returning an action object, return a function. It makes Redux apps capable of asynchronous operations and side effects.
23. What is Redux Saga?
- • Redux Saga is a Redux middleware that makes writing intricate asynchronous logic more synchronous and comprehensible. To manage side effects like data fetching and asynchronous action processing, generator functions are used.
24. What is Express.js Router?
- • It's a middleware that lets you mount route handlers at particular URLs and organize them into distinct modules. It offers an approach to arrange route handlers in applications written in Express.js.
25. What are higher-order components (HOCs) in React.js and how are they used?
- • Higher-order components (HOCs) in React.js are functions that take a component as input and return a new enhanced component with additional functionality. They are used for code reuse, logic abstraction, and adding features like authentication, data fetching, or styling to multiple components.
26. Explain the concept of WebSocket communication and how it differs from HTTP communication.
- • WebSocket communication is a protocol that provides full-duplex, bidirectional communication channels over a single TCP connection, allowing real-time data exchange between clients and servers. Unlike HTTP, which uses request-response model and stateless connections, WebSockets maintain persistent connections for continuous data transmission and server-to-client push notifications.
27. What are microservices and how are they implemented in Node.js applications?
- • Microservices architecture is an approach to building applications as a collection of small, independent services that communicate over lightweight protocols like HTTP or messaging queues. In Node.js applications, microservices can be implemented using frameworks like Express.js for building individual services, which can then be deployed and managed independently.
28. Explain the concept of containerization and its benefits in deploying Node.js applications.
- • Containerization involves packaging applications and their dependencies into lightweight, portable containers that can run consistently across different environments. Docker is a popular containerization platform used for deploying Node.js applications, providing benefits like scalability, isolation, version control, and easy deployment across cloud platforms.
29. What is the role of Redux in React.js applications and how does it manage application state?
- • Redux is a predictable state container for JavaScript applications, commonly used with React.js for managing application state in a centralized store. It follows a unidirectional data flow where actions are dispatched to update the state, and components subscribe to the state changes using mapStateToProps() and mapDispatchToProps() functions.
30. Explain the concept of microservices architecture and its advantages over monolithic architecture in the context of MERN stack applications.
- • An application can be divided into smaller, independent services using microservices architecture, each of which can be created, deployed, and scaled independently. Advantages include scalability, flexibility, technology diversity, easier maintenance, and improved fault isolation compared to monolithic architectures.
31. Discuss the role of WebSockets in real-time communication and how they can be implemented in a MERN stack application.
- • WebSockets enable full-duplex, real-time communication between clients and servers. They can be implemented in a MERN stack using libraries like Socket.io, allowing bi-directional communication for features like chat applications, real-time updates, and notifications.
32. Explain the concept of load balancing and its importance in ensuring scalability and high availability in a MERN stack application.
- • Load balancing distributes incoming traffic across multiple servers (instances) to optimize resource utilization, prevent overloading, and ensure high availability and performance. Use load balancers (e.g., AWS ELB, NGINX) to evenly distribute traffic, handle failovers, and scale horizontally as traffic demands fluctuate.
33. Describe the role of Redux middleware like Redux Thunk or Redux Saga in managing asynchronous actions and side effects in a MERN stack application.
- • Redux Thunk enables handling asynchronous logic in Redux action creators, dispatching multiple actions sequentially or conditionally. Redux Saga provides a more complex solution with generator functions, enabling tasks like complex side effects, async/await behavior, and managing long-running processes in a structured way.
34. Discuss the use of performance monitoring tools (e.g., New Relic, Datadog) in identifying and resolving performance bottlenecks in a MERN stack application.
- • Performance monitoring tools track application performance metrics (response times, CPU/memory usage, database queries) and provide insights into bottlenecks, errors, and inefficiencies. Use these tools for real-time monitoring, alerting, performance profiling, and optimizing critical components for better user experience.
35. What is MongoDB Atlas?
- • MongoDB Atlas is a fully managed cloud database service provided by MongoDB. It allows you to deploy, operate, and scale MongoDB databases in the cloud without the need for manual intervention.
36. Explain the process of internationalization (i18n) and localization in a MERN stack application, including tools and best practices.
- • Internationalization involves designing applications to support multiple languages and regions, while localization adapts content and UI elements for specific locales. Use i18n libraries like react-i18next or i18next for managing translations, locale-specific formatting, date/time formats, and handling language switches based on user preferences.
37. Describe the process of deploying a MERN stack application to a cloud platform like AWS or Azure, including deployment strategies and considerations for scalability.
- • Deploy MERN applications using cloud services like AWS Elastic Beanstalk, Azure App Service, or Docker containers on Kubernetes. Considerations include choosing the right deployment model (single instance, load-balanced, auto-scaling), configuring environment variables, managing dependencies, setting up monitoring/alerting, and ensuring scalability with horizontal scaling options.
38. Explain the concept of load balancing and its importance in ensuring scalability and high availability in a MERN stack application.
- • Load balancing distributes incoming traffic across multiple servers (instances) to optimize resource utilization, prevent overloading, and ensure high availability and performance. Use load balancers (e.g., AWS ELB, NGINX) to evenly distribute traffic, handle failovers, and scale horizontally as traffic demands fluctuate.
39. How would you handle cross-origin resource sharing (CORS) issues in a MERN stack application, and what are the best practices?
- • Configure CORS on the server to allow or restrict cross-origin requests based on origin, methods, and headers. Best practices include specifying allowed origins, using preflight requests for complex requests, setting appropriate headers (Access-Control-Allow-Origin, Access-Control-Allow-Methods), and implementing CSRF protection.
40. Describe the role of Redux middleware like Redux Thunk or Redux Saga in managing asynchronous actions and side effects in a MERN stack application
- • Redux Thunk enables handling asynchronous logic in Redux action creators, dispatching multiple actions sequentially or conditionally. Redux Saga provides a more complex solution with generator functions, enabling tasks like complex side effects, async/await behavior, and managing long-running processes in a structured way.
41. Discuss the use of performance monitoring tools (e.g., New Relic, Datadog) in identifying and resolving performance bottlenecks in a MERN stack application.
- • Performance monitoring tools track application performance metrics (response times, CPU/memory usage, database queries) and provide insights into bottlenecks, errors, and inefficiencies. Use these tools for real-time monitoring, alerting, performance profiling, and optimizing critical components for better user experience.
42. Explain the process of internationalization (i18n) and localization in a MERN stack application, including tools and best practices.
- • Internationalization involves designing applications to support multiple languages and regions, while localization adapts content and UI elements for specific locales. Use i18n libraries like react-i18next or i18next for managing translations, locale-specific formatting, date/time formats, and handling language switches based on user preferences.
43. Discuss the implementation of authentication using OAuth 2.0 in a MERN stack application and the security considerations involved.
- • Implement OAuth 2.0 for authentication using libraries like Passport.js. Security considerations include using secure tokens (JWT), HTTPS/TLS encryption, client authentication, refresh tokens, scope-based access control, token expiration, and secure storage of tokens.
44. How can you handle form submissions in a React.js application?
- • Form submissions in React.js can be handled by using controlled components, where form input values are controlled by React state and updated through onChange handlers. onSubmit event handlers can be used to handle form submissions and send data to the backend.
45. Explain the concept of middleware in Express.js.
- • Middleware in Express.js are functions that have access to the request (req) and response (res) objects in the request-response cycle. They can perform tasks such as logging, authentication, parsing request data, and passing control to the next middleware using next().
46. What is MongoDB Atlas and how does it facilitate MongoDB database management?
- • MongoDB Atlas is a cloud-based database service for MongoDB that provides automated provisioning, scaling, and management of MongoDB databases. It simplifies database administration tasks and offers features like automated backups, monitoring, and security controls.
47. What is MongoDB Atlas?
- • MongoDB Atlas is a fully managed cloud database service provided by MongoDB. It allows you to deploy, operate, and scale MongoDB databases in the cloud without the need for manual intervention.
48. Explain the concept of RESTful APIs and how they are used in a MERN stack application.
- • RESTful APIs follow the principles of Representational State Transfer (REST) for designing networked applications. In a MERN stack application, RESTful APIs are used for communication between the frontend React.js application and the backend Node.js/Express.js server to perform CRUD (Create, Read, Update, Delete) operations on data.
49. What is the role of package.json in a Node.js project?
- • The package.json file in a Node.js project contains metadata about the project, including dependencies, scripts for running tasks (e.g., starting the server), version information, author details, and configuration settings. It is used by Node.js for package management and project configuration.
50. How can you deploy a MERN stack application to a hosting platform like Heroku or Netlify?
- • Deploying a MERN stack application to hosting platforms involves steps such as setting up a production build of the React.js frontend, configuring environment variables, creating a Node.js server for the backend, specifying start scripts in package.json, and deploying the application using platform-specific deployment instructions or CI/CD pipelines.
UI UX Development Interview Questions And Answers
1. What is UI?
- • UI stands for User Interface. It refers to the graphical layout of an application that users interact with.
2. What is UX?
- • UX stands for User Experience. It focuses on enhancing user satisfaction by improving the usability and accessibility of a product.
3. Differentiate between UI and UX.
- • UI is the presentation and interactivity of a product, while UX is the overall experience users have with the product.
4. What is the importance of UI/UX in web development?
- • UI/UX plays a crucial role in web development as it directly impacts user satisfaction, engagement, and retention.
5. Explain the user-centered design approach.
- • User-centered design is an iterative design process that involves understanding user needs, prototyping solutions, and testing with users to ensure the final product meets their requirements.
6. How do wireframes work?
- • Wireframes are simple visual depictions of the design of a web page or application. Without mentioning design components, they describe the interface's organization and capabilities.
7. What tools do you use for creating wireframes?
- • Common tools for creating wireframes include Sketch, Adobe XD, Figma, and Balsamiq.
8. What is a prototype?
- • A prototype is a high-fidelity representation of a product's design and functionality. It allows stakeholders to interact with the product before development begins.
9. Describe the idea of usability testing.
- • The purpose of usability testing is to find usability problems in products by watching users interact with them and getting feedback on how to make them better.
10. In UX design, what are personas?
- • Personas are made-up characters designed to symbolize various user kinds. They aid in designers' comprehension of user objectives, demands, and behaviors.
11. What is responsive design?
- • Responsive design is an approach to web design that ensures a website's layout and content adapt to different screen sizes and devices.
12. Explain the concept of information architecture.
- • The technique of arranging and structuring content on a website or application to improve usability and findability is known as information architecture.
13. What is the importance of typography in UI/UX design?
- • Typography plays a crucial role in UI/UX design as it affects readability, hierarchy, and overall visual appeal.
14. What is a style guide?
- • A style guide is a document that outlines design standards and guidelines for a project. It includes specifications for colors, typography, layout, and other design elements.
15. Explain the concept of accessibility in UI/UX design.
- • Accessibility in UI/UX design refers to designing products that are usable and accessible to people with disabilities. It involves considerations such as screen reader compatibility, color contrast, and keyboard navigation.
16. What are UI patterns?
- • UI patterns are recurrent fixes for typical design issues. They offer tried-and-true guidelines for creating user interface designs.
17. What is A/B testing?
- • A/B testing is a method of comparing two versions of a web page or application to determine which one performs better in terms of user engagement or conversion rate.
18. What is the difference between UI design and graphic design?
- • UI design focuses on designing interfaces for digital products, while graphic design encompasses a broader range of visual communication, including print media and branding.
19. Explain the concept of user flow.
- • User flow refers to the path a user takes to complete a task within a website or application. It involves understanding user actions and interactions from start to finish.
20. What is the importance of user feedback in UX design?
- • User feedback is essential in UX design as it provides valuable insights into user preferences, pain points, and expectations, helping designers make informed decisions.
21. What are UI components?
- • UI components are reusable elements used to build interfaces, such as buttons, forms, navigation menus, and sliders.
22. What is the role of color in UI design?
- • Color in UI design affects visual hierarchy, brand identity, and user emotions. It is used to convey information, create contrast, and establish visual connections.
23. Explain the concept of gestalt principles in UI design.
- • Gestalt principles are a set of laws that describe how humans perceive visual elements as a whole rather than individual parts. They include principles such as proximity, similarity, closure, and continuity.
24. What is the importance of white space in UI design?
- • White space, also known as negative space, is essential in UI design as it improves readability, visual clarity, and overall user experience by reducing clutter and creating breathing room between elements.
25. What is the role of motion design in UI/UX design?
- • Motion design, including animations and transitions, enhances user engagement, communicates feedback, and guides users through interactions in digital products.
26. Describe the idea of affordance in user interface design.
- • The perceived action possibilities of an item or piece are referred to as affordability. It conveys how a UI element's visual cues might be used to engage with users.
27. What is the 8-point grid system in UI design?
- • The 8-point grid system is a design framework that aligns UI elements and spacing on an 8-pixel grid. It helps maintain consistency and alignment throughout the design.
28. Explain the concept of cognitive load in UI/UX design.
- • Cognitive load refers to the mental effort required to process information and perform tasks within a user interface. UI/UX designers aim to minimize cognitive load by simplifying interfaces and reducing complexity.
29. What is a heuristic evaluation in UX design?
- • Heuristic evaluation is a method of evaluating the usability of a product based on a set of predefined usability principles or heuristics. It involves experts identifying usability issues and recommending improvements.
30. What is the importance of user research in UX design?
- • User research is essential in UX design as it helps designers gain insights into user behaviors, needs, and motivations, informing the design process and ensuring products meet user requirements.
31. Describe how progressive disclosure works in user interface design.
- • Progressive disclosure is a design approach that shows users features or information progressively in response to their actions or degree of interaction. It lessens cognitive overload and simplifies complicated interfaces.
32. What is a persona in UX design?
- • A persona is a fictional representation of a target user group based on research and user data. It helps designers empathize with users and design products that meet their needs and preferences.
33. What is the role of usability testing in UX design?
- • Usability testing involves observing users as they interact with a product to identify usability issues and gather feedback for improvements. It helps designers validate design decisions and ensure products are intuitive and easy to use.
34. How does usability testing fit into the process of designing UX?
- • In order to find usability problems and obtain input for enhancements, usability testing entails watching users as they engage with a product. It assists designers in verifying their choices and making sure their creations are user-friendly and intuitive.
35. Describe what usability heuristics are.
- • A set of broad rules or recommendations called usability heuristics is used to assess a product's usability. These encompass concepts like system status visibility, system and real world alignment, and user autonomy and control.
36. Explain the concept of user empathy in UX design.
- • User empathy is the ability to understand and share the feelings, thoughts, and experiences of users. It helps designers create products that meet user needs and provide meaningful experiences.
37. What is the importance of visual hierarchy in UI design?
- • Visual hierarchy refers to the arrangement of visual elements in a way that communicates their importance and relationship to one another. It guides users' attention and helps them navigate interfaces more effectively.
38. In UX design, what function do UI animations serve?
- • User interface animations, such as transitions and microinteractions, improve user experience by giving users visual cues, assisting them with interactions, and fostering a feeling of delight and engagement.
39. Explain the concept of user testing in UX design.
- • User testing involves observing users as they interact with a product to identify usability issues and gather feedback for improvements. It helps designers validate design decisions and ensure products meet user needs and expectations.
40. What is the importance of user feedback in UI/UX design?
- • User feedback provides valuable insights into user preferences, pain points, and expectations. It helps designers make informed decisions and prioritize features or improvements based on user needs.
41. What is the role of usability metrics in UX design?
- • Usability metrics, such as task success rate, time on task, and error rate, are used to measure the effectiveness, efficiency, and satisfaction of a product's usability. They help designers identify areas for improvement and track progress over time.
42. Explain the concept of user-centered design in UX design.
- • User-centered design is an iterative design process that involves understanding user needs, prototyping solutions, and testing with users to ensure the final product meets their requirements. It focuses on designing products that are intuitive, usable, and enjoyable for users.
43. What is the importance of consistency in UI/UX design?
- • Consistency in UI/UX design creates a familiar and predictable user experience, reducing cognitive load and enhancing usability. It involves maintaining consistent design patterns, layouts, and interactions throughout the product.
44. What is the role of usability testing in UX design?
- • Usability testing involves observing users as they interact with a product to identify usability issues and gather feedback for improvements. It helps designers validate design decisions and ensure products are intuitive and easy to use.
45. Explain the concept of user research in UX design.
- • User research is essential in UX design as it helps designers gain insights into user behaviors, needs, and motivations, informing the design process and ensuring products meet user requirements.
46. What is the importance of user personas in UX design?
- • User personas help designers understand the needs, goals, and behaviors of target users. They provide a framework for making design decisions that prioritize user needs and preferences.
47. Describe the idea of "information architecture" in terms of user experience design.
- • Information architecture is the act of arranging and arranging content on a website or application in a way that makes it easier to locate and use. It entails developing logical content hierarchies and unambiguous navigation routes.
48. What is the role of visual design in UI/UX design?
- • Visual design focuses on the aesthetic appeal and visual elements of a product, including color, typography, layout, and imagery. It enhances the overall user experience and brand identity.
49. Explain the concept of user flow in UX design.
- • User flow refers to the path a user takes to complete a task within a website or application. It involves understanding user actions and interactions from start to finish and optimizing the flow for efficiency and usability.
50. How important is user input for UI/UX design?
- • User feedback gives important information about expectations, pain points, and preferences of the user. Based on user needs, it assists designers in making well-informed judgments and prioritizing products or upgrades.
SEO Training Interview Questions And Answers
1. What is SEO?
- • SEO (Search Engine Optimization) is the process of optimizing a website to improve its visibility and ranking in search engine results pages (SERPs).
2. What are the key elements of SEO?
- • Key elements of SEO include on-page optimization, off-page optimization, technical optimization, and content optimization.
3. What is on-page optimization?
- • Optimising individual web pages to raise their search engine ranks is known as on-page optimisation. Meta tags, HTML source code, and content optimisation are all included in this.
4. What is off-page optimization?
- • The term "off-page optimisation" describes the efforts you take to raise your website's search engine ranks without actually being on it. This covers influencer outreach, social media marketing, and backlink building.
5. What is technical optimization?
- • Technical optimisation is the process of optimising a website's technical components in order to boost its search engine exposure. Enhancing a website's mobile friendliness, speed, and structure falls under this category.
6. What is keyword research?
- • Finding the terms and phrases individuals use while looking for information online is known as keyword research. It facilitates the comprehension of user intent and the appropriate optimisation of website content.
7. What are long-tail keywords?
- • Long-tail keywords are more targeted, longer, and more focused keyword phrases that are less competitive. Although there are usually fewer searches, their conversion rates are higher.
8.What is the importance of backlinks in SEO?
- • Backlinks are crucial to SEO since they serve as endorsements from other websites. Search engine rankings and a website's authority can both be raised by high-quality backlinks from reputable websites.
9. What is anchor text?
- The clickable text inside a hyperlink is known as anchor text. Because search engines utilise it to determine the context and relevancy of the linked page, it is crucial for SEO.
10. What are meta tags?
- • Meta tags are HTML elements that provide information about a web page to search engines and website visitors. Common meta tags include meta titles, meta descriptions, and meta keywords.
11. What is a sitemap?
- A sitemap is a file that lists every URL on a website. It facilitates more effective website page discovery and crawling by search engines.
12. What is robots.txt?
- • Robots.txt is a text file that instructs search engine crawlers which pages or sections of a website should not be crawled or indexed.
13. What is canonicalization?
- • Canonicalization is the process of selecting the preferred URL when multiple URLs point to the same content. It helps prevent duplicate content issues and improves SEO.
14. What is Google Search Console (formerly Webmaster Tools)?
- • Google Search Console is a free tool provided by Google that helps website owners monitor, maintain, and troubleshoot their website's presence in Google search results.
15. What is Google Analytics?
- • Google Analytics is a web analytics service that keeps track of and reports on traffic to websites. It offers insightful data on user behaviour, website performance, and conversions.
16. What is a 301 redirect?
- • A permanent redirection from one URL to another is called a 301 redirect. With link equity passed and search rankings maintained, it is utilised to reroute users and search engines from an outdated URL to a fresh URL.
17. What is duplicate content?
- • Duplicate content refers to content that appears on multiple URLs within the same website or across different websites. It can negatively impact SEO by diluting link equity and confusing search engines.
18. What is a nofollow link?
- An hyperlink with the rel="nofollow" attribute instructs search engines not to give the connected page any link equity. Paid links and user-generated content are the two main uses for it.
19. What is a featured snippet?
- • A featured snippet is a synopsis of a Google search result that appears at the top of the user's query. Without needing the user to click on a search result, it seeks to quickly respond to their query.
20. Describe mobile-first indexing.
- • Google prioritises indexing and ranking material from mobile versions of websites. This technique is known as mobile-first indexing. It implies that in order for websites to function properly, they must be mobile-friendly.
21. What is page speed?
- • Page speed refers to the time it takes for a web page to load completely. It is an important ranking factor in Google's search algorithm and affects user experience and bounce rates.
22. What is a schema markup?
- • Schema markup is a code added to a website's HTML to provide search engines with more information about the content. It helps search engines understand the context of the content and display rich snippets in search results.
23. What is a crawl budget?
- • Crawl budget refers to the number of pages Googlebot (Google's web crawling bot) will crawl and index on a website within a given timeframe. It depends on various factors such as website authority, page quality, and server speed.
24. What is density of keywords?
- The percentage of times a keyword occurs on a web page relative to all the words on the page is known as keyword density. Due to worries about keyword stuffing, it is no longer as significant as it previously was as an SEO statistic.
25. What is a 404 error?
- • A 404 error (or Not Found error) occurs when a web page cannot be found by the server. It is important to fix 404 errors to ensure a good user experience and avoid negative impacts on SEO.
26. What is internal linking?.
- • The process of creating a link from one page on a website to another is known as internal linking. It facilitates better user navigation, link equity distribution, and search engines' discovery and crawling of website pages.
27. What is anchor text optimization?
- • Anchor text optimization involves using relevant and descriptive anchor text in hyperlinks to improve search engine rankings for targeted keywords. It is important to use natural and varied anchor text to avoid over-optimization.
28. What is content optimization?
- • Content optimization is the process of improving website content to make it more relevant, valuable, and visible to search engines and users. It includes optimizing content structure, keywords, readability, and multimedia elements.
29. What is local SEO?
- • Local SEO is the process of optimizing a website to improve its visibility in local search results. It includes optimizing Google My Business listings, local citations, and location-based keywords.
30. What is a backlink profile?
- • All of the hyperlinks pointing to a certain website are gathered into a backlink profile. Recognising spammy or low-quality backlinks that could hurt SEO requires careful monitoring and analysis of backlink profiles.
31. What is anchor text diversity?
- • Anchor text diversity refers to using a variety of anchor text types (e.g., exact match, partial match, branded, generic) in backlinks pointing to a website. It helps maintain a natural backlink profile and avoid over-optimization penalties.
32. What is domain authority?
- • Moz created the domain authority statistic, which forecasts a website's position in search engine rankings. It depends on elements including the quantity and calibre of backlinks as well as the general calibre of the website.
33. What is page authority?
- • Page authority is a Moz-created metric that forecasts a website's position in search engine rankings. It is dependent on variables like backlink quantity, backlink quality, and article relevancy.
34. What is cannibalization of keywords?
- • When a website has many pages that focus on the same keyword or keyword phrase, this is known as keyword cannibalization. Lower search rankings can result from it since it can perplex search engines and lessen the authority of particular pages.
35. What is a Google penalty?
- • A Google penalty is a punishment imposed by Google on websites that violate its Webmaster Guidelines. Penalties can result in a drop in search rankings or removal of a website from search results altogether.
36. What is black hat SEO?
- • A black hat Unethical methods of manipulating search engine rankings are referred to as SEO. Penalties or removal from search engine results may follow from engaging in certain activities, which are against search engine guidelines.
37. What is white hat SEO?
- • White hat SEO refers to ethical practices used to improve search engine rankings. These practices comply with search engine guidelines and focus on providing valuable content and a positive user experience.
38. What is gray hat SEO?
- • SEO techniques that lie in the middle of white hat and black hat categories are referred to as "grey hat" SEO. Grey hat strategies can nevertheless be risky and in violation of search engine criteria, even though they are less immoral than black hat strategies.
39. What is Google My Business?
- • A free product from Google that lets companies control their online visibility on Google Maps and Search is called Google My Business. It aids companies in drawing in and interacting with neighbourhood clients.
40.What What are Bing Webmaster Tools?
- • It's a free tool from Microsoft Bing that lets website owners keep an eye on and control their site's visibility in Bing search results. It offers insightful data on search queries, crawl failures, and website performance.
41. How do SEO and SEM differ from one another?
- • SEO (Search Engine Optimization) focuses on improving a website's visibility and ranking in organic (unpaid) search results. SEM (Search Engine Marketing) involves paid advertising strategies to increase a website's visibility in search engine results pages (SERPs).
42. What is a meta description?
- • An HTML attribute that offers a condensed synopsis of the content of a web page is called a meta description. It affects click-through rates and is displayed beneath the meta title in search engine results pages (SERPs).
43. What is a title tag?
- • An HTML element that displays a web page's title is called a title tag. It is displayed as the clickable headline for a specific search result on search engine results pages (SERPs).
44. How does keyword stuffing work?
- • The technique of overusing keywords or keyword phrases in meta tags, anchor text, or website content in an effort to manipulate search engine rankings is known as "keyword stuffing." It is regarded as a black hat SEO tactic and may lead to search engine penalties.
45. What is the Google Knowledge Graph?
- • The Google Knowledge Graph is a knowledge base used by Google to enhance its search engine's understanding of entities and their relationships. It provides information in the form of knowledge panels that appear alongside search results.
46. What is a site audit?
- • A site audit is a comprehensive analysis of a website's performance, structure, and SEO health. It helps identify technical issues, on-page optimization opportunities, and areas for improvement to enhance overall SEO performance.
47. What is a 302 redirect?
- • A temporary redirection from one URL to another is known as a 302 redirect. A 302 redirect does not convey link equity or tell search engines to update their index with the new URL, in contrast to a 301 redirect, which is permanent.
48. What is the difference between follow and nofollow links?
- • Follow links are hyperlinks that search engines can follow to discover and index the linked page, passing link equity in the process. Nofollow links are hyperlinks with a rel="nofollow" attribute that instruct search engines not to follow the link or pass link equity.
49. What is the difference between indexing and crawling?
- • Crawling is the process by which search engine bots (e.g., Googlebot) navigate the web, discover web pages, and collect information about them. Indexing is the process of adding web pages to a search engine's index, making them searchable.
50. What is the difference between a dofollow and a nofollow link?
- • Dofollow links are hyperlinks that search engines can follow to discover and index the linked page, passing link equity in the process. Nofollow links are hyperlinks with a rel="nofollow" attribute that instruct search engines not to follow the link or pass link equity.
Social Media Marketing Interview Questions And Answers
1. What is SMM, or social media marketing?
- • Social media marketing (SMM) is a type of digital marketing that makes use of social media platforms to advertise goods, services, or brands. It also involves interacting with audiences to build brand awareness, improve website traffic, and produce leads or sales.
2. What makes social media marketing crucial for companies?
- • Social media marketing (SMM) is crucial for companies because it offers an affordable means of connecting and interacting with a wide audience, fostering brand loyalty, obtaining client input, boosting website traffic, and boosting sales and conversions.
3. List a few well-known social media sites that are used for advertising.
- • Social media sites, including Facebook, Instagram, Twitter, LinkedIn, Pinterest, YouTube, Snapchat, and TikTok, are widely used for marketing purposes.
4. What are the key components of a successful social media marketing strategy?
- • The key components of a successful SMM strategy include defining clear objectives, identifying target audience segments, creating engaging content, selecting appropriate platforms, implementing a content calendar, monitoring and analysing metrics, and adapting strategies based on insights.
5. How do you define your target audience for social media marketing campaigns?
- • Define the target audience by considering demographics (age, gender, location), psychographics (interests, behaviours, attitudes), and buyer personas based on market research and customer insights.
6. What types of content are effective for social media marketing?
- • Effective content for SMM includes visuals (images, videos, and infographics), engaging text posts, user-generated content, interactive polls or quizzes, behind-the-scenes glimpses, and informative or entertaining content relevant to the audience.
7. How are social media marketing strategies evaluated for effectiveness?
- • The success of SMM campaigns can be measured using key performance indicators (KPIs) such as engagement metrics (likes, comments, and shares), reach and impressions, website traffic from social media, conversion rates, and ROI.
8. What is the role of hashtags in social media marketing?
- • Hashtags are used in SMM to categorise content, increase discoverability, and engage with trending topics or conversations relevant to the brand. They help expand reach and visibility on social media platforms.
9. How do you engage with followers on social media platforms?
- • Engage with followers by responding to comments and messages promptly, asking questions to encourage interaction, sharing user-generated content, running contests or giveaways, and actively participating in conversations related to the brand or industry.
10. What are some best practices for social media advertising?
- • Best practices for social media advertising include defining clear campaign objectives, targeting specific audience segments, using compelling visuals and ad copy, testing different ad formats and creatives, and monitoring and optimising campaigns based on performance metrics.
11. How do you determine the optimal posting frequency on social media platforms?
- • Determine the optimal posting frequency by considering audience preferences, platform algorithms, and content performance. Test different posting schedules and monitor engagement metrics to identify the frequency that resonates best with your audience.
12. What is the role of social media influencers in social media marketing?
- • Social media influencers can play a significant role in SMM by promoting brands or products to their engaged audience, increasing brand visibility, and driving engagement and conversions through sponsored content or collaborations.
13. What are some effective strategies for increasing engagement on social media platforms?
- • Strategies for increasing engagement include posting high-quality and relevant content, using compelling visuals, asking questions, encouraging user-generated content, running contests or polls, and responding to comments and messages promptly.
14. How do you handle negative comments or feedback on social media?
- • Handle negative comments or feedback by acknowledging the concern, apologising if necessary, providing solutions or assistance, taking the conversation offline if appropriate, and addressing the issue transparently and professionally to maintain brand reputation.
15. What is the role of social media analytics in SMM?
- • Social media analytics provide insights into the performance of SMM campaigns and content. They help track key metrics, identify trends, measure ROI, and make data-driven decisions to optimise SMM strategies for better results.
16. How do you optimise social media profiles for search engines?
- • Optimise social media profiles for search engines by using relevant keywords in profile names, bios, and descriptions, including links to your website, and regularly updating profiles with fresh content and updates.
17. What are some common mistakes to avoid in social media marketing?
- • Common mistakes in SMM include neglecting social media profiles, posting irrelevant or low-quality content, being overly promotional, ignoring customer feedback, and failing to track and analyse performance metrics.
18. How do you leverage user-generated content (UGC) in social media marketing?
- • Leverage UGC by sharing customer photos, reviews, testimonials, and other content created by users. It helps build trust, authenticity, and community around the brand, encouraging further engagement and participation.
19. What is the role of storytelling in social media marketing?
- • Storytelling humanises the brand, creates emotional connections with the audience, and conveys brand values, missions, and narratives through compelling stories shared on social media platforms.
20. What are some effective ways to measure ROI in social media marketing?
- • Measure ROI in SMM by tracking key metrics such as engagement rates, website traffic from social media, conversion rates, leads generated, and revenue attributed to social media campaigns. Compare the costs of SMM efforts to the benefits or returns generated to calculate ROI.
21. What is the process for making a social media content calendar?
- • Make a social media content calendar by defining themes and topics for your posts, planning posts for the best times on each platform, including trending hashtags, and making sure your material is a balance between promotional and interesting information.
22. What are some strategies for increasing social media followers?
- • Strategies for increasing social media followers include posting high-quality content regularly, engaging with followers, using relevant hashtags, running contests or giveaways, collaborating with influencers, and promoting social media profiles on other channels.
23. What is the significance of engagement rate in social media marketing?
- • Engagement rate measures the level of interaction your social media content receives relative to the number of followers or impressions. It's significant in SMM as it indicates how well your content resonates with your audience.
24. What are some strategies to encourage user-generated content (UGC)?
- • Strategies to encourage UGC include running contests or challenges, featuring customer testimonials or reviews, creating branded hashtags for users to share content, and actively engaging with and acknowledging users who create content related to your brand.
25. What is the role of social media monitoring in SMM?
- • Social media monitoring involves tracking brand mentions, keywords, and conversations on social media platforms. It's essential in SMM for understanding audience sentiment, identifying trends, and responding promptly to mentions or comments.
26. How do you measure the effectiveness of influencer marketing in SMM?
- • The effectiveness of influencer marketing in SMM can be measured by tracking key metrics such as engagement rates, website traffic from influencer referrals, follower growth, and conversions attributed to influencer campaigns.
27. What is the importance of A/B testing in social media marketing?
- • A/B testing allows marketers to compare different versions of content or ads to determine which performs better. It's important in SMM for optimizing content, ads, and strategies to improve engagement and conversions.
28. What is the role of video content in SMM?
- • Video content is highly engaging and can drive higher levels of engagement and sharing on social media platforms. It's essential in SMM for capturing attention, conveying messages effectively, and increasing reach and engagement.
29. What are some best practices for responding to customer inquiries on social media?
- • Respond promptly, be professional and empathetic, personalize responses, provide solutions or assistance, take conversations offline if necessary, and follow up to ensure the issue is resolved satisfactorily.
30. What is the role of social media advertising in SMM?
- • Social media advertising allows businesses to target specific audiences, promote content, and drive conversions through paid campaigns on platforms like Facebook Ads, Instagram Ads, Twitter Ads, LinkedIn Ads, and Pinterest Ads.
31. What are some common mistakes to avoid in SMM?
- • Common mistakes in SMM include neglecting social media profiles, posting irrelevant or low-quality content, being overly promotional, ignoring customer feedback, and failing to track and analyze performance metrics.
32. What is the role of storytelling in SMM?
- • Storytelling humanizes the brand, creates emotional connections with the audience, and conveys brand values, missions, and narratives through compelling stories shared on social media platforms.
33. How do you optimize social media profiles for search engines?
- • Optimize social media profiles for search engines by using relevant keywords in profile names, bios, and descriptions, including links to your website, and regularly updating profiles with fresh content and updates.
34. What is the significance of social media analytics in SMM?
- • Social media analytics provide insights into the performance of SMM campaigns and content. They help track key metrics, identify trends, measure ROI, and make data-driven decisions to optimize SMM strategies for better results.
35. How do you measure the effectiveness of SMM campaigns?
- • Measure the effectiveness of SMM campaigns using key metrics such as engagement rates, reach and impressions, website traffic from social media, conversion rates, and follower growth.
36. What are some strategies for increasing social media followers?
- • Strategies for increasing social media followers include posting high-quality content regularly, engaging with followers, using relevant hashtags, running contests or giveaways, collaborating with influencers, and promoting social media profiles on other channels.
37. How do you handle negative comments or feedback on social media?
- • Handle negative comments or feedback by acknowledging the concern, apologizing if necessary, providing solutions or assistance, taking the conversation offline if appropriate, and addressing the issue transparently and professionally to maintain brand reputation.
38. What is the role of social media in customer service?
- • Social media provides a direct channel for customers to ask questions, seek assistance, and provide feedback or complaints. It allows businesses to respond promptly, publicly address issues, and resolve customer concerns in real-time.
39. How do you leverage social media for lead generation?
- • Leverage social media for lead generation by creating compelling content, using targeted advertising, providing valuable resources or incentives in exchange for contact information, and nurturing leads through engagement and follow-up.
40. What are some common SMM mistakes to avoid?
- • Common mistakes in SMM include neglecting social media profiles, posting irrelevant or low-quality content, being overly promotional, ignoring customer feedback, and failing to track and analyze performance metrics.
41. What is the role of social media in SEO?
- • Social media indirectly impacts SEO by increasing brand visibility, driving website traffic, and generating backlinks and social signals that can influence search engine rankings.
42. How do you create a social media content calendar?
- • Create a social media content calendar by outlining content themes and topics, scheduling posts at optimal times for each platform, incorporating relevant hashtags and trends, and ensuring a mix of promotional and engaging content.
43. What is the significance of engagement rate in SMM?
- • Engagement rate measures the level of interaction your social media content receives relative to the number of followers or impressions. It's significant in SMM as it indicates how well your content resonates with your audience.
44. What are some strategies to encourage user-generated content (UGC)?
- • Strategies to encourage UGC include running contests or challenges, featuring customer testimonials or reviews, creating branded hashtags for users to share content, and actively engaging with and acknowledging users who create content related to your brand.
45. What is the role of social media monitoring in SMM?
- • Social media monitoring involves tracking brand mentions, keywords, and conversations on social media platforms. It's essential in SMM for understanding audience sentiment, identifying trends, and responding promptly to mentions or comments.
46. How do you measure the effectiveness of influencer marketing in SMM?
- • The effectiveness of influencer marketing in SMM can be measured by tracking key metrics such as engagement rates, website traffic from influencer referrals, follower growth, and conversions attributed to influencer campaigns.
47. What is the importance of A/B testing in SMM?
- • A/B testing allows marketers to compare different versions of content or ads to determine which performs better. It's important in SMM for optimising content, ads, and strategies to improve engagement and conversions.
48. What is the role of video content in SMM?
- • Video content is highly engaging and can drive higher levels of engagement and sharing on social media platforms. It's essential in SMM for capturing attention, conveying messages effectively, and increasing reach and engagement.
49. What are some best practices for responding to customer inquiries on social media?
- • Respond promptly, be professional and empathetic, personalize responses, provide solutions or assistance, take conversations offline if necessary, and follow up to ensure the issue is resolved satisfactorily.
50. What is the role of social media advertising in SMM?
- • Social media advertising allows businesses to target specific audiences, promote content, and drive conversions through paid campaigns on platforms like Facebook Ads, Instagram Ads, Twitter Ads, LinkedIn Ads, and Pinterest Ads.
Google Ads and PPC Interview Questions And Answers
1. What is Google Ads?
- • Google Ads is an online advertising platform where advertisers bid on keywords to display their ads on Google's search results pages and partner websites.
2. Explain the difference between PPC and CPC.
- • The Pay-Per-Click (PPC) advertising model requires advertisers to pay a fee each time a click is made on their advertisement. The actual amount an advertiser pays for each click on their ad is known as CPC (Cost-Per-Click).
3. What are the key components of a Google Ads campaign?
- • The key components include campaigns, ad groups, keywords, ads, and targeting settings.
4. How do you improve Quality Score in Google Ads?
- • Quality Score can be improved by focusing on relevant keywords, ad copy, landing page experience, and click-through rate (CTR).
5. What is the importance of ad extensions in Google Ads?
- • Ad extensions improve ad visibility and provide additional information to users, leading to higher click-through rates and improved ad performance.
6. How do you set up conversion tracking in Google Ads?
- • Conversion tracking is set up by placing a tracking code snippet on the confirmation page that appears after a user completes a desired action, such as making a purchase or filling out a form.
7. Explain the difference between Search Network and Display Network in Google Ads.
- • The Search Network displays text ads on Google search results pages, while the Display Network displays image, video, and text ads on a network of partner websites.
8. How do you target specific locations in Google Ads?
- • Specific locations can be targeted in Google Ads by selecting geographic targeting options such as countries, regions, cities, or radius targeting around a specific location.
9. What is ad rotation in Google Ads?
- • Ad rotation determines how often different ads within an ad group are shown, optimizing for clicks, conversions, or evenly rotating ads.
10. How do you measure the effectiveness of a Google Ads campaign?
- • Effectiveness can be measured using metrics such as click-through rate (CTR), conversion rate, cost per conversion, return on ad spend (ROAS), and Quality Score.
11. What is the purpose of negative keywords in Google Ads?
- • Negative keywords help prevent ads from being triggered by irrelevant search queries, improving ad targeting and reducing wasted ad spend.
12. How do you create a new campaign in Google Ads?
- • To create a new campaign, you need to navigate to the Campaigns tab in Google Ads, click on the "+" button, and follow the prompts to set up campaign settings, ad groups, keywords, and ads.
13. What is ad relevance in Google Ads?
- • Ad relevance measures how closely related your ad is to the keywords you're targeting and the content of your landing page. It affects your Quality Score and ad performance.
14. How do you optimize bids in Google Ads?
- • Bids can be optimized by adjusting keyword bids based on performance data, implementing bid strategies like target CPA or target ROAS, and using bid adjustments for devices, locations, or audiences.
15. What are broad match, phrase match, and exact match keywords in Google Ads?
- • Broad match targets a wide range of variations of a keyword, phrase match targets specific keyword phrases, and exact match targets the exact keyword or close variations.
16. How do you set up remarketing in Google Ads?
- • Remarketing is set up by adding a remarketing tag to your website and creating remarketing lists based on user behavior, such as visitors who viewed specific pages or completed specific actions.
17. What is the purpose of ad scheduling in Google Ads?
- • Ad scheduling allows you to specify certain days of the week and times of day when your ads should be shown, optimizing ad delivery based on when your target audience is most active.
18. How do you create ad groups in Google Ads?
- • Ad groups are created within campaigns by grouping related keywords and ads together. You can create ad groups based on themes, products, or services you want to promote.
19. What is the Google Ads Quality Score?
- • A metric called Quality Score evaluates the quality and relevancy of your landing pages, keywords, and advertisements. It has an impact on cost-per-click (CPC), ad rank, and ad position.
20. How do you use ad scheduling to optimize performance in Google Ads?
- • Ad scheduling allows you to adjust bids or turn off ads during specific times of the day or days of the week when conversion rates are lower or competition is higher.
21. What are the different bidding strategies available in Google Ads?
- • Bidding strategies include manual CPC bidding, automated bidding strategies like target CPA or target ROAS, and enhanced CPC bidding.
22. How do you use ad extensions to improve ad performance in Google Ads?
- • Ad extensions provide additional information like phone numbers, site links, and location information to your ads, improving ad visibility and click-through rates.
23. What is the purpose of ad position in Google Ads?
- • The location of your advertisement on the display network or search results page is referred to as its "ad position." In general, more visibility and clicks come from higher ad placements.
24. How do you measure conversion tracking in Google Ads?
- • Conversion tracking is measured by tracking the number of conversions generated from your ads, such as purchases, form submissions, or phone calls.
25. What is ad relevance and why is it important in Google Ads?
- • Ad relevance measures how closely your ad matches the intent of a user's search query. It's important because higher ad relevance leads to better Quality Scores and lower costs.
26. How do you use ad rotation settings to optimize ad performance in Google Ads?
- • Ad rotation settings allow you to specify how often different ads within an ad group are shown. You can optimize for clicks, conversions, or evenly rotate ads for testing purposes.
27. What is the purpose of keyword match types in Google Ads?
- • Types of keyword match control which searches result in your adverts. There are various match kinds that enable varying levels of targeting, such as phrase match, exact match, broad match, and broad match modifier.
28. How do you use audience targeting in Google Ads?
- • Audience targeting allows you to show your ads to specific groups of people based on their demographics, interests, or behaviors, increasing ad relevance and performance.
29. What is the Google Ads Auction Insights report?
- • The Auction Insights report in Google Ads provides visibility into how your ads are performing compared to competitors in the same auctions, including metrics like impression share and average position.
30. How do you optimize ad copy in Google Ads?
- • Ad copy can be optimized by testing different headlines, descriptions, and calls-to-action to improve click-through rates and ad relevance.
31. What is the difference between automated and manual bidding in Google Ads?
- • Automated bidding strategies use machine learning algorithms to adjust bids based on performance goals, while manual bidding allows advertisers to set bids themselves.
32. How do you use ad scheduling to optimize ad delivery in Google Ads?
- • Ad scheduling allows you to specify certain days of the week and times of day when your ads should be shown, optimizing ad delivery based on when your target audience is most active.
33. What is the purpose of the Google Ads Keyword Planner tool?
- • The Keyword Planner tool helps advertisers research and select keywords for their campaigns by providing keyword ideas, search volume data, and historical performance metrics.
34. How do you optimize landing pages for Google Ads campaigns?
- • Landing pages can be optimized by ensuring they are relevant to the ad copy and keywords, have clear calls-to-action, load quickly, and provide a good user experience on both desktop and mobile devices.
35. What is the Google Ads Display Network?
- • The Google Ads Display Network is a collection of millions of websites, videos, and apps where advertisers can display their ads using targeting options like demographics, interests, and remarketing.
36. How do you use location targeting in Google Ads?
- • Location targeting allows you to show your ads to users in specific geographic locations, such as countries, regions, cities, or custom-defined areas.
37. What are the different ad formats available in Google Ads?
- • Ad formats include text ads, image ads, video ads, responsive ads, and app promotion ads, each optimized for different placements and devices.
38. How do you measure the performance of a Google Ads campaign?
- • Performance can be measured using metrics such as click-through rate (CTR), conversion rate, cost per conversion, return on ad spend (ROAS), and Quality Score.
39. What is the purpose of ad extensions in Google Ads?
- • Ad extensions provide additional information to users, improving ad visibility and click-through rates. Examples include sitelinks, callouts, location extensions, and call extensions.
40. How do you use bid adjustments in Google Ads?
- • Bid adjustments allow you to increase or decrease bids for specific targeting options like devices, locations, ad schedules, or audiences, optimizing bids for different user segments.
41. How do you optimize ad campaigns for mobile devices in Google Ads?
- • Ad campaigns can be optimized for mobile devices by creating mobile-friendly ad copy and landing pages, using mobile-specific ad extensions, and adjusting bids for mobile users.
42. What is the purpose of ad relevance in Google Ads?
- • Ad relevance measures how closely your ad matches the intent of a user's search query. Higher ad relevance leads to better Quality Scores and lower costs.
43. How do you use ad testing to improve ad performance in Google Ads?
- • Ad testing involves creating multiple ad variations and testing them against each other to determine which ones perform best in terms of click-through rates and conversions.
44. How do you use dynamic search ads in Google Ads?
- • Dynamic search ads automatically generate headlines and landing pages based on the content of your website, dynamically matching search queries to relevant pages.
45. What is the purpose of remarketing in Google Ads?
- • Remarketing allows you to show ads to users who have previously visited your website or app, increasing brand awareness and encouraging them to return and complete a desired action.
46. How do you optimize keyword bids in Google Ads?
- • Keyword bids can be optimized by adjusting bids based on performance data, using automated bidding strategies like target CPA or target ROAS, and testing different bidding strategies to maximize ROI.
47. What is the Google Ads Ad Rank formula?
- • Ad Rank is calculated based on bid amount, ad quality (Quality Score), and the expected impact of ad extensions and other ad formats.
48. How do you use ad scheduling to optimize ad delivery in Google Ads?
- • Ad scheduling allows you to specify certain days of the week and times of day when your ads should be shown, optimizing ad delivery based on when your target audience is most active.
49. Why does Google AdWords use frequency capping?
- • Frequency capping enhances user experience by limiting the frequency with which the same user sees your advertising over a predetermined period of time. This helps to avoid ad fatigue.
50. How can campaign performance in Google Ads be measured using conversion tracking?
- • By tracking particular user behaviours, like purchases, sign-ups, or downloads, after your adverts, you can get valuable information into the effectiveness of your campaigns and return on investment.
Email Marketing Interview Questions And Answers
1. What is email marketing?
- • Email marketing is a digital marketing strategy that involves sending emails to a targeted audience to promote products, services, or events, build brand awareness, and nurture customer relationships.
2. What are the benefits of email marketing?
- • Benefits of email marketing include reaching a large audience, cost-effectiveness, easy customization, high ROI, and the ability to track and measure performance metrics.
3. What is an email marketing campaign?
- • An email marketing campaign is a series of targeted emails sent to a specific audience with a predefined goal, such as promoting a product launch, driving sales, or nurturing leads.
4. How do you build an email list for email marketing?
- • You can build an email list for email marketing by collecting email addresses through opt-in forms on your website, social media channels, events, and other marketing channels, ensuring compliance with data protection regulations.
5. What is an email marketing automation?
- • Email marketing automation involves using software to send personalized and timely emails to subscribers based on predefined triggers or actions, such as welcome emails, abandoned cart reminders, and follow-up sequences.
6. What is the CAN-SPAM Act, and why is it important for email marketing?
- • The CAN-SPAM Act is a law that sets rules and regulations for commercial email messages, including requirements for sender identification, opt-out mechanisms, and compliance with unsubscribe requests. It's important for email marketers to ensure legal compliance and maintain trust with subscribers.
7. What are the key metrics to track in email marketing?
- • Key metrics to track in email marketing include open rate, click-through rate (CTR), conversion rate, bounce rate, unsubscribe rate, and overall ROI.
8. How do you improve email deliverability?
- • To improve email deliverability, you can maintain a clean and engaged email list, use a reputable email service provider, personalize emails, avoid spammy content, and monitor email performance metrics.
9. What is A/B testing in email marketing?
- • Email marketers can use A/B testing to test two or more email variations with various audience segments to see which version converts better and has higher open, click-through, and click-through rates.
10. How do you create an effective subject line for email marketing?
- • Effective subject lines for email marketing are concise, clear, and compelling, using personalization, urgency, curiosity, and relevance to capture the recipient's attention and encourage opens.
11. What is the importance of segmentation in email marketing?
- • Segmentation in email marketing involves dividing your email list into smaller, targeted segments based on demographics, behavior, or preferences. It's important for delivering personalized and relevant content, improving engagement, and driving conversions.
12. How do you personalize email marketing campaigns?
- • Email marketing campaigns can be personalized by addressing recipients by name, segmenting lists based on preferences or behavior, dynamically inserting content based on user data, and tailoring messages to specific audience segments.
13. What is the significance of email design in email marketing?
- • Email design in email marketing plays a crucial role in capturing attention, conveying brand identity, and optimizing user experience. It includes elements such as layout, colors, fonts, images, and responsive design for mobile devices.
14. How do you optimize email content for better engagement?
- • Email content can be optimized for better engagement by providing valuable and relevant content, using compelling visuals and calls-to-action, optimizing for mobile devices, and personalizing messages based on recipient preferences.
15. What is the purpose of email automation workflows in email marketing?
- • Email automation workflows in email marketing allow you to set up automated sequences of emails triggered by specific actions or events, such as welcome emails, abandoned cart reminders, or lead nurturing sequences, to streamline communication and drive conversions.
16. What is the best way to assess an email marketing campaign's effectiveness?
- • The success of an email marketing campaign can be measured using key performance indicators (KPIs) such as open rate, click-through rate (CTR), conversion rate, bounce rate, unsubscribe rate, and overall return on investment (ROI).
17. What is the role of segmentation in email marketing?
- • Segmentation in email marketing involves dividing your email list into smaller, targeted segments based on demographics, behavior, or preferences. It allows for personalized and relevant messaging, leading to higher engagement and conversions.
18. How do you handle email unsubscribes in email marketing?
- • In email marketing, unsubscribe requests must be fulfilled quickly and effectively in order to abide by laws like the CAN-SPAM Act. Maintaining a positive sender reputation and subscriber confidence is facilitated by offering a simple and straightforward unsubscribe option.
19. What are the best practices for email deliverability?
- • Best practices for email deliverability include maintaining a clean and engaged email list, using double opt-in confirmation, authenticating your domain with DKIM and SPF records, avoiding spammy content, and monitoring email performance metrics
20. What is the best way to write email subject lines to increase open rates?
- • To optimize email subject lines for better open rates, you can use personalization, urgency, curiosity, and relevance, while keeping them concise and clear. A/B testing different subject line variations can help determine the most effective approach.
21. What is the importance of responsive design in email marketing?
- • Responsive design in email marketing ensures that emails are optimized for various devices and screen sizes, providing a consistent and user-friendly experience for recipients on desktops, smartphones, and tablets.
22. How do you maintain a clean email list in email marketing?
- • To maintain a clean email list in email marketing, regularly remove inactive subscribers, verify email addresses, use double opt-in confirmation, and comply with unsubscribe requests promptly. Keeping your list clean improves deliverability and engagement metrics.
23. What role does personalization play in email marketing?
- • Personalization in email marketing involves tailoring content, subject lines, and offers to individual recipients based on their preferences, behavior, or demographic information. It helps increase engagement, relevance, and conversions.
24. How do you optimize email content for better engagement?
- • To optimize email content for better engagement, focus on providing valuable and relevant content, using compelling visuals, clear calls-to-action, and optimizing for mobile devices. A/B testing can help determine the most effective content strategies.
25. What are the key elements of an effective email marketing strategy?
- • Key elements of an effective email marketing strategy include defining goals and objectives, understanding the target audience, building and maintaining a quality email list, creating compelling content, designing visually appealing emails, and analyzing performance metrics for optimization.
26. What are the different types of email marketing campaigns?
- • Different types of email marketing campaigns include promotional emails, newsletters, transactional emails (order confirmations, shipping notifications), welcome emails, abandoned cart emails, and re-engagement emails targeting inactive subscribers.
27. What is your approach to email marketing bounces?
- • Email bounces in email marketing occur when an email cannot be delivered to the recipient's inbox. Bounces can be classified as soft bounces (temporary issues like a full inbox) or hard bounces (permanent issues like an invalid email address). Monitoring and addressing bounces helps maintain list hygiene and deliverability.
28. How do you measure the success of an email marketing campaign?
- • Key performance indicators (KPIs) including open rate, click-through rate (CTR), conversion rate, bounce rate, unsubscribe rate, and overall return on investment (ROI) can be used to gauge the effectiveness of an email marketing campaign.
29. What is the role of segmentation in email marketing?
- • Segmentation in email marketing involves dividing your email list into smaller, targeted segments based on demographics, behavior, or preferences. It allows for personalized and relevant messaging, leading to higher engagement and conversions.
30. How do you handle email unsubscribes in email marketing?
- • In email marketing, unsubscribe requests must be fulfilled quickly and effectively in order to abide by laws like the CAN-SPAM Act. Maintaining a positive sender reputation and subscriber confidence is facilitated by offering a simple and straightforward unsubscribe option.
31. What are the best practices for email deliverability?
- • Best practices for email deliverability include maintaining a clean and engaged email list, using double opt-in confirmation, authenticating your domain with DKIM and SPF records, avoiding spammy content, and monitoring email performance metrics
32. What are some best practices for crafting email subject lines that increase open rates?
- • While maintaining clarity and conciseness, you can leverage personalisation, urgency, curiosity, and relevance to optimise email subject lines for higher open rates. The best strategy may be ascertained by A/B testing various topic line changes.
33. What is the importance of responsive design in email marketing?
- • Responsive design in email marketing ensures that emails are optimized for various devices and screen sizes, providing a consistent and user-friendly experience for recipients on desktops, smartphones, and tablets.
34. How do you maintain a clean email list in email marketing?
- • To maintain a clean email list in email marketing, regularly remove inactive subscribers, verify email addresses, use double opt-in confirmation, and comply with unsubscribe requests promptly. Keeping your list clean improves deliverability and engagement metrics.
35. What role does personalization play in email marketing?
- • In email marketing, personalisation refers to adjusting offers, subject lines, and content to each receiver individually based on their demographics, interests, and behaviours. Conversions, relevancy, and engagement all go up as a result.
36. How do you optimize email content for better engagement?
- • To optimize email content for better engagement, focus on providing valuable and relevant content, using compelling visuals, clear calls-to-action, and optimizing for mobile devices. A/B testing can help determine the most effective content strategies.
37. What are the key elements of an effective email marketing strategy?
- • Key elements of an effective email marketing strategy include defining goals and objectives, understanding the target audience, building and maintaining a quality email list, creating compelling content, designing visually appealing emails, and analyzing performance metrics for optimization.
38. What are the different types of email marketing campaigns?
- • Different types of email marketing campaigns include promotional emails, newsletters, transactional emails (order confirmations, shipping notifications), welcome emails, abandoned cart emails, and re-engagement emails targeting inactive subscribers.
39. In email marketing, what is your approach to email bounces?
- • Email bounces in email marketing occur when an email cannot be delivered to the recipient's inbox. Bounces can be classified as soft bounces (temporary issues like a full inbox) or hard bounces (permanent issues like an invalid email address). Monitoring and addressing bounces helps maintain list hygiene and deliverability.
40. What is the importance of email segmentation in email marketing?
- • Email segmentation in email marketing involves dividing your email list into smaller, targeted segments based on demographics, behavior, or preferences. It allows for personalized and relevant messaging, leading to higher engagement and conversions.
41. How do you create an effective email marketing campaign?
- • To create an effective email marketing campaign, start by defining your goals and target audience, segmenting your email list, creating compelling content, designing visually appealing emails, optimizing for mobile devices, and testing different elements for optimization.
42. What are the key elements of an effective email subject line?
- • Key elements of an effective email subject line include personalization, relevance, clarity, urgency, and curiosity. A strong subject line captures the recipient's attention and encourages them to open the email.
43. How do you measure the success of an email marketing campaign?
- • The success of an email marketing campaign can be measured using key performance indicators (KPIs) such as open rate, click-through rate (CTR), conversion rate, bounce rate, unsubscribe rate, and overall return on investment (ROI).
44. What are the best practices for designing email templates?
- • Best practices for designing email templates include using responsive design, clear and concise messaging, visually appealing graphics, prominent calls-to-action, optimized for mobile devices, and testing across different email clients.
45. What is the significance of email deliverability in email marketing?
- • Email deliverability in email marketing refers to the ability to successfully deliver emails to recipients' inboxes without being filtered as spam. It's crucial for reaching subscribers, maintaining sender reputation, and maximizing the effectiveness of email campaigns.
46. How do you optimize email content for better engagement?
- • To optimize email content for better engagement, focus on providing valuable and relevant content, using compelling visuals, clear calls-to-action, and optimizing for mobile devices. A/B testing can help determine the most effective content strategies.
47. What are the key elements of an effective call-to-action (CTA) in email marketing?
- • Key elements of an effective call-to-action (CTA) in email marketing include clarity, visibility, relevance, and urgency. A strong CTA encourages recipients to take the desired action, such as making a purchase or signing up for an event.
48. What are the different types of email marketing campaigns?
- • Different types of email marketing campaigns include promotional emails, newsletters, transactional emails, welcome emails, abandoned cart emails, re-engagement emails, and drip campaigns.
49. What is the role of testing and optimization in email marketing?
- • Testing and optimization in email marketing involve experimenting with different elements such as subject lines, content, design, and sending times to identify what resonates best with your audience and improve campaign performance over time.
50. What are the common challenges faced in email marketing, and how do you overcome them?
- • Common challenges in email marketing include low open rates, high unsubscribe rates, poor deliverability, and maintaining subscriber engagement. To overcome these challenges, focus on providing valuable content, segmenting your audience, maintaining list hygiene, and testing and optimizing your campaigns regularly.
Whatsapp Marketing Interview Questions And Answers
1. What is WhatsApp marketing?
- • WhatsApp marketing refers to the use of WhatsApp as a messaging platform to promote products, services, or brands, engage with customers, and drive conversions through targeted communication.
2. How can businesses use WhatsApp for marketing purposes?
- • Businesses can use WhatsApp for marketing by creating business accounts, sending personalized messages to customers, providing customer support, running promotional campaigns, and facilitating transactions through WhatsApp Business API.
3. What are the benefits of WhatsApp marketing?
- • Benefits of WhatsApp marketing include direct and instant communication with customers, high engagement rates, personalization options, cost-effectiveness, and the ability to reach a global audience.
4. What is a WhatsApp Business account?
- • A WhatsApp Business account is a dedicated account for businesses that allows them to communicate with customers on WhatsApp, access business features such as automated messages and labels, and use WhatsApp Business API for advanced functionalities.
5. How can businesses grow their WhatsApp subscriber list?
- • Businesses can grow their WhatsApp subscriber list by promoting their WhatsApp number on their website and social media channels, offering incentives for subscribing, providing valuable content, and engaging with customers proactively.
6. What are WhatsApp Broadcast Lists?
- • WhatsApp Broadcast Lists allow businesses to send messages to multiple recipients at once without creating a group chat. Recipients receive messages individually, maintaining privacy and preventing group chat clutter.
7. How can companies market their products and services using WhatsApp Broadcast Lists?
- • Businesses can use WhatsApp Broadcast Lists for marketing by segmenting their subscriber list based on interests or demographics and sending targeted messages, updates, promotions, and announcements to specific groups of subscribers.
8. What are some best practices for WhatsApp marketing?
- • Best practices for WhatsApp marketing include obtaining explicit consent from subscribers before sending messages, personalizing messages, providing value in each communication, respecting privacy, and responding promptly to customer inquiries.
9. What are WhatsApp Business API and its benefits?
- • WhatsApp Business API is a solution that allows businesses to integrate WhatsApp messaging capabilities into their existing systems and applications, enabling automation, scalability, and advanced features such as chatbots and transactional messaging.
10. How does WhatsApp Business API differ from regular WhatsApp Business accounts?
- • When it comes to features and capabilities, WhatsApp Business API surpasses standard WhatsApp Business accounts. These include automation, chatbots, message templates, interaction with CRM systems, and the capacity to send updates and notifications.
11. Can businesses use WhatsApp for advertising purposes?
- • WhatsApp does not currently support advertising within the app. However, businesses can leverage WhatsApp for marketing through organic communication, customer engagement, and promotional campaigns using features like WhatsApp Business accounts and WhatsApp Business API.
12. How can businesses measure the effectiveness of their WhatsApp marketing efforts?
- • Businesses can measure the effectiveness of their WhatsApp marketing efforts by tracking metrics such as message open rates, response rates, conversion rates, customer feedback, and overall engagement with their WhatsApp messages and campaigns.
13. What are some common challenges faced in WhatsApp marketing?
- • Common challenges in WhatsApp marketing include obtaining and maintaining subscriber consent, managing large subscriber lists, ensuring message deliverability, maintaining user privacy, and staying compliant with WhatsApp's terms of service and regulations.
14. How can businesses maintain customer privacy in WhatsApp marketing?
- • Businesses can maintain customer privacy in WhatsApp marketing by obtaining explicit consent before sending messages, respecting opt-out requests, securing customer data, and adhering to privacy regulations such as GDPR and CCPA.
15. What is the significance of personalization in WhatsApp marketing?
- • In WhatsApp marketing, personalisation refers to adjusting messages to specific receivers according to their interests, actions, and previous communications with the company. It improves marketing campaigns' overall efficacy, relevance, and consumer engagement.
16. How can businesses use WhatsApp for customer support?
- • Businesses can use WhatsApp for customer support by providing a dedicated WhatsApp number for inquiries and assistance, offering timely responses to customer messages, resolving issues efficiently, and using automation for common queries.
17. What are some key features of WhatsApp Business accounts?
- • Key features of WhatsApp Business accounts include creating a business profile with essential business information, using labels and tags for organizing contacts, setting up automated responses and greetings, and accessing messaging statistics.
18. How can businesses leverage WhatsApp groups for marketing purposes?
- • Businesses can leverage WhatsApp groups for marketing purposes by creating groups based on common interests or demographics, sharing valuable content, fostering community engagement, and providing exclusive offers or promotions to group members.
19. What are some best practices for engaging with customers on WhatsApp?
- • Best practices for engaging with customers on WhatsApp include being responsive to messages, providing timely assistance and support, personalizing interactions, sending valuable content, and maintaining a conversational tone.
20. How can businesses integrate WhatsApp with their CRM systems?
- • Businesses can integrate WhatsApp with their CRM systems using WhatsApp Business API, which allows for seamless communication between WhatsApp and CRM platforms, enabling automation, data synchronization, and personalized messaging.
21. Can businesses use WhatsApp for conducting surveys or collecting feedback?
- • Yes, businesses can use WhatsApp for conducting surveys or collecting feedback by sending targeted messages to customers and requesting their input through text, images, or multimedia content. Responses can be collected and analyzed to gather valuable insights.
22. How can businesses ensure message deliverability in WhatsApp marketing?
- • Businesses can ensure message deliverability in WhatsApp marketing by adhering to WhatsApp's guidelines and policies, avoiding spammy content, obtaining subscriber consent, maintaining a positive sender reputation, and sending relevant and valuable messages.
23. How does WhatsApp Business API pricing work?
- • WhatsApp Business API pricing typically involves a combination of setup fees, monthly subscription fees based on the number of active users or messages sent, and variable costs for additional features such as message templates and session messages.
24. What are some creative ways businesses can use WhatsApp for marketing?
- • Creative ways businesses can use WhatsApp for marketing include running interactive contests or quizzes, hosting live Q&A sessions, providing exclusive behind-the-scenes content, offering personalized recommendations, and delivering exclusive offers or promotions.
25. What are WhatsApp message templates, and how can businesses use them?
- • WhatsApp message templates are pre-approved message formats that businesses can use for specific types of communications, such as order confirmations, appointment reminders, or customer service updates. Businesses can use message templates to streamline communication and ensure compliance.
26. How can businesses leverage WhatsApp for event promotion?
- • Businesses can leverage WhatsApp for event promotion by creating event-specific WhatsApp groups or broadcast lists, sharing event details, updates, and reminders, providing exclusive perks or discounts for attendees, and facilitating RSVPs and inquiries.
27. What are some considerations for businesses when using WhatsApp for marketing in different regions or countries?
- • Some considerations for businesses when using WhatsApp for marketing in different regions or countries include understanding cultural norms and preferences, complying with local regulations and data protection laws, respecting language preferences, and adapting messaging strategies accordingly.
28. How can businesses prevent their WhatsApp messages from being marked as spam?
- • Businesses can prevent their WhatsApp messages from being marked as spam by sending relevant and valuable content, obtaining subscriber consent, avoiding excessive messaging frequency, and adhering to WhatsApp's guidelines and policies.
29. What are some common mistakes to avoid in WhatsApp marketing?
- • Some common mistakes to avoid in WhatsApp marketing include sending unsolicited messages, spamming subscribers with irrelevant content, neglecting to obtain subscriber consent, using automation excessively, and not personalizing messages.
30. How can businesses use WhatsApp for lead generation?
- • Businesses can use WhatsApp for lead generation by sharing valuable content that encourages engagement and interaction, offering incentives for subscribing or providing contact information, and using automation to qualify and nurture leads through targeted messaging.
31. What is the difference between WhatsApp Business and WhatsApp Business API?
- • WhatsApp Business is a standalone application designed for small and medium-sized businesses to interact with customers using WhatsApp's features. WhatsApp Business API, on the other hand, is an interface that allows larger businesses to integrate WhatsApp with their existing systems and applications for advanced functionalities.
32. How does WhatsApp verify business accounts?
- • WhatsApp verifies business accounts through a process that involves verifying the business's phone number and confirming its authenticity through documentation or other means. Verified business accounts receive a green checkmark badge, indicating their authenticity to users.
33. Can businesses use WhatsApp for e-commerce transactions?
- • Yes, businesses can use WhatsApp for e-commerce transactions by integrating WhatsApp Business API with their e-commerce platforms, enabling customers to browse products, place orders, make payments, and receive order updates directly through WhatsApp.
34. What are some best practices for engaging customers through WhatsApp status updates?
- • Best practices for engaging customers through WhatsApp status updates include sharing behind-the-scenes content, showcasing new products or services, running limited-time promotions, providing exclusive offers or discounts, and encouraging interaction through polls or questions.
35. How can businesses use WhatsApp for customer relationship management (CRM)?
- • Businesses can use WhatsApp for CRM by integrating WhatsApp Business API with their CRM systems, enabling seamless communication, personalized messaging, automated responses, and tracking customer interactions and preferences.
36. How can businesses encourage customers to contact them on WhatsApp?
- • Businesses can encourage customers to contact them on WhatsApp by prominently displaying their WhatsApp number on their website, social media profiles, and marketing materials, offering incentives for contacting them through WhatsApp, and providing timely responses to inquiries.
37. What are some typical difficulties in WhatsApp marketing?
- • Common challenges in WhatsApp marketing include obtaining and maintaining subscriber consent, managing large subscriber lists, ensuring message deliverability, maintaining user privacy, and staying compliant with WhatsApp's terms of service and regulations.
38. How can businesses measure the effectiveness of their WhatsApp marketing efforts?
- • Businesses can measure the effectiveness of their WhatsApp marketing efforts by tracking metrics such as message open rates, response rates, conversion rates, customer feedback, and overall engagement with their WhatsApp messages and campaigns./li>
39. What are some strategies for improving customer engagement on WhatsApp?
- • Strategies for improving customer engagement on WhatsApp include providing valuable and relevant content, personalizing messages, offering timely assistance and support, running interactive campaigns or contests, and encouraging feedback and interaction.
40. How can businesses ensure message deliverability in WhatsApp marketing?
- • Businesses can ensure message deliverability in WhatsApp marketing by adhering to WhatsApp's guidelines and policies, avoiding spammy content, obtaining subscriber consent, maintaining a positive sender reputation, and sending relevant and valuable messages.
41. How can businesses leverage WhatsApp groups for marketing purposes?
- • Businesses can leverage WhatsApp groups for marketing purposes by creating groups based on common interests or demographics, sharing valuable content, fostering community engagement, and providing exclusive offers or promotions to group members.
42. What are some best practices for engaging with customers on WhatsApp?
- • Best practices for engaging with customers on WhatsApp include being responsive to messages, providing timely assistance and support, personalizing interactions, sending valuable content, and maintaining a conversational tone.
43. What are some creative ways businesses can use WhatsApp for marketing?
- • Creative ways businesses can use WhatsApp for marketing include running interactive contests or quizzes, hosting live Q&A sessions, providing exclusive behind-the-scenes content, offering personalized recommendations, and delivering exclusive offers or promotions.
44. How can companies stop the marking of their WhatsApp communications as spam?
- • Companies can avoid having their WhatsApp messages classified as spam by following WhatsApp's rules and regulations, getting user authorization, offering pertinent and helpful material, and avoiding sending too many messages too often.
45. What are WhatsApp message templates, and how can businesses use them?
- • WhatsApp message templates are pre-approved message formats that businesses can use for specific types of communications, such as order confirmations, appointment reminders, or customer service updates. Businesses can use message templates to streamline communication and ensure compliance.
46. Can businesses use WhatsApp for conducting surveys or collecting feedback?
- • Yes, businesses can use WhatsApp for conducting surveys or collecting feedback by sending targeted messages to customers and requesting their input through text, images, or multimedia content. Responses can be collected and analyzed to gather valuable insights.
47. How can businesses leverage WhatsApp for event promotion?
- • Businesses can leverage WhatsApp for event promotion by creating event-specific WhatsApp groups or broadcast lists, sharing event details, updates, and reminders, providing exclusive perks or discounts for attendees, and facilitating RSVPs and inquiries.
48. What are some considerations for businesses when using WhatsApp for marketing in different regions or countries?
- • Businesses should take into account cultural norms and preferences, adhere to local laws and data protection rules, respect language preferences, and modify messaging methods when utilising WhatsApp for marketing in other regions or nations.
49. What are some common mistakes to avoid in WhatsApp marketing?
- • Some common mistakes to avoid in WhatsApp marketing include sending unsolicited messages, spamming subscribers with irrelevant content, neglecting to obtain subscriber consent, using automation excessively, and not personalizing messages.
50. How can businesses use WhatsApp for lead generation?
- • Businesses can use WhatsApp for lead generation by sharing valuable content that encourages engagement and interaction, offering incentives for subscribing or providing contact information, and using automation to qualify and nurture leads through targeted messaging.
DevOps Interview Questions And Answers
1. What is DevOps?
- • DevOps is a culture, philosophy, and practice that emphasizes collaboration and communication between development (Dev) and operations (Ops) teams, with the goal of delivering software and services more rapidly, reliably, and efficiently.
2. What are the key components of DevOps?
- • The key components of DevOps include culture, automation, measurement, and sharing (also known as CALMS).
3. Explain Continuous Integration (CI).
- • Continuous Integration is the practice of frequently integrating code changes into a shared repository, where automated builds and tests are run.
4. What is Continuous Delivery (CD)?
- • Continuous Delivery is the practice of ensuring that code changes are always in a deployable state, allowing for rapid and reliable releases.
5. Differentiate between Continuous Deployment and Continuous Delivery.
- • Continuous Deployment automatically deploys every code change to production, while Continuous Delivery ensures that code changes are always in a deployable state but requires manual approval for deployment to production.
6. What is Infrastructure as Code (IaC)?
- • Infrastructure as Code is the practice of managing and provisioning infrastructure using machine-readable definition files, allowing for automated infrastructure management.
7. List a few well-known IaC tools.
- • Terraform, AWS CloudFormation, Azure Resource Manager, and Google Cloud Deployment Manager are a few of the well-known IaC products.
8. What is Git and how does DevOps use it?
- • During software development, changes to source code are tracked using the distributed version control system Git. Version control, teamwork, and the implementation of CI/CD pipelines are its uses in DevOps.
9. Explain the concept of "Immutable Infrastructure."
- • Immutable Infrastructure is the practice of never modifying running infrastructure components. Instead, when changes are required, new components are deployed, and the old ones are replaced.
10. Describe Docker and explain how it helps with DevOps procedures.
- • Applications and their dependencies can be packed into lightweight containers using the Docker containerization platform. It makes DevOps processes easier by facilitating easier deployment and scalability, as well as consistency across environments.
11. Describe Kubernetes.
- • The deployment, scaling, and maintenance of containerized applications may be automated with the help of Kubernetes, an open-source container orchestration platform.
12. Describe the deployment of Blue-Green.
- • The Blue-Green Deployment approach involves maintaining two identical production environments, referred to as "blue" and "green," through deployment. There is always just one live environment; the other is for testing and deployment. Deployments with minimal downtime are possible using this method.
13. What is the purpose of a CI/CD pipeline?
- • A CI/CD pipeline automates the steps required to build, test, and deploy software, enabling rapid and reliable delivery of applications.
14. What is the role of configuration management in DevOps?
- • Configuration management automates the provisioning, configuration, and management of infrastructure and application environments, ensuring consistency and reliability.
15. What does "Shift-Left" mean in DevOps?
- • Shift-Left is the practice of integrating security, testing, and other processes earlier in the software development lifecycle, typically during the planning and coding phases, to catch issues earlier and improve overall software quality.
16. What are microservices, and how do they relate to DevOps?
- • Microservices are an architectural approach where applications are composed of small, independently deployable services that communicate through APIs. Microservices align with DevOps principles by enabling teams to independently develop, deploy, and scale services.
17. What is "Monitoring as Code"?
- • Watching as Code is the process of using automation and version control tools to define and manage monitoring setups in addition to application code.
18. Explain the concept of "ChatOps."
- • ChatOps is a collaboration model where development and operations teams use chat tools to automate workflows, share information, and execute tasks in real-time, improving communication and efficiency.
19. What is "Infrastructure as a Service" (IaaS)?
- • Infrastructure as a Service is a cloud computing model where virtualized computing resources are provided over the internet, allowing users to provision and manage infrastructure components such as servers, storage, and networking on-demand.
20. Describe "Platform as a Service" (PaaS) in brief.
- • In the cloud computing paradigm known as Platform as a Service (PaaS), a provider offers a platform that lets users create, execute, and administer applications without having to deal with the hassles of constructing and managing the underlying infrastructure.
21. What are a few critical standards of DevOps culture?
- • Key standards of DevOps culture incorporate coordinated effort, shared liability, mechanization, consistent improvement, and client centricity.
22. Make sense of the "Three Different ways" of DevOps.
- • The "Three Different ways" of DevOps, as characterized by Quality Kim in "The Phoenix Undertaking," are stream, criticism, and nonstop learning. They address the fundamental standards and practices of DevOps.
23. What is "Canary Sending"?
- • Canary Sending is an organization technique where another rendition of an application is bit by bit carried out to a subset of clients or servers, considering testing and checking before a full rollout.
24. What is "Disarray Designing"?
- • Disarray Designing is the act of purposefully bringing disappointments and unsettling influences into a framework to test its flexibility and recognize shortcomings, eventually further developing in general framework unwavering quality.
25. Make sense of the idea of "DevSecOps."
- • DevSecOps is the act of coordinating security rehearses into the DevOps interaction, guaranteeing that security is focused on all through the product improvement lifecycle.
26. What is "Component Hailing"?
- • Highlight Hailing is a strategy used to empower or impair highlights in an application at runtime, considering controlled highlight deliveries, trial and error, and rollback capacities.
27. What is "Nonstop Trying"?
- • Ceaseless Testing is the act of mechanizing testing all through the product improvement lifecycle, from advancement to sending, to guarantee that code changes meet quality and execution standards.
28. What are a few normal measurements utilized in DevOps?
- • Normal measurements utilized in DevOps incorporate lead time, arrangement recurrence, mean chance to recuperate (MTTR), mean time between disappointments (MTBF), and consumer loyalty measurements.
29. Make sense of the idea of "Shift-Right" in DevOps.
- • Shift-Right is the act of broadening testing and observing into creation conditions to accumulate constant input and experiences, empowering quicker recognition and goal of issues.
30. What is "Serverless Figuring"?
- • Serverless Registering is a distributed computing model where cloud suppliers progressively deal with the designation and provisioning of servers, permitting engineers to zero in on composing code without stressing over server the executives.
31. What is "Permanent Sending"?
- • Unchanging Sending is an organization procedure where application relics are sent as permanent, read-just items, wiping out the requirement for set up updates and decreasing the gamble of design float.
32. What is "Ceaseless Consistence"?
- • Nonstop Consistence is the act of persistently observing and upholding consistence necessities all through the product advancement lifecycle, guaranteeing that applications satisfy administrative and security guidelines.
33. What is "GitOps"?
- • GitOps is a functional model where foundation and application arrangements are overseen involving Git stores as the single wellspring of truth, taking into consideration forming, computerization, and joint effort.
34. What are "Administration Level Targets" (SLOs)?
- • Administration Level Goals are explicit, quantifiable focuses on that characterize the ideal degree of unwavering quality and execution for a help, normally communicated as a rate or scope of satisfactory qualities.
35. What is "Shift-Down" in DevOps?
- • Shift-Down is the act of improving and mechanizing functional undertakings to diminish manual mediation and let loose assets for higher-esteem exercises, like development and vital preparation.
36. Make sense of the idea of "Dull Sending off."
- • Dull Sending off is an organization procedure where new elements or changes are conveyed to creation yet stay stowed away from end-clients until they are fit to be initiated, taking into consideration slow rollout and testing.
37. What is "GitOps ChatOps"?
- • GitOps ChatOps is a mix of GitOps and ChatOps, where framework and application organizations are overseen through talk interfaces, empowering continuous joint effort and mechanization.
38. What is "Design Float," and how might it be alleviated?
- • Design Float happens when the genuine arrangement of sent assets separates from their planned setup after some time. It tends to be relieved through mechanization, rendition control, and normal setup reviews.
39. Make sense of the idea of "Shift-Up" in DevOps.
- • Shift-Up is the act of adjusting DevOps drives to more extensive business targets and key objectives, guaranteeing that innovation drives add to generally speaking business achievement.
40. What is "Serverless Design"?
- • Serverless Design is a building approach where applications are constructed utilizing serverless registering administrations, like AWS Lambda or Purplish blue Capabilities, disposing of the requirement for overseeing server foundation.
41. What is "Discernibleness," and for what reason is it significant in DevOps?
- • Recognizability is the capacity to comprehend the inner condition of a framework in view of its outer results. It is significant in DevOps for recognizing and diagnosing issues rapidly, empowering viable episode reaction and nonstop improvement.
42. What is "Security as Code"?
- • Security as Code is the act of coordinating security controls and designs into code stores and CI/Album pipelines, guaranteeing that safety efforts are applied reliably and naturally all through the product advancement lifecycle.
43. Make sense of the idea of "Shift-Down Security."
- • Shift-Down Security is the act of incorporating security controls and measures into functional cycles and work processes, empowering ceaseless checking, recognition, and reaction to security dangers.
44. What is "Occasion Driven Design," and how can it connect with DevOps?
- • Occasion Driven Design is a building design where application parts convey through the trading of occasions. It connects with DevOps by empowering decoupled, versatile, and tough frameworks that line up with DevOps standards.
45. What are a few critical contemplations for executing DevOps in heritage conditions?
- • Key contemplations for executing DevOps in heritage conditions incorporate social change, inheritance framework modernization, robotization of manual cycles, and gradual reception of DevOps rehearses.
46. Make sense of the idea of "Constant Criticism" in DevOps.
- • Consistent Input is the act of gathering, investigating, and following up on criticism from partners, clients, and observing frameworks all through the product advancement lifecycle, empowering ceaseless improvement and arrangement with client needs.
47. What is "Consistence as Code"?
- • Consistence as Code is the act of systematizing consistence prerequisites and controls into form controlled arrangements and mechanization scripts, guaranteeing that consistence measures are reliably applied and auditable.
48. What is "GitOps Work process"?
- • GitOps Work process is a product conveyance model where all foundation and application changes are overseen through Git vaults, with mechanization instruments observing these stores and applying changes to the objective conditions, guaranteeing forming, auditability, and cooperation.
49. What are "Kubernetes Administrators," and how would they improve DevOps rehearses?
- • Kubernetes Administrators are programming augmentations that robotize the administration of mind boggling, stateful applications on Kubernetes groups. They improve DevOps rehearses by empowering computerized lifecycle the board, scaling, and upkeep of uses running on Kubernetes.
50. How would you gauge the outcome of a DevOps execution?
- • The outcome of a DevOps execution can be estimated utilizing different measurements, including sending recurrence, lead time, mean chance to recuperate (MTTR), consumer loyalty, and business influence measurements like income development or cost investment funds.
51. What is the contrast between Persistent Joining and Nonstop Sending?
- • Persistent Mix (CI) includes habitually incorporating code changes into a common vault with mechanized forms and tests. Nonstop Arrangement (Album) naturally conveys code changes to creation in the wake of finishing mechanized assessments.
52. Make sense of the idea of "Foundation as Code" (IaC).
- • Foundation as Code is the act of overseeing and provisioning framework utilizing code, taking into account mechanized and steady framework arrangement.
53. What is a "Pipeline as Code," and for what reason is it significant in CI/Cd?
- • Pipeline as Code includes characterizing CI/Album pipelines utilizing code, which considers variant control, joint effort, and mechanization of the whole programming conveyance process.
54. How does Docker contrast from virtualization?
- • Docker utilizes containerization, what shares the host operating system piece, making it more lightweight than virtualization. Virtualization includes running different working frameworks on a solitary actual host.
55. What is the reason for a compartment coordination instrument like Kubernetes?
- • Kubernetes mechanizes the arrangement, scaling, and the board of containerized applications, giving elements like burden adjusting, self-mending, and moving updates.
56. Make sense of the job of a "Converse Intermediary" in a microservices design.
- • An opposite intermediary guides client solicitations to the fitting microservices, taking care of burden adjusting, security, and steering, consequently upgrading the versatility and security of the microservices engineering.
57. What is the meaning of the "12-factor application" strategy in DevOps?
- • The 12-factor application technique gives best practices to building versatile and viable applications in a cutting edge, cloud-local climate, lining up with DevOps standards.
58. What are the upsides of utilizing adaptation control frameworks like Git in DevOps?
- • Git empowers forming, cooperation, and discernibility of code changes, significant for CI/Album pipelines, and works with fanning and converging for equal improvement endeavors.
59. How does Blue-Green Arrangement vary from Canary Sending?
- • Blue-Green Sending keeps two indistinguishable creation conditions yet switches between them during organization. Canary Sending steadily carries out changes to a subset of clients or servers.
60. Make sense of the idea of "Moving Arrangement."
- • Moving Sending refreshes a framework continuously by steadily supplanting cases, guaranteeing the application stays accessible during the organization cycle.
61. What is the reason for a "Heap Balancer" in a DevOps climate?
- • A Heap Balancer circulates approaching organization traffic across various servers to guarantee ideal asset use, further develop versatility, and improve the accessibility of utilizations.
62. How does DevOps add to further developed programming quality?
- • DevOps advances consistent testing, cooperation, and mechanization, lessening manual mistakes, further developing code quality, and empowering quicker location and goal of issues.
63. What is "Git Stream," and how can it support code cooperation?
- • Git Stream is a spreading model that characterizes a bunch of stretching shows, making it simpler for groups to team up, oversee delivers, and keep a spotless variant history.
64. What is the job of "Curio Vaults" in CI/Cd pipelines?
- • Antique Vaults store and oversee fabricate ancient rarities, conditions, and different parallels, guaranteeing steady and dependable relic forms all through the CI/Album process.
65. What is the motivation behind "Consistent Checking" in DevOps?
- • Ceaseless Checking includes constant perception of framework measurements and logs to recognize issues, track execution, and work with proactive issue goal.
66. How does indeed "Element Flipping" support ceaseless organization?
- • Highlight Flipping considers the dynamic empowering or debilitating of elements underway, working with controlled include delivers and limiting the effect of issues.
67. What are the key contemplations while carrying out "Changeless Framework"?
- • Key contemplations incorporate regarding framework as dispensable, making new occurrences for refreshes, and limiting manual changes to running foundation parts.
68. What is "GitLab CI/Compact disc," and how can it smooth out improvement processes?
- • GitLab CI/Disc is an underlying CI/Cd arrangement in GitLab. It smoothes out improvement by giving a solitary stage to form control, CI/Cd pipelines, and task the executives.
69. How does indeed "Constant Incorporation" add to quicker programming conveyance?
- • Consistent Mix diminishes reconciliation issues by habitually consolidating code changes, empowering early identification of mistakes and guaranteeing a constantly deployable codebase.
70. Make sense of the idea of "Shift-Left Security" in DevOps.
- • Shift-Left Security includes coordinating security rehearses from the get-go in the product improvement lifecycle to distinguish and address weaknesses before they arrive at creation.
71. What is "Serverless Checking," and for what reason is it significant?
- • Serverless Observing includes following and dissecting the exhibition and conduct of serverless capabilities, enhancing asset use and distinguish expected issues.
72. Indeed how does "Kanban" support DevOps work processes?
- • Kanban is a visual administration apparatus that assists groups with picturing work, limit work underway, and enhance the progression of work, advancing persistent conveyance in a DevOps climate.
73. What is the motivation behind "Compartment Coordination" in microservices designs?
- • Holder Organization robotizes the sending, scaling, and the executives of containerized applications, guaranteeing effective asset use and high accessibility.
74. How does indeed "Reliance The executives" add to DevOps rehearses?
- • Reliance The executives includes following and overseeing programming conditions, guaranteeing steady and reproducible forms and diminishing the gamble of reliance related issues.
75. What is "Framework Robotization," and for what reason is it fundamental in DevOps?
- • Foundation Mechanization includes utilizing code to arrangement and oversee framework, guaranteeing consistency, repeatability, and versatility in a DevOps climate.
76. Make sense of the idea of "Dairy cattle, not Pets" in server the executives.
- • "Cows, not Pets" alludes to regarding servers as dispensable and tradable substances, limiting manual arrangement and stressing mechanization for overseeing framework.
77. What is the job of "Persistent Arrangement" in the DevOps lifecycle?
- • Nonstop Sending computerizes the delivery interaction, permitting code changes to be consequently conveyed to creation in the wake of breezing through mechanized assessments.
78. How does indeed "Adaptation to non-critical failure" add to framework unwavering quality in DevOps?
- • Adaptation to non-critical failure includes planning frameworks to endure and recuperate from disappointments, improving framework dependability and limiting personal time.
79. What is "Containerization," and how can it work on sending?
- • Containerization includes epitomizing applications and their conditions into compartments, giving consistency and transportability across various conditions, and working on organization.
80. How would you guarantee "High Accessibility" in a DevOps climate?
- • High Accessibility includes planning frameworks with excess parts and failover components, guaranteeing consistent activity and limiting assistance disturbances.
81. What is "Test-Driven Advancement" (TDD), and how can it line up with DevOps rehearses?
- • Test-Driven Advancement includes composing tests prior to composing code, advancing code quality, early deformity recognition, and supporting robotization in the testing system.
82. What is "Consistent Documentation," and for what reason is it significant in DevOps?
- • Nonstop Documentation includes keeping up with state-of-the-art documentation close by code changes, guaranteeing that documentation stays important and supporting information sharing.
83. Make sense of the idea of "Permanent Framework" and its advantages.
- • Permanent Framework includes making and conveying foundation parts in a manner that forestalls change after organization, improving consistency, unwavering quality, and security.
84. Indeed how does "Framework as Code" support DevOps standards?
- • Framework as Code empowers mechanized and reliable foundation provisioning, forming, and the board, lining up with DevOps standards of mechanization and repeatability.
85. What is the job of "Design The board" in a DevOps climate?
- • Design The executives includes computerizing the administration and organization of foundation setups, guaranteeing consistency and repeatability across conditions.
86. What is "Nonstop Organization," and how can it vary from Persistent Conveyance?
- • Nonstop Organization consequently sends each code change to creation in the wake of breezing through computerized assessments, though Consistent Conveyance guarantees that code changes are generally in a deployable state however requires manual endorsement for sending.
87. Make sense of the idea of "Foundation as Code" (IaC) and its advantages.
- • Framework as Code includes overseeing and provisioning foundation utilizing code, giving mechanization, consistency, and forming capacities, which line up with DevOps standards.
88. Indeed how does "Containerization" improve versatility in a DevOps climate?
- • Containerization epitomizes applications and their conditions into compartments, empowering lightweight, compact, and versatile organization of uses in a DevOps climate.
89. What is "GitOps," and how can it smooth out DevOps work processes?
- • GitOps is a functional model that involves Git stores as the wellspring of truth for foundation and application organizations, working with mechanization, forming, and joint effort in DevOps work processes.
90. How would you guarantee "Security as Code" in a DevOps climate?
- • Security as Code includes incorporating security rehearses into the product improvement lifecycle, guaranteeing that safety efforts are robotized, steady, and coordinated into CI/Disc pipelines.
91. What is the job of "Persistent Checking" in DevOps rehearses?
- • Persistent Checking includes ongoing perception of framework measurements and logs to recognize issues, track execution, and work with proactive issue goal in a DevOps climate.
92. Make sense of the idea of "Mayhem Designing" and its advantages in DevOps.
- • Confusion Designing includes purposely acquainting disappointments into a framework with test its flexibility and recognize shortcomings, at last further developing framework dependability and execution in DevOps.
93. What is "Shift-Left Testing," and how can it further develop programming quality in DevOps?
- • Shift-Left Testing includes moving testing exercises prior in the product improvement lifecycle, empowering early imperfection identification, diminishing revamp, and further developing by and large programming quality in DevOps.
94. How does indeed "Design Float" influence foundation the executives in a DevOps climate?
- • Setup Float happens when the genuine arrangement of sent assets veers from their expected design, prompting irregularities and likely issues in framework the executives in DevOps.
95. What is "Constant Reconciliation," and how can it uphold DevOps rehearses?
- • Persistent Joining includes habitually coordinating code changes into a common storehouse, computerizing constructs and tests, and guaranteeing a consistently deployable codebase, supporting DevOps practices of mechanization and cooperation.
96. Make sense of the idea of "Framework as Code" (IaC) and its advantages in DevOps.
- • Framework as Code includes overseeing and provisioning foundation utilizing code, giving mechanization, consistency, forming, and versatility helps that line up with DevOps standards.
97. What is "Kubernetes," and how can it work with compartment arrangement in DevOps?
- • Kubernetes is an open-source compartment organization stage that computerizes the sending, scaling, and the executives of containerized applications, working with proficient asset use and high accessibility in DevOps.
98. How does indeed "Blue-Green Sending" further develop organization unwavering quality in DevOps?
- • Blue-Green Organization includes keeping two indistinguishable creation conditions and exchanging between them during arrangement, diminishing free time and limiting the gamble of sending disappointments in DevOps.
99. What is "Constant Conveyance," and how can it contrast from Persistent Arrangement?
- • Nonstop Conveyance guarantees that code changes are generally in a deployable state yet requires manual endorsement for sending, though Ceaseless Organization consequently conveys each code change to creation subsequent to finishing robotized assessments.
100. Make sense of the job of "ChatOps" in DevOps rehearses.
- • ChatOps is a coordinated effort model where improvement and tasks groups use visit instruments to robotize work processes, share data, and execute undertakings progressively, further developing correspondence, effectiveness, and straightforwardness in DevOps rehearses.
Linux Admin Interview Questions And Answers
1. What is Linux?
- • Linux encompasses an open-source operating system kernel conceived by Linus Torvalds, derived from UNIX. It finds extensive utilization in servers, embedded systems, and desktop computers alike.
2. What characterizes a shell?
- • A shell serves as a command-line interpreter, facilitating user engagement with the operating system through the input of commands. Prominent examples encompass Bash, Zsh, and Korn shell (ksh).
3. What approach is employed to determine the size of a directory in Linux?
- • Employing the du command, accompanied by the -sh option, alongside the directory path, enables the presentation of a directory's size in a format easily comprehensible to humans.
4. Which command is instrumental in unveiling the manual pages in Linux?
- • The man command serves the purpose of revealing extensive manual pages in Linux. For instance, executing man ls reveals the manual page for the ls command.
5. Elaborate on the utility of the grep command.
- • Serving as a powerful tool, the grep command facilitates the exploration of specific patterns or textual content within files. It harmoniously integrates regular expressions and an assortment of options, thereby enabling customization of the search process.
6. What defines SSH?
- • SSH, an abbreviation for Secure Shell, stands as a cryptographic network protocol that guarantees secure remote access to systems and secure file transfers between them.
7. What role does the passwd command fulfill?
- • The passwd command stands as a tool employed to alter a user's password within the Linux environment.
8. Elucidate the purpose of the chmod command.
- • The chmod command serves as a mechanism for modifying the permissions attributed to files and directories within the Linux ecosystem.
9. What signifies a symbolic link in Linux?
- • A symbolic link, colloquially referred to as a symlink, embodies a distinctive file type, functioning as a pointer directing to another file or directory. It assumes the role of a shortcut or alias for its target counterpart.
10. What purpose does the df command serve?
- • The df command assumes the responsibility of presenting comprehensive information pertaining to disk space utilization within the file system.
11. How does one verify network connectivity in Linux?
- • By employing the ping command, followed by the domain name or IP address, one can effectively scrutinize network connectivity in the Linux environment.
12. Clarify the objectives of the top command.
- • The top command, when executed, imparts real-time insights into system processes, encompassing CPU usage, memory consumption, and additional system resources.
13. What characterizes a Linux distribution?
- • A Linux distribution, often referred to as a distro, embodies a customized version of the Linux operating system, incorporating the Linux kernel alongside supplementary software and tools tailored to cater to specific use cases.
14. What constitutes a daemon in Linux?
- • Within the Linux ecosystem, a daemon represents a background process that continuously operates, undertaking system-oriented tasks such as hardware management, network service administration, or system logging.
15. Specify the purpose behind the ps command.
- • The ps command functions as a valuable instrument, enabling the display of pertinent information concerning active processes within the system.
16. What is the function of a package manager in Linux?
- • A package manager is a crucial utility in Linux that facilitates the installation, updating, and management of software packages. This tool simplifies the process of acquiring and maintaining software on a Linux system, ensuring efficient software management. Prominent examples include apt for Debian-based systems and yum for Red Hat-based systems.
17. How can one obtain a comprehensive list of all files and directories within a particular directory in Linux?
- • The comprehensive listing of all files and directories within a specific directory can be achieved by utilizing the ls command devoid of any arguments. This command provides a detailed representation of the contents, allowing users to efficiently navigate and manage their directories.
18. What is the underlying purpose of the tar command?
- • The tar command serves as a versatile tool for creating, viewing, and manipulating tar archives within the Linux environment. Tar archives are instrumental in consolidating multiple files and directories into a single, easily manageable file. This command empowers users to efficiently bundle and extract various components, simplifying tasks such as backups and software distribution.
19. How is the kernel defined within the Linux operating system?
- • The kernel represents the fundamental core of the Linux operating system, responsible for managing crucial system resources such as memory, CPU utilization, and devices. Essentially, the kernel provides essential functionality for other software applications to operate seamlessly within the Linux ecosystem.
20. What is the primary purpose of the mv command in Linux?
- • In Linux, the mv command is primarily employed for the purpose of relocating files and directories from one location to another. This command facilitates efficient file management, enabling users to effortlessly organize and rearrange their system's file structure.
21. Explain the practical application of the grep command with a relevant example.
- • The grep command proves invaluable for conducting targeted searches within files, allowing users to identify specific patterns or text. For example, executing the command grep "pattern" file.txt enables users to swiftly search for the specified pattern within the file.txt file, streamlining the process of locating relevant information.
22. How is a process defined within the Linux system?
- • Within Linux, a process refers to the active instance of a running program. Each process possesses a unique process ID (PID) and consumes system resources such as CPU and memory. The Linux environment accommodates multiple concurrent processes, enabling efficient multitasking.
23. What is the procedure for terminating a process within the Linux system?
- • To terminate a process in Linux, one can employ the kill command followed by the process ID (PID). This procedure facilitates the swift termination of the targeted process. For instance, utilizing the command kill PID would effectively terminate the process associated with the specified PID.
24. What is the significance of the chmod command in Linux?
- • The chmod command holds significance within the Linux system as it enables users to modify and manipulate the permissions assigned to files and directories. By utilizing this command, users can exercise granular control over access permissions, enhancing the security and manageability of their system's files and directories.
25. Elucidate the practical application of the passwd command with a relevant example.
- • The passwd command plays a pivotal role in Linux as it allows users to change their respective passwords. Executing the command passwd username would facilitate the modification of the password for the specified user, offering users enhanced security and control over their system access.
26. How does the grep command contribute to the Linux system's functionality?
- • The grep command operates as a powerful tool within the Linux environment, serving to search for specific patterns or text within files. This functionality streamlines tasks such as data analysis and information retrieval, ultimately enhancing the efficiency and productivity of users.
27. What constitutes a shell script within the Linux ecosystem?
- • A shell script is essentially a text file comprising a series of commands that are executed by the shell interpreter within the Linux environment. These scripts provide users with an efficient method of automating tasks and executing complex commands, enhancing the system's usability and convenience.
28. Explain the practical use of the ls command in Linux, accompanied by a relevant example.
- • The ls command assumes a key role in Linux as it allows users to obtain a comprehensive listing of files and directories. For instance, executing the command ls -l would generate a detailed representation of files and directories within the system, providing users with valuable insights into their system's organization and structure.
29. What is the purpose of the ifconfig command within the Linux system?
- • The ifconfig command serves the crucial function of displaying essential information pertaining to network interfaces within the Linux environment. This includes details such as IP addresses and network configuration, enabling users to effectively manage and troubleshoot their network connections.
30. How can system information be accessed within the Linux environment?
- • To access system information in Linux, users can employ the uname command, supplemented with various options such as -a. By executing this command, users can retrieve critical system details like the kernel version and system architecture, facilitating efficient system administration.
31. What is the primary utility of the mv command within the Linux system?
- • The mv command plays a significant role within Linux as it enables users to seamlessly move files and directories from one location to another. By utilizing this command, users can effortlessly organize their system's file structure, enhancing overall file management capabilities.
32. Elucidate the practical application of the tar command within the Linux environment, accompanied by a relevant example.
- • The tar command assumes a valuable role in Linux as it enables users to create, view, and manipulate tar archives. For instance, executing the command tar -cvf archive.tar file1 file2 would successfully create a tar archive named archive.tar, comprising the specified files file1 and file2. This functionality streamlines tasks such as file bundling and archiving.
33. What is the definition of a symbolic link within the Linux system?
- • A symbolic link, also referred to as a symlink, represents a distinctive file type that serves as a pointer to another file or directory. By acting as a shortcut or alias, symbolic links facilitate efficient navigation and access to target files or directories, streamlining user workflows.
34. Explain the underlying purpose of the du command within the Linux system, supplemented by a relevant example.
- • The du command fulfills a vital role within Linux, allowing users to obtain comprehensive information regarding disk usage. For example, executing the command du -sh directory would display the size of the specified directory in a human-readable format, supplying users with valuable insights into their system's storage utilization.
35. What is the primary purpose of the df command within the Linux system?
- • The df command serves an essential role within Linux as it provides users with valuable information regarding disk space usage on the file system. By executing this command, users can easily assess and monitor their system's storage capacity, facilitating efficient resource management.
36. Elucidate the practical use of the ps command within the Linux environment, accompanied by a relevant example.
- • The ps command assumes considerable significance within Linux as it empowers users to retrieve pertinent information about active processes on the system. Executing the command ps -ef would generate a detailed display containing information about all processes, enhancing system monitoring and administration capabilities.
37. What is the underlying purpose of the uptime command within the Linux system?
- • The uptime command is instrumental in facilitating access to essential information regarding a system's uptime, load average, and current time within the Linux environment. This command provides users with valuable insights into their system's performance and availability, aiding in efficient system management.
38. Explain the practical application of the kill command within the Linux system, accompanied by a relevant example.
- • The kill command plays a crucial role within Linux as it enables users to terminate processes efficiently. For instance, executing the command kill PID would promptly terminate the process associated with the specified process ID (PID), streamlining the process of process management and system control.
39. How is a package manager defined within the Linux system?
- • A package manager represents an indispensable tool within Linux, facilitating the installation, updating, and management of software packages. This tool simplifies the process of acquiring and maintaining software on a Linux system, enhancing system functionality and user convenience.
40. Explain the underlying purpose of the head command within the Linux environment, supplemented by a relevant example.
- • The head command serves a crucial role within Linux as it enables users to view the initial lines of a file. For instance, executing the command head -n 10 file.txt would display the first 10 lines of the file.txt file, aiding in efficient data analysis and information retrieval.
41. What is the objective of utilizing the tail command in the Linux operating system?
- • The tail command serves the purpose of presenting the final few lines of a file in the Linux environment.
42. Elucidate the functionality of the find command in Linux, incorporating an illustrative instance.
- • The find command is employed for conducting searches for files and directories in Linux. For instance, executing find /path -name "*.txt" would search for files with the extension ".txt" within the designated directory.
43. What is the significance of the grep command in Linux?
- • The grep command holds significance in Linux as it facilitates the search for specific patterns or textual content within files.
44. Elaborate on the usage of the sed command in Linux, providing an example.
- • The sed command is utilized for the manipulation and transformation of textual data within the Linux platform. To exemplify, the command sed 's/search/replace/g' file.txt would replace all occurrences of the term "search" with "replace" in the file.txt.
45. What is the purpose of the awk command in Linux?
- • The awk command finds purpose in Linux for text processing and pattern matching. It is commonly employed for extracting and manipulating data from files.
46. Elucidate the functionality of the cut command in Linux, accompanied by an example.
- • The cut command is employed for extracting specific sections of text from files within the Linux environment. For instance, executing cut -d',' -f1 file.csv would extract the first column from a CSV file.
47. What is the objective of the sort command in Linux?
- • The sort command serves the purpose of arranging lines of text in a sorted manner within Linux. It can sort lines alphabetically, numerically, or according to other criteria.
48. Elaborate on the usage of the uniq command in Linux, providing an example.
- • The uniq command is used in Linux for filtering out adjacent duplicate lines from textual input. For instance, executing uniq file.txt would remove any duplicate lines present in the file.txt.
49. What is the significance of the wc command in Linux?
- • The wc command is used in Linux for calculating the number of lines, words, and characters contained within text files.
50. Elucidate the functionality of the tee command in Linux, accompanied by an example.
- • The tee command enables simultaneous reading from standard input, writing to standard output, and saving to files within the Linux operating system. For example, executing command | tee file.txt executes the specified command, writes its output to the file.txt, and also displays it on the terminal.
51. What is the function of the grep command in Linux?
- • The grep command serves the purpose of locating specific patterns or textual content within files in the Linux operating system.
52. Can you elaborate on the application of the sed command in Linux by providing an example?
- • In Linux, the sed command is utilized to perform text manipulation and transformations. For instance, sed 's/search/replace/g' file.txt replaces all instances of the "search" pattern with the "replace" pattern in the file.txt document.
53. What is the objective of the awk command in Linux?
- • The primary objective of the awk command in Linux is to facilitate text processing and pattern matching. It is frequently employed to extract and manipulate data from various files.
54. Can you explain the usage of the cut command in Linux with an example?
- • The cut command in Linux is employed to extract specific sections of text from files. For example, using the command cut -d',' -f1 file.csv will extract the first column from a CSV file based on the delimiter ",".
55. What is the purpose of the sort command in Linux?
- • The sort command in Linux is intended to arrange lines of text in a specific order. It can sort lines alphabetically, numerically, or based on other specified criteria.
56. Can you explain the application of the uniq command in Linux with an example?
- • The uniq command in Linux is used to eliminate duplicate lines from consecutive text input. For instance, executing uniq file.txt will remove duplicate lines from the file.txt document.
57. What is the function of the wc command in Linux?
- • The wc command in Linux is employed to calculate the number of lines, words, and characters in text files.
58. Can you explain the usage of the tee command in Linux with an example?
- • In Linux, the tee command is utilized to simultaneously read from standard input, write to standard output, and save the output to files. For example, executing command | tee file.txt will execute the command, write the output to file.txt, and display it on the terminal.
59. What is the definition of a shell script in Linux?
- • A shell script in Linux refers to a text file that contains a series of commands which are executed by the shell interpreter.
60. Can you explain the purpose of the crontab command in Linux?
- • The crontab command in Linux is used to schedule tasks or commands to run at specific times, dates, or intervals on a regular basis.
61. What is the function of the chmod command in Linux?
- • The chmod command in Linux is utilized to modify or change the permissions of files and directories.
62. Can you explain the application of the chown command in Linux with an example?
- • The chown command in Linux is employed to alter the ownership of files and directories. For example, executing chown user:group file.txt will modify the ownership of file.txt to the specified user and group.
63. What is meant by a symbolic link in Linux?
- • In Linux, a symbolic link, also known as a symlink, is a distinctive type of file that serves as a reference or shortcut to another file or directory.
64. Can you explain the purpose of the find command in Linux with an example?
- • The find command is used to search for files and directories. For example, executing find /path -name "*.txt" will search for files with the .txt extension in the specified directory.
65. What is the objective of the tar command in Linux?
- • The tar command in Linux is employed to create, view, and manipulate tar archives. It is commonly used for consolidating multiple files and directories into a single file.
66. Can you explain the usage of the df command in Linux with an example?
- • The df command in Linux is used to display information regarding disk space utilization on the file system.
67. What is the purpose of the du command in Linux?
- • The du command in Linux serves the purpose of presenting information about disk usage.
68. Can you explain the application of the mount command in Linux with an example?
- • The mount command in Linux is used to attach or mount filesystems and devices. For example, executing mount /dev/sdb1 /mnt will mount the sdb1 partition to the /mnt directory.
69. What is the function of the umount command in Linux?
- • The umount command in Linux is utilized to unmount or detach filesystems and devices.
70. Can you explain the usage of the lsof command in Linux with an example?
- • The lsof command in Linux is used to list open files and processes. For example, executing lsof /path/to/file will display the processes that have the specified file open.
71. What is the function of the ps command in the Linux operating system?
- • The ps command serves to provide detailed information about the active processes running on the Linux system.
72. Can you elucidate the purpose of the kill command in Linux along with an illustrative example?
- • The kill command is primarily employed to terminate processes within the Linux environment. For instance, by executing the command "kill PID," where PID represents the process ID, the specified process will be terminated.
73. What is the significance of the nice command in Linux?
- • The nice command enables the execution of commands with specified priorities in the Linux operating system.
74. Could you provide an explanation of the renice command in Linux, accompanied by an example?
- • The renice command is utilized to modify the priority of currently running processes in Linux. For example, executing the command "renice +10 -p PID" will increase the priority of the process with the assigned process ID (PID) by 10.
75. How would you define a Linux distribution?
- • A Linux distribution, also known as a distro, refers to a specific version of the Linux operating system that consists of the Linux kernel, in addition to customized software and tools tailored for specific use cases.
76. What is the purpose of the uname command in Linux, and could you provide an example?
- • The primary function of the uname command in Linux is to display system information such as the kernel version and system architecture. For instance, executing the command "uname -a" will provide a comprehensive overview of the system information.
77. What is the objective of the hostname command in Linux?
- • The hostname command is employed to either display or modify the hostname of the Linux system.
78. Can you explain the use of the ifconfig command in Linux, supported by an example?
- • The ifconfig command is utilized to display or configure network interfaces within the Linux environment.
79. What is the purpose of the ping command in Linux?
- • The ping command serves the purpose of testing the accessibility of a host on an IP network and measuring the round-trip time for packets sent to that particular host.
80. Explain the use of the traceroute command in Linux, providing an example.
- • The traceroute command is employed to trace the route that packets take from the local system to a destination host within the Linux operating system.
81. What is the significance of the netstat command in Linux?
- • The netstat command is used to display various network-related information, including network connections, routing tables, and interface statistics, within the Linux environment.
82. Can you elucidate the use of the route command in Linux, accompanied by an example?
- • The route command enables users to view or manipulate the IP routing table within the Linux operating system.
83. What is the purpose of the iptables command in Linux?
- • The iptables command is utilized to configure packet filtering, Network Address Translation (NAT), and perform other firewall-related tasks within the Linux environment.
84. Explain the use of the ssh command in Linux, supported by an example.
- • The ssh command is utilized to establish a secure, encrypted connection to a remote system within the Linux environment. For example, executing the command "ssh username@hostname" will connect to the remote system using the specified username.
85. What is the objective of the scp command in Linux?
- • The scp command serves the purpose of securely copying files between a local and a remote system within the Linux environment.
86. Can you explain the use of the rsync command in Linux, accompanied by an example?
- • The rsync command is employed to synchronize files and directories between a local and a remote system within the Linux operating system./li>
87. What is the purpose of the curl command in Linux?
- • The curl command is utilized to transfer data to or from a server using various protocols such as HTTP, HTTPS, FTP, and SCP within the Linux environment.
88. Explain the use of the wget command in Linux, providing an example.
- • The wget command is employed to download files from the internet using HTTP, HTTPS, or FTP protocols within the Linux operating system.
89. What is the significance of the tar command in Linux?
- • The tar command is used to create, view, and manipulate tar archives within the Linux environment. It is commonly employed for bundling multiple files and directories into a single file.
90. Can you elucidate the use of the zip command in Linux, supported by an example?
- • The zip command serves to create, view, and manipulate zip archives within the Linux operating system.
91. What is the purpose of the rsync command in Linux?
- • The rsync command is utilized to synchronize files and directories between a local and a remote system within the Linux environment.
92. Explain the use of the scp command in Linux, providing an example.
- • The scp command is employed to securely copy files between a local and a remote system within the Linux operating system.
93. What is the significance of the chmod command in Linux?
- • The chmod command is used to modify the permissions of files and directories within the Linux environment.
94. Can you elucidate the use of the chown command in Linux, accompanied by an example?
- • The chown command is employed to modify the ownership of files and directories within the Linux operating system.
95. What is the purpose of the find command in Linux?
- • The find command serves to search for files and directories within the Linux environment.
96. Explain the use of the df command in Linux, supported by an example.
- • The df command is utilized to display information regarding disk space usage on the file system within the Linux operating system.
97. What is the objective of the du command in Linux?
- • The du command is employed to display information about disk usage within the Linux environment.
98. Can you explain the use of the mount command in Linux, providing an example?
- • The mount command serves to mount filesystems and devices within the Linux operating system.
99. What is the purpose of the umount command in Linux?
- • The umount command is used to unmount filesystems and devices within the Linux environment.
100. Explain the use of the lsof command in Linux with an example.
- • The lsof command is used to list open files and processes in Linux.
AWS Interview Questions And Answers
1. What is AWS?
- • AWS (Amazon Web Administrations) is a distributed computing stage that offers a large number of administrations including processing power, capacity choices, systems administration, data sets, and the sky is the limit from there, given by Amazon.
2. What are the critical parts of AWS?
- • Key parts of AWS incorporate Versatile Register Cloud (EC2) for virtual servers, Basic Capacity Administration (S3) for object capacity, Social Data set Help (RDS) for oversaw data sets, and Virtual Confidential Cloud (VPC) for systems administration.
3. What is EC2?
- • EC2 (Versatile Figure Cloud) is a web administration given by AWS that permits clients to lease virtual servers, known as cases, on which they can run their own applications.
4. What is S3?
- • S3 (Basic Capacity Administration) is an article stockpiling administration given by AWS that permits clients to store and recover a lot of information as items, like documents or pictures, over the web.
5. What is IAM?
- • IAM (Character and Access The board) is a help given by AWS to overseeing client personalities and consents. It permits clients to safely control admittance to AWS assets.
6. What is a VPC?
- • VPC (Virtual Confidential Cloud) is a help given by AWS that permits clients to make a virtual organization in the cloud, which is separated from other virtual organizations and can be modified by unambiguous necessities.
7. What is RDS?
- • RDS (Social Data set Help) is an assistance given by AWS that permits clients to set up, work, and scale social data sets in the cloud without dealing with the basic foundation.
8. What is Lambda?
- • Lambda is a serverless processing administration given by AWS that permits clients to run code without provisioning or overseeing servers. It consequently scales and deals with the framework expected to run the code.
9. What is CloudFormation?
- • CloudFormation is a help given by AWS that permits clients to characterize and arrangement AWS foundation as code utilizing layouts. It empowers mechanized organization and the board of AWS assets.
10. What is Auto Scaling?
- • Auto Scaling is a component given by AWS that permits clients to naturally scale their EC2 examples in view of predefined conditions, for example, computer processor use or organization traffic.
11. What is CloudWatch?
- • CloudWatch is an observing and the board administration given by AWS that permits clients to gather and track measurements, screen log documents, set cautions, and consequently respond to changes in AWS assets.
12. What is Versatile Beanstalk?
- • Flexible Beanstalk is a help given by AWS that permits clients to convey and oversee applications in the cloud without stressing over the basic framework. It upholds different programming dialects and structures.
13. What is Highway 53?
- • Highway 53 is a versatile space name framework (DNS) web administration given by AWS that permits clients to course traffic to different AWS administrations or outside endpoints in light of determined rules.
14. What is CloudFront?
- • CloudFront is a substance conveyance organization (CDN) administration given by AWS that conveys content, for example, website pages, recordings, and pictures, to clients with low inactivity and high exchange speeds by storing content at edge areas all over the planet.
15. What is a S3 can?
- • A S3 can is a holder for putting away items, like records or pictures, in S3. Each item put away in a S3 pail is doled out an extraordinary key and can be gotten to over the web through an exceptional URL.
16. What is DynamoDB?
- • DynamoDB is a completely overseen NoSQL data set help given by AWS that offers quick and unsurprising execution with consistent versatility. Reasonable for applications require low-dormancy information access at any scale.
17. What is Ice sheet?
- • Icy mass is a minimal expense stockpiling administration given by AWS that is intended for information chronicling and long haul reinforcement. It offers secure and sturdy capacity with adaptable recovery choices.
18. What is an EC2 case?
- • An EC2 case is a virtual server given by AWS that can run different working frameworks and applications. Clients can browse an extensive variety of example types in light of their particular necessities for central processor, memory, stockpiling, and systems administration.
19. What is an AMI?
- • An AMI (Amazon Machine Picture) is a format used to make virtual servers, or EC2 occurrences, in AWS. It contains the working framework, applications, and design settings expected to send off an occasion.
20. What is an EBS volume?
- • An EBS (Flexible Block Store) volume is a block stockpiling gadget given by AWS that can be joined to EC2 examples to give tireless capacity. It permits clients to make, append, and disengage volumes to EC2 cases depending on the situation.
21. What is CloudTrail?
- • CloudTrail is a help given by AWS that records Programming interface calls and moves made by clients, administrations, and AWS assets in an AWS account. It gives perceivability into client movement and asset changes for security and consistence purposes.
22. What is the contrast among S3 and EBS?
- • S3 (Straightforward Capacity Administration) is an item stockpiling administration for putting away and recovering a lot of information over the web, while EBS (Flexible Block Store) is a block stockpiling administration for joining industrious capacity volumes to EC2 cases.
23. What is the contrast among RDS and DynamoDB?
- • RDS (Social Data set Help) is an overseen administration for running social information bases like MySQL, PostgreSQL, and SQL Server, while DynamoDB is a completely overseen NoSQL data set help.
24. What is the contrast among CloudWatch and CloudTrail?
- • CloudWatch is a checking and the board administration for gathering and following measurements and logs, while CloudTrail is a logging administration for recording Programming interface calls and moves made in AWS.
25. What is the contrast between an EC2 example and a Lambda capability?
- • An EC2 occasion is a virtual server that runs applications and administrations, while a Lambda capability is a serverless processing administration that runs code because of occasions without provisioning or overseeing servers.
26. What is the distinction between a public subnet and a private subnet in VPC?
- • A public subnet is a subnet in VPC with a course to the web passage, permitting assets inside the subnet to get to the web, while a private subnet is a subnet without a course to the web entryway, giving extra security to assets inside the subnet.
27. What is the contrast between an IAM client and an IAM job?
- • An IAM client is an element with extremely durable long haul qualifications used to cooperate with AWS administrations, while an IAM job is a substance with transitory certifications used to appoint admittance to AWS assets to clients or administrations.
28. What is the distinction between IAM arrangements and IAM jobs?
- • IAM approaches are reports that characterize authorizations for clients, gatherings, and jobs in AWS, while IAM jobs are elements with impermanent qualifications used to assign admittance to AWS assets.
29. What is the distinction between the AWS CLI and the AWS SDK?
- • The AWS CLI (Order Line Connection point) is an order line instrument used to collaborate with AWS administrations by means of the order line, while the AWS SDK (Programming Improvement Pack) is a bunch of libraries and devices for building applications that incorporate with AWS administrations.
30. What is the contrast between even scaling and vertical scaling?
- • Level scaling includes adding more examples or assets to a framework to deal with expanded load, while vertical scaling includes expanding the limit of existing occurrences or assets by adding more central processor, memory, or capacity.
31. What is Cross-Beginning Asset Sharing (CORS) in AWS?
- • CORS is a system that permits web applications running in a program to make solicitations to an unexpected space in comparison to the one from which the application was served. In AWS, CORS can be designed for S3 pails to control admittance to assets from various starting points.
32. What is an AWS Locale?
- • An AWS District is an actual area in the existence where AWS has server farms. Every District comprises of different Accessibility Zones and is totally autonomous of different Locales.
33. What is an AWS Accessibility Zone?
- • An AWS Accessibility Zone is at least one discrete server farms inside an AWS Locale that are detached from one another. They give high accessibility and adaptation to internal failure for AWS administrations.
34. What is AWS Lambda?
- • AWS Lambda is a serverless processing administration given by AWS that permits clients to run code without provisioning or overseeing servers. It consequently scales and deals with the framework expected to run the code.
35. What is AWS ECS?
- • AWS ECS (Versatile Compartment Administration) is a holder coordination administration given by AWS that permits clients to run, stop, and oversee Docker holders on a group of EC2 examples.
36. What is AWS Fargate?
- • AWS Fargate is a serverless figure motor for compartments given by AWS that permits clients to run Docker holders without provisioning or overseeing servers. It naturally scales and deals with the foundation expected to run the holders.
37. What is AWS EKS?
- • AWS EKS (Flexible Kubernetes Administration) is an overseen Kubernetes administration given by AWS that permits clients to send, make due, and scale Kubernetes bunches in the AWS cloud.
38. What is AWS SNS?
- • AWS SNS (Straightforward Warning Help) is a completely overseen informing administration given by AWS that permits clients to send notices to different endpoints, like email, SMS, HTTP, or Lambda capabilities.
39. What is AWS SQS?
- • AWS SQS (Basic Line Administration) is a completely overseen message lining administration given by AWS that permits clients to decouple and scale microservices, conveyed frameworks, and serverless applications.
40. What is AWS SES?
- • AWS SES (Straightforward Email Administration) is a versatile and savvy email sending and getting administration given by AWS that permits clients to send and get messages utilizing SMTP or the AWS SDKs.
41. What is AWS CloudFormation?
- • AWS CloudFormation is a help given by AWS that permits clients to characterize and arrangement AWS foundation as code utilizing formats. It empowers mechanized organization and the board of AWS assets.
42. What is AWS CloudWatch Logs?
- • AWS CloudWatch Logs is a help given by AWS that permits clients to screen, store, and access log records from AWS assets, for example, EC2 occurrences, Lambda capabilities, and CloudTrail.
43. What is AWS CloudTrail?
- • AWS CloudTrail is a logging administration given by AWS that records Programming interface calls and moves made by clients, administrations, and AWS assets in an AWS account. It gives perceivability into client movement and asset changes for security and consistence purposes.
44. What is AWS CodeDeploy?
- • AWS CodeDeploy is a sending administration given by AWS that mechanizes the most common way of conveying applications to EC2 examples, Lambda capabilities, and ECS bunches. It empowers fast and solid sending of uses.
45. What is AWS CodePipeline?
- • AWS CodePipeline is a ceaseless joining and constant conveyance (CI/Compact disc) administration given by AWS that mechanizes the most common way of building, testing, and sending code changes. It empowers quick and solid conveyance of updates to applications.
46. What is AWS CodeBuild?
- • AWS CodeBuild is a completely overseen construct administration given by AWS that gathers source code, runs tests, and creates programming bundles prepared for sending. It empowers constant incorporation and ceaseless conveyance (CI/Compact disc) work processes.
47. What is AWS CodeCommit?
- • AWS CodeCommit is a completely overseen source control administration given by AWS that has private Git stores. It empowers groups to safely store and oversee source code in the AWS cloud.
48. What is AWS CodeArtifact?
- • AWS CodeArtifact is a completely overseen relic storehouse administration given by AWS that has programming bundles and conditions. It empowers groups to safely store, distribute, and share programming antiquities in the AWS cloud.
49. What is AWS CodeDeploy?
- • AWS CodeDeploy is a sending administration given by AWS that robotizes the method involved with conveying applications to EC2 examples, Lambda capabilities, and ECS bunches. It empowers fast and dependable sending of uses.
50. What is AWS CodePipeline?
- • AWS CodePipeline is a nonstop reconciliation and constant conveyance (CI/Disc) administration given by AWS that mechanizes the most common way of building, testing, and sending code changes. It empowers fast and solid conveyance of updates to applications.
51. What is AWS Lambda?
- • AWS Lambda is a serverless figuring administration given by AWS that permits you to run code without provisioning or overseeing servers. It naturally scales and deals with the foundation expected to run your code.
52. What is AWS Versatile Beanstalk?
- • AWS Flexible Beanstalk is a help given by AWS that makes it simple to send, make due, and scale web applications and administrations. It naturally handles the organization, limit provisioning, load adjusting, and auto-scaling of your application.
53. What is AWS S3 Ice sheet?
- • AWS S3 Icy mass is a minimal expense stockpiling administration given by AWS that is intended for information chronicling and long haul reinforcement. It offers secure and strong capacity with adaptable recovery choices.
54. What is AWS IAM?
- • AWS IAM (Personality and Access The executives) is a help given by AWS to overseeing client characters and consents. It permits you to safely control admittance to AWS assets.
55. What is AWS CloudFormation?
- • AWS CloudFormation is a help given by AWS that permits you to characterize and arrangement AWS framework as code utilizing formats. It empowers mechanized organization and the executives of AWS assets.
56. What is AWS DynamoDB?
- • AWS DynamoDB is a fully managed NoSQL database service provided by AWS that provides predictable and rapid execution along with constant adaptability. Applications that demand low idleness information access at any scale should be reasonable.
57. What is AWS RDS?
- • AWS RDS (Social Data set Help) is a help given by AWS that permits you to set up, work, and scale social data sets in the cloud without dealing with the fundamental framework.
58. What is AWS Highway 53?
- • AWS Highway 53 is a versatile space name framework (DNS) web administration given by AWS that permits you to course traffic to different AWS administrations or outer endpoints in view of determined rules.
59. What is AWS CloudFront?
- • AWS CloudFront is a substance conveyance organization (CDN) administration given by AWS that conveys content, for example, site pages, recordings, and pictures, to clients with low inertness and high exchange speeds by reserving content at edge areas all over the planet.
60. What is AWS ECS?
- • AWS ECS (Versatile Holder Administration) is a compartment organization administration given by AWS that permits you to run, stop, and oversee Docker holders on a bunch of EC2 examples.
61. What is AWS EKS?
- • AWS EKS (Flexible Kubernetes Administration) is an overseen Kubernetes administration given by AWS that permits you to send, make due, and scale Kubernetes groups in the AWS cloud.
62. What is AWS SNS?
- • AWS SNS (Basic Notice Administration) is a completely overseen informing administration given by AWS that permits you to send warnings to various endpoints, like email, SMS, HTTP, or Lambda capabilities.
63. What is AWS SQS?
- • AWS SQS (Straightforward Line Administration) is a completely overseen message lining administration given by AWS that permits you to decouple and scale microservices, disseminated frameworks, and serverless applications.
64. What is AWS SES?
- • AWS SES (Basic Email Administration) is a versatile and financially savvy email sending and getting administration given by AWS that permits you to send and get messages utilizing SMTP or the AWS SDKs.
65. What is AWS CloudWatch?
- • AWS CloudWatch is an observing and the executives administration given by AWS that permits you to gather and track measurements, screen log records, set cautions, and consequently respond to changes in AWS assets.
66. What is AWS CloudTrail?
- • AWS CloudTrail is a logging administration given by AWS that records Programming interface calls and moves made by clients, administrations, and AWS assets in an AWS account. It gives perceivability into client action and asset changes for security and consistence purposes.
67. What is AWS CodeDeploy?
- • AWS CodeDeploy is an organization administration given by AWS that mechanizes the method involved with conveying applications to EC2 cases, Lambda capabilities, and ECS groups. It empowers quick and dependable arrangement of utilizations.
68. What is AWS CodePipeline?
- • AWS CodePipeline is a nonstop reconciliation and constant conveyance (CI/Cd) administration given by AWS that mechanizes the method involved with building, testing, and sending code changes. It empowers fast and solid conveyance of updates to applications.
69. What is AWS CodeBuild?
- • AWS CodeBuild is a completely overseen fabricate administration given by AWS that gathers source code, runs tests, and delivers programming bundles prepared for organization. It empowers consistent coordination and nonstop conveyance (CI/Disc) work processes.
70. What is AWS CodeCommit?
- • AWS CodeCommit is a completely overseen source control administration given by AWS that has private Git vaults. It empowers groups to safely store and oversee source code in the AWS cloud.
71. What is AWS CodeArtifact?
- • AWS CodeArtifact is a completely overseen relic storehouse administration given by AWS that has programming bundles and conditions. It empowers groups to safely store, distribute, and share programming curios in the AWS cloud.
72. What is AWS Cloud9?
- • AWS Cloud9 is a cloud-based coordinated improvement climate (IDE) given by AWS that permits you to compose, run, and investigate code with only an internet browser. It upholds different programming dialects and coordinated effort highlights.
73. What is AWS Paste?
- • AWS Paste is a completely overseen extricate, change, and burden (ETL) administration given by AWS that makes it simple to plan and load information for investigation. It consequently finds, lists, and changes information put away in different sources.
74. What is AWS Athena?
- • AWS Athena is an intuitive question administration given by AWS that permits you to break down information put away in S3 utilizing standard SQL questions. It empowers you to rapidly and effectively inquiry enormous datasets without overseeing foundation.
75. What is AWS EMR?
- • AWS EMR (Versatile MapReduce) is a completely overseen enormous information handling administration given by AWS that permits you to process and dissect a lot of information utilizing Apache Hadoop, Apache Flash, and other large information structures.
76. What is AWS Step Capabilities?
- • AWS Step Capabilities is a serverless organization administration given by AWS that permits you to facilitate the parts of conveyed applications utilizing visual work processes. It empowers you to construct and run multi-step work processes with blunder taking care of and state the executives.
77. What is AWS Bunch?
- • AWS Bunch is a completely overseen group handling administration given by AWS that permits you to run clump figuring jobs at any scale. It consequently arrangements the perfect proportion of figure assets and deals with the execution of cluster occupations.
78. What is AWS IoT?
- • AWS IoT (Web of Things) is an overseen cloud stage given by AWS that permits you to interface and oversee IoT gadgets at scale safely. It empowers you to gather, process, and dissect information from associated gadgets.
79. What is AWS X-Beam?
- • AWS X-Beam is a conveyed following help given by AWS that permits you to break down and investigate disseminated applications. It gives start to finish perceivability into demands as they travel through your application and across AWS administrations.
80. What is AWS AppSync?
- • AWS AppSync is a completely overseen GraphQL administration given by AWS that permits you to construct versatile and secure information APIs for portable and web applications. It consequently refreshes information continuously and gives disconnected abilities to versatile applications.
81. What is AWS Direct Interface?
- • AWS Direct Interface is an organization administration given by AWS that permits you to lay out a devoted organization association between your on-premises server farm and AWS. It furnishes a private and dependable association with predictable organization execution.
82. What is AWS VPN?
- • AWS VPN (Virtual Confidential Organization) is a help given by AWS that permits you to safely interface your on-premises organization to your AWS assets utilizing scrambled burrows over the web. It gives secure admittance to your AWS assets without presenting them to the public web.
83. What is AWS WAF?
- • AWS WAF (Web Application Firewall) is an overseen firewall administration given by AWS that permits you to shield your web applications from normal web exploits and assaults. It empowers you to make custom principles to impede or permit traffic in light of determined conditions.
84. What is AWS Safeguard?
- • AWS Safeguard is an overseen DDoS (Dispersed Disavowal of Administration) security administration given by AWS that shields your web applications from enormous and complex DDoS assaults. It gives generally on location and relief of DDoS assaults to keep your applications accessible.
85. What is AWS Firewall Administrator?
- • AWS Firewall Director is an overseen security administration given by AWS that permits you to halfway design and oversee firewall rules across your AWS records and assets. It empowers you to authorize security approaches and safeguard your applications from unapproved access.
86. What is AWS Insider facts Supervisor?
- • AWS Mysteries Chief is an overseen administration given by AWS that permits you to safely store, turn, and oversee delicate application insider facts, for example, Programming interface keys, passwords, and data set certifications. It empowers you to unify and mechanize the administration of your privileged insights.
87. What is AWS Frameworks Chief?
- • AWS Frameworks Supervisor is an overseen administration given by AWS that permits you to mechanize the administration of your foundation and applications at scale. It gives a bound together UI and a bunch of instruments for overseeing and observing your AWS assets.
88. What is AWS Config?
- • AWS Config is an overseen administration given by AWS that permits you to survey, review, and assess the setup of your AWS assets. It empowers you to follow changes to your assets and guarantee consistence with your association's arrangements.
89. What is AWS OpsWorks?
- • AWS OpsWorks is a setup the executives administration given by AWS that permits you to computerize the sending, design, and the board of your applications and foundation. It upholds Gourmet specialist and Manikin for characterizing and overseeing arrangements.
90. What is AWS CloudHSM?
- • AWS CloudHSM is an overseen equipment security module (HSM) administration given by AWS that permits you to create, store, and oversee cryptographic keys in a committed equipment security module. It gives secure and consistent key administration for your applications.
91. What is AWS KMS?
- • AWS KMS (Key Administration) is an overseen administration given by AWS that permits you to make and control encryption keys used to encode your information. It empowers you to coordinate encryption into your applications and safeguard your information very still and on the way.
92. What is AWS Testament Supervisor?
- • AWS Endorsement Chief is an overseen administration given by AWS that permits you to arrangement, make due, and convey SSL/TLS testaments for your AWS assets. It empowers you to protect your sites and applications with HTTPS.
93. What is AWS CloudWatch Logs Bits of knowledge?
- • AWS CloudWatch Logs Bits of knowledge is a help given by AWS that permits you to intuitively look and dissect log information continuously. It empowers you to acquire experiences into your applications and investigate issues rapidly.
94. What is AWS AppConfig?
- • AWS AppConfig is an overseen application setup administration given by AWS that permits you to make due, approve, and send application designs at scale. It empowers you to rapidly and securely carry out include banners, settings, and arrangements to your applications.
95. What is AWS Fargate?
- • AWS Fargate is a serverless register motor for compartments given by AWS that permits you to run Docker holders without provisioning or overseeing servers. It consequently scales and deals with the foundation expected to run your compartments.
96. What is AWS Lambda@Edge?
- • AWS Lambda@Edge is an expansion of AWS Lambda that permits you to run Lambda capabilities at AWS edge areas. It empowers you to alter and upgrade the substance conveyance and security of your web applications.
97. What is AWS Step Capabilities?
- • AWS Step Capabilities is a serverless organization administration given by AWS that permits you to facilitate the parts of conveyed applications utilizing visual work processes. It empowers you to assemble and run multi-step work processes with mistake taking care of and state the executives.
98. What is AWS X-Beam?
- • AWS X-Beam is a dispersed following help given by AWS that permits you to dissect and investigate disseminated applications. It gives start to finish perceivability into demands as they travel through your application and across AWS administrations.
99. What is AWS Enhance?
- • AWS Enhance is a bunch of devices and administrations given by AWS that permits you to construct versatile and secure web and portable applications. It gives a brought together work process to creating and sending applications with highlights like verification, examination, and disconnected information synchronization.
100. What is AWS Media Administrations?
- • AWS Media Administrations is a bunch of overseen administrations gave by AWS to handling, putting away, and conveying media content at scale. It incorporates administrations like AWS Natural MediaConvert, AWS Essential MediaLive, and AWS Basic MediaPackage for encoding, real time, and conveying video content.
Data Science with Python Interview Questions And Answers
1. What is Python and for what reason is it utilized in information science?
- • Python is an undeniable level programming language known for its straightforwardness and lucidness. It's broadly utilized in information science because of its broad libraries like NumPy, Pandas, and sci kit-realize, which give amazing assets to information control, examination, and AI.
2. What is NumPy and how could it be utilized in information science?
- • NumPy is a Python library for mathematical registering that offers help for huge, multi-faceted clusters and grids, alongside an assortment of numerical capabilities to proficiently work on these exhibits. It's fundamental in information science for dealing with mathematical information.
3. What are Pandas and how could they be utilized in information science?
- • Pandas is a Python library for information control and examination, especially for working with organized information like tables or CSV documents. It gives information structures like DataFrames and Series, and capabilities to deal with missing information, channel, bunch, and change information.
4. What is scikit-realize and how could it be utilized in information science?
- • sci-kit-learn is a Python library for AI based on top of NumPy, SciPy, and Matplotlib. It gives basic and effective apparatuses for information mining and information examination, including different calculations for characterization, relapse, bunching, and dimensionality decrease.
5. What is Matplotlib and how could it be utilized in information science?
- • Matplotlib is a Python library for making static, intelligent, and energized representations in information science. It gives a MATLAB-like connection point and coordinates well with NumPy and Pandas to plot information in different configurations like line plots, dissipate plots, histograms, and so forth.
6. What is the contrast between NumPy clusters and Python records?
- • NumPy clusters are more effective than Python records for mathematical tasks since they are homogeneous and fixed-size, taking into account vectorized activities. NumPy exhibits likewise give a more extensive scope of numerical capabilities upgraded for clusters.
7. What is a DataFrame in Pandas?
- • A Data Frame is a 2-layered named information structure in Pandas that addresses a plain information structure with lines and sections. It's like a bookkeeping sheet or SQL table and gives functionalities to information control and examination.
8. How would you peruse information from a CSV document into a data frame in Pandas?
- • You can utilize the pd.read_csv() capability in Pandas to peruse information from a CSV document into a data frame. For instance: df = pd.read_csv('file.csv').
9. What are the fundamental information types upheld by NumPy?
- • NumPy upholds different information types including numbers, drifting point numbers, complex numbers, booleans, strings, and date time objects. These information types are addressed by different information types like int32, float64, complex128, bool, str, datetime64, and so forth.
10. What is the contrast between a data frame and a Series in Pandas?
- • A data frame is a 2-layered information structure that addresses even information with lines and segments, while a Series is a 1-layered information structure that addresses a solitary section or column of information with a record.
11. How would you choose explicit segments from a data frame in Pandas?
- • You can utilize square sections [] or the loc[] and iloc[] accessors to choose explicit segments from a DataFrame. For instance: df['column'], df.loc[:, ' column'], df.iloc[:, column_index].
12. How would you channel lines in light of conditions in a DataFrame in Pandas?
- • You can utilize boolean ordering or the question() strategy to channel lines in light of conditions in a data frame. For instance: df[df['column'] > value], df.query('column > esteem').
13. What is the contrast between the loc[] and iloc[] accessors in Pandas?
- • The loc[] accessor is utilized to choose information given marks (line and section names), while the iloc[] accessor is utilized to choose information in light of the number of files (column and segment records).
14. What is the reason for the groupby() capability in Pandas?
- • The group () capability in Pandas is utilized to bunch information in a data frame in light of at least one segment and perform tasks like conglomeration, change, or sifting on each gathering.
15. How would you deal with missing information in a DataFrame in Pandas?
- • You can utilize strategies like isnull(), dropna(), fillna(), or add() to deal with missing information in a data frame in Pandas. These techniques permit you to recognize, eliminate, supplant, or add missing qualities to your information.
16. What is the motivation behind the apply() capability in Pandas?
- • The apply() capability in Pandas is utilized to apply a capability along a pivot of a data frame or Series. It permits you to apply custom or underlying capabilities to each line or segment of the data frame.
17. How would you link DataFrames in Pandas?
- • You can utilize the concat() capability in Pandas to link DataFrames in an upward direction (along columns) or on a level plane (along segments). For instance: pd.concat([df1, df2], axis=0) for vertical link and pd.concat([df1, df2], axis=1) for flat connection.
18. What is the reason for the union() capability in Pandas?
- • The union() capability in Pandas is utilized to combine at least two DataFrames in light of a typical section or record. It performs information base style joins like inward, external, left, and right joins to consolidate information from different DataFrames.
19. What is the motivation behind the pivot_table() capability in Pandas?
- • The pivot_table() capability in Pandas is utilized to make a bookkeeping sheet-style turn table from a data frame. It permits you to sum up and total information in a data frame by turning on at least one segment.
20. What is the reason for the value_counts() capability in Pandas?
- • The value_counts() capability in Pandas is utilized to count the events of special qualities in a Series. It returns a Series containing the counts of exceptional qualities, arranged in plummeting requests naturally.
21. What is the reason for the corr() capability in Pandas?
- • The corr() capability in Pandas is utilized to process the connection between sections in a data frame. It returns a relationship network showing the connection coefficients between all sets of segments.
22. What is the motivation behind the portray() capability in Pandas?
- • The depict() capability in Pandas is utilized to create graphic measurements for numeric and object (all-out) segments in a data frame. It gives outline measurements like count, mean, standard deviation, least, greatest, and percentiles.
23. What is the reason for the plot() capability in Pandas?
- • The plot() capability in Pandas is utilized to make different kinds of plots and representations from information in a data frame. It gives a helpful connection point to Matplotlib for producing line plots, bar plots, disperse plots, histograms, and so on.
24. What is the motivation behind the seaborn library in Python?
- • Seaborn is a Python representation library given Matplotlib that gives an undeniable level connection point to drawing appealing and useful factual illustrations. It works on the most common way of making complex representations like heat maps, violin plots, match plots, and so forth.
25. What is the reason for the train_test_split() capability in scikit-learn?
- • The train_test_split() capability in sci-kit-learn is utilized to part a dataset into preparing and testing sets for model assessment. It haphazardly divides the information into two subsets given a predetermined test size or train size.
26. What is cross-approval, and for what reason is it utilized in AI?
- • Cross-approval is a strategy utilized in AI to assess the exhibition of a model by dividing the information into numerous subsets (creases) and preparing the model on every subset while assessing it on the excess subsets. It gives a more vigorous gauge of the model's presentation by diminishing the effect of examining fluctuation.
27. What is regularisation, and for what reason is it utilised in AI?
- • Regularization is a strategy utilized in AI to forestall overfitting by adding a punishment term to the misfortune capability that punishes huge coefficients or model intricacy. It assists with summing up the model and working on its presentation of inconspicuous information.
28. What are hyperparameters, and how would you tune them in AI?
- • Hyperparameters are boundaries that are set before the preparation interaction and control the growing experience of an AI calculation. Models incorporate the learning rate, regularisation strength, and number of stowed-away units in a brain organization. Hyperparameters are tuned using methods like framework search, arbitrary inquiry, or Bayesian advancement to find the ideal qualities that amplify the model's presentation.
29. What is highlight scaling, and for what reason is it significant in AI?
- • Highlight scaling is a procedure utilized in AI to normalize or standardize the scope of free factors or elements in the dataset. It's significant because it guarantees that all elements contribute similarly to the model's presentation and keeps highlights with bigger scopes from overwhelming the educational experience.
30. What is one-hot encoding, and for what reason is it utilised in AI?
- • One-hot encoding is a procedure used to address straight-out factors as twofold vectors where every class is addressed by a parallel worth (0 or 1). It's utilised in AI to deal with absolute factors in models that require mathematical information, like brain organisation and direct relapse.
31. What is feature engineering and why is it important in machine learning?31. What is highlight design, and for what reason is it significant in AI?
- • Highlight design is the most common way of making new elements or changing existing highlights in the dataset to work on the exhibition of AI models. It's significant because the nature of the elements can fundamentally affect the model's prescient power and speculation capacity.
32. What is cross-entropy misfortune, and for what reason is it utilised in orderly undertakings?
- • Cross-entropy misfortune, otherwise called log misfortune, is a misfortune capability utilized in characterization undertakings to gauge the distinction between the anticipated probabilities of the model and the real marks. It's ordinarily utilized in calculated relapse and brain networks for multi-class grouping issues..
33. What is the reason for the GridSearchCV() capability in scikit-learn?
- • The GridSearchCV() capability in sci-kit-learn is utilised to perform lattice search cross-approval to tune the hyperparameters of an AI model. It thoroughly looks through a predefined framework of hyperparameters and assesses the model presentation utilising cross-approval.
34. What is the motivation behind the RandomForestClassifier() capability in scikit-learn?
- • The RandomForestClassifier() capability in sci-kit-learn is utilized to make an irregular backwoods classifier, which is a group learning strategy that forms various choice trees and joins their forecasts to work on the precision and power of the model.
35. What is the reason for the GradientBoostingClassifier() capability in scikit-learn?
- • The GradientBoostingClassifier() capability in sci-kit-learn is utilized to make a slope-supporting classifier, which is a group learning technique that forms a succession of choice trees where each tree rectifies the blunders of the past tree to work on the model's prescient power.
36. What is the reason for the KNeighborsClassifier() capability in scikit-learn?
- • The KNeighborsClassifier() capability in sci-kit-learn is utilized to make a k-closest neighbors classifier, which is a non-parametric technique utilized for order errands. It relegates a class name to an info test given the larger part class mark of its k closest neighbors in the component space.
37. What is the motivation behind the DecisionTreeClassifier() capability in scikit-learn?
- • The DecisionTreeClassifier() capability in sci-kit-learn is utilized to make a choice tree classifier, which is a managed learning calculation that pursues expectations by gaining straightforward choice standards gathered from the preparation information.
38. What is the motivation behind the LogisticRegression() capability in scikit-learn?
- • The LogisticRegression() capability in sci-kit-learn is utilised to make a calculated relapse classifier, which is a direct model for double-characterization errands. It gauges the likelihood that an information test has a place with a specific class utilising a calculated (sigmoid) capability.
39. What is the reason for the StandardScaler() capability in scikit-learn?
- • The StandardScaler() capability in sci-kit-learn is utilized to normalize highlights by eliminating the mean and scaling to unit fluctuation. It changes the information to have a mean of 0 and a standard deviation of 1, which is significant for calculations that depend on highlight scaling, like help vector machines and k-closest neighbours.
40. What is the reason for the MinMaxScaler() capability in scikit-learn?
- • The MinMaxScaler() capability in sci-kit-learn is utilized to standardize highlights by scaling each component to a predefined range, normally somewhere in the range of 0 and 1. It protects the state of the first dissemination and is less impacted by exceptions compared with normalisation.
41. What is the reason for the PCA() capability in scikit-learn?
- • The PCA() capability in sci-kit-learn is utilized to perform head part examination (PCA), which is a dimensionality decrease procedure that changes high-layered information into a lower-layered space while protecting a large portion of the fluctuation in the information. It's valuable for representation, sound reduction, and accelerating learning calculations.
42. What is the reason for the TSNE() capability in sci-kit-learn?
- • The TSNE() capability in sci-kit-learn is utilized to perform t-conveyed stochastic neighbor implanting (t-SNE), which is a dimensionality decrease procedure that envisions high-layered information in a lower-layered space while saving the nearby design of the information. It's normally utilized for imagining high-layered datasets in a few aspects.
43. What is the reason for the train_test_split() capability in scikit-learn?
- • The train_test_split() capability in sci-kit-learn is utilised to partition a dataset into preparation and testing sets for model assessment. It haphazardly divides the information into two subsets, given a predetermined test size or train size.
44. What is the reason for the cross_val_score() capability in scikit-learn?
- • The cross_val_score() capability in sci-kit-learn is utilized to perform cross-approval and assess the exhibition of a model by dividing the information into numerous subsets (folds), preparing the model on each overlap, and assessing it on the leftover folds. It returns a variety of scores for each overlap, which can be utilized to appraise the model's presentation.
45. What is the reason for the GridSearchCV() capability in scikit-learn?
- • The GridSearchCV() capability in sci-kit-learn is utilised to perform lattice search cross-approval to tune the hyperparameters of an AI model. It thoroughly looks through a predefined framework of hyperparameters and assesses the model presentation utilising cross-approval.
46. What is the motivation behind the RandomizedSearchCV() capability in scikit-learn?
- • The RandomizedSearchCV() capability in sci-kit-learn is utilised to perform randomised search cross-approval to tune the hyperparameters of an AI model. It arbitrarily tests hyperparameters from determined conveyances and assesses the model presentation using cross-approval.
47. What is the motivation behind the make_classification() capability in scikit-learn?
- • The make_classification() capability in sci-kit-learn is utilized to produce a manufactured dataset for parallel or multi-class grouping undertakings with determined highlights, classes, and class conveyance. It's valuable for testing and prototyping AI calculations.
48. What is the reason for the make_regression() capability in scikit-learn?
- • The make_regression() capability in sci-kit-learn is utilized to create a manufactured dataset for relapse undertakings with determined highlights, coefficients, and commotion levels. It's helpful for testing and prototyping relapse calculations.
49. What is the motivation behind the make_blobs() capability in scikit-learn?
- • The make_blobs() capability in sci-kit-learn is utilized to create an engineered dataset with bunches of focuses for grouping errands with determined focuses, bunch standard deviation, and number of tests per bunch. It's valuable for testing and prototyping bunching calculations.
50. What is the motivation behind the make_moons() capability in scikit-learn?
- • The make_moons() capability in sci-kit-learn is utilized to produce an engineered dataset for paired order errands with two interleaving half circles. It's valuable for testing and prototyping non-straight grouping calculations.
51. What is the reason for the pickle module in Python?
- • The pickle module in Python is utilized for serializing and deserializing Python objects. It permits objects to be changed over into a byte stream, which can be saved to a document or communicated over an organization, and later reproduced into the first items.
52. Make sense of the contrast among == and is administrators in Python.
- • The == administrator is utilized to check assuming the upsides of two articles are equivalent, while the is administrator is utilized to check in the event that two items allude to a similar memory area (i.e., they are a similar item).
53. What is the reason for the __init__ strategy in Python classes?
- • The __init__ strategy is a unique technique in Python classes that is called when another occurrence of the class is made. It is utilized to introduce the case with default values or contentions passed during launch.
54. What is the motivation behind the __str__ technique in Python classes?
- • The __str__ technique is an exceptional strategy in Python classes that is called when the str() capability is utilized to switch an item over completely to a string. It ought to return a comprehensible string portrayal of the article.
55. What is a lambda capability in Python?
- • A lambda capability in Python is an unknown capability characterized utilizing the lambda catchphrase. It can take quite a few contentions however can have one articulation. Lambda capabilities are ordinarily utilized for short, basic tasks.
56. What is list perception in Python?
- • List cognizance in Python is a brief method for making records by applying an articulation to every component of an iterable and sifting components in light of a condition. It gives a more reduced and clear sentence structure contrasted with customary for circles.
57. What is the reason for the zip() capability in Python?
- • The zip() capability in Python is utilized to consolidate numerous iterables (records, tuples, and so on.) into a solitary iterable of tuples where each tuple contains components from comparing positions in the information iterables.
58. What is the motivation behind the guide() capability in Python?
- • The guide() capability in Python is utilized to apply a capability to every component of an iterable (list, tuple, and so on.) what's more, return an iterator that yields the outcomes. It gives a more brief and proficient option in contrast to utilizing a for circle with a capability call.
59. What is the reason for the channel() capability in Python?
- • The channel() capability in Python is utilized to apply a capability to every component of an iterable and return an iterator that yields just the components for which the capability brings Valid back. It gives a helpful method for sifting components in view of a condition.
60. What is the motivation behind the decrease() capability in Python?
- • The lessen() capability in Python is utilized to apply a capability to the components of an iterable and decrease them to a solitary worth. It more than once applies the capability to sets of components until the iterable is depleted, creating a solitary outcome.
61. What is the motivation behind the count() capability in Python?
- • The specify() capability in Python is utilized to repeat over an iterable (list, tuple, and so forth.) what's more, return an iterator of tuples where each tuple contains a count (beginning from 0 naturally) and the relating esteem from the iterable.
62. What is the reason for the arranged() capability in Python?
- • The arranged() capability in Python is utilized to sort the components of an iterable (list, tuple, and so forth.) in climbing request and return another rundown with the arranged components. It doesn't adjust the first iterable.
63. What is the motivation behind the any() capability in Python?
- • The any() capability in Python is utilized to check if any component of an iterable (list, tuple, and so forth.) assesses to Valid. It returns Valid assuming somewhere around one component is Valid, in any case it gets back Misleading.
64. What is the motivation behind the all() capability in Python?
- • The all() capability in Python is utilized to check if all components of an iterable (list, tuple, and so forth.) assess to Valid. It returns Valid assuming all components are Valid, in any case it gets back Bogus.
65. What is the motivation behind the isinstance() capability in Python?
- • The isinstance() capability in Python is utilized to check in the event that an item is an occasion of a predefined class or a subclass of the predetermined class. It returns Valid in the event that the article is a case of the class, in any case it gets back Misleading.
66. What is the motivation behind the issubclass() capability in Python?
- • The issubclass() capability in Python is utilized to check in the event that a class is a subclass of another class. It returns Valid on the off chance that the class is a subclass, in any case it gets back Bogus.
67. What is the reason for the super() capability in Python?
- • The super() capability in Python is utilized to call strategies for the superclass from a subclass. It returns an intermediary object that delegates strategy calls to the superclass, permitting you to get to techniques and properties of the superclass.
68. What is strategy goal request (MRO) in Python?
- • Technique goal request (MRO) in Python is the request where classes are looked for strategy and characteristic goal in legacy ordered progressions. It follows the C3 linearization calculation to decide the request where base classes are looked.
69. What is numerous legacy in Python?
- • Different legacy in Python is the capacity for a class to acquire qualities and strategies from various base classes. It permits a subclass to acquire from more than one superclass, empowering code reuse and advancing particular plan.
70. What is administrator over-burdening in Python?
- • Administrator over-burdening in Python is the capacity to characterize custom way of behaving for worked in administrators (+, - , *,/, and so on.) when applied to objects of a client characterized class. It permits objects to answer administrators in a characteristic and natural manner.
71. What is technique superseding in Python?
- • Technique superseding in Python is the capacity for a subclass to give a particular execution of a strategy that is now characterized in its superclass. It permits a subclass to tweak or broaden the way of behaving of a strategy acquired from its superclass.
72. What is technique over-burdening in Python?
- • Technique over-burdening in Python is the capacity to characterize various strategies with a similar name yet various marks (number or kinds of boundaries) in a class. Dissimilar to some other programming dialects, Python doesn't uphold strategy over-burdening of course.
73. What is a generator in Python?
- • A generator in Python is a unique kind of iterator that produces esteems lethargically on request as opposed to putting away them in memory at the same time. It permits you to repeat over a grouping of values without unequivocally making and putting away the whole succession in memory.
74. What is a decorator in Python?
- • A decorator in Python is a capability that wraps another capability or strategy and broadens its way of behaving without changing its source code. It permits you to add usefulness to existing capabilities or techniques progressively at runtime.
75. What is a setting director in Python?
- • A setting director in Python is an item that executes the setting the executives convention (utilizing __enter__() and __exit__() techniques) to oversee assets (e.g., records, network associations) inside a specific situation (e.g., with proclamation). It permits you to guarantee that assets are appropriately overseen and tidied up.
76. What is the reason for the proclamation in Python?
- • The explanation in Python is utilized to make a setting chief and oversee assets inside a unique situation. It guarantees that assets are appropriately overseen and tidied up regardless of whether a special case happens inside the specific situation.
77. What is the motivation behind the async and anticipate watchwords in Python?
- • The async and anticipate catchphrases in Python are utilized to characterize offbeat capabilities and coroutines, separately. They are utilized in nonconcurrent programming to perform non-hindering I/O activities and simultaneous execution of errands.
78. What is the reason for the asyncio module in Python?
- • The asyncio module in Python is a library for nonconcurrent programming and occasion circle-based simultaneousness. It gives an occasion circle, coroutines, undertakings, and utilities for composing nonconcurrent I/O-bound and significant-level organized network code.
79. What is the reason for the concurrent? futures module in Python?
- • The concurrent. futures module in Python is a significant level connection point for non-concurrently executing callables (capabilities or techniques) with a string pool or a cycle pool. It gives the ThreadPoolExecutor and ProcessPoolExecutor classes for simultaneous execution of assignments.
80. What is the reason for the stringing module in Python?
- • The stringing module in Python is a low-level connection point for working with strings. It gives classes and works to making, controlling, and synchronizing strings for simultaneous execution of undertakings.
81. What is the Worldwide Translator Lock (GIL) in Python?
- • The Worldwide Translator Lock (GIL) in Python is a mutex that safeguards admittance to Python objects, keeping different local strings from executing Python bytecodes at the same time in multi-strung Python programs. It is a component utilized in the CPython translator to guarantee string wellbeing however can restrict execution in multi-strung central processor-bound programs.
82. What is the reason for the logging module in Python?
- • The logging module in Python is a standard library module for logging symptomatic and enlightening messages from Python programs. It gives classes and works to making and arranging lumberjacks, overseers, formatters, and channels to control the result and organization of log messages.
83. What is the reason for the unit test module in Python?
- • The unit test module in Python is a standard library module for unit testing Python code. It gives classes and techniques to characterize experiments, test suites, and test sprinters to computerize the testing of capabilities, strategies, and classes.
84. What is the motivation behind the doc test module in Python?
- • The doc test module in Python is a standard library module for testing Python code by running models implanted in docstrings and contrasting the result with the normal outcomes. It gives a basic and lightweight method for composing and running tests straightforwardly inside the documentation of Python modules and capabilities.
85. What is the reason for the PDB module in Python?
- • The PDB module in Python is a standard library module for intelligent troubleshooting of Python programs. It gives a debugger that permits you to examine the execution of a program, set breakpoints, step through code, and assess articulations intelligently.
86. What is the motivation behind the traceback module in Python?
- • The traceback module in Python is a standard library module for catching and designing traceback data from exemptions and stack follows. It gives capabilities to separate and organize traceback data to help with troubleshooting and mistake announcing.
87. What is the motivation behind the timeit module in Python?
- • The timeit module in Python is a standard library module for estimating the execution season of little code bits or capabilities. It gives a helpful point of interaction to running timing examinations and looking at the exhibition of various executions.
88. What is the reason for the sys module in Python?
- • The sys module in Python is a standard library module that gives admittance to framework explicit boundaries and capabilities. It permits you to collaborate with the Python mediator, access order line contentions, control the runtime climate, and interface with the working framework.
89. What is the motivation behind the os module in Python?
- • The os module in Python is a standard library module that furnishes capabilities for connecting with the working framework. It permits you to perform tasks like document and registry control, process the executives, and climate variable control, and that's just the beginning.
90. What is the motivation behind the OS? path module in Python?
- • The os. path module in Python is a standard library module that gives capabilities to controlling record ways and performing way-related tasks in a stage-free way. It permits you to build, standardize, split, join, and control record ways without stressing over stage explicit contrasts.
91. What is the motivation behind the shutil module in Python?
- • The shutil module in Python is a standard library module that gives undeniable level record tasks and utilities for duplicating, moving, chronicling, and erasing documents and registries. It gives capabilities for working document framework ways, recording articles, and cataloging trees.
92. What is the reason for the glob module in Python?
- • The glob module in Python is a standard library module that gives capabilities to look for documents and catalogs utilizing special case designs like those utilized in shell orders. It permits you to find and count records and catalogs that match determined designs.
93. What is the reason for the argparse module in Python?
- • The argparse module in Python is a standard library module for parsing order line contentions and choices. It gives a helpful and adaptable method for characterizing and parsing order line interfaces for Python contents and applications.
94. What is the motivation behind the JSON module in Python?
- • The JSON module in Python is a standard library module that gives capabilities to encoding and unraveling JSON (JavaScript Item Documentation) information. It permits you to serialize Python objects to JSON strings and deserialize JSON strings to Python objects.
95. What is the motivation behind the CSV module in Python?
- • The CSV module in Python is a standard library module that gives capabilities to perusing and composing CSV (Comma-Isolated Values) records. It permits you to peruse information from CSV records into Python information structures like records or word references and compose information from Python information designs to CSV documents.
96. What is the reason for the pickle module in Python?
- • The pickle module in Python is a standard library module for serializing and deserializing Python objects. It permits objects to be changed over into a byte stream, which can be saved to a document or communicated over an organization, and later reproduced into the first items.
97. What is the motivation behind the solicitations module in Python?
- • The solicitations module in Python is an outsider library for making HTTP demands and cooperating with web APIs. It gives an undeniable level point of interaction to sending HTTP demands, taking care of reactions, and overseeing meetings, treats, and headers.
98. What is the motivation behind the beautifulsoup4 module in Python?
- • The beautifulsoup4 module in Python is an outsider library for parsing HTML and XML records. It gives a helpful connection point to exploring and controlling the parse tree of HTML and XML records, separating information, and scratching pages.
99. What is the motivation behind the scrappy system in Python?
- • The scrappy structure in Python is an outsider web slithering and web scratching system for separating information from sites. It gives a strong and extensible design for composing web bugs to creep and scratch pages, separately organize information, and store it in different configurations.
100. What is the motivation behind the selenium library in Python?
- • The selenium library in Python is an outsider library for robotizing internet browsers. It gives a WebDriver Programming interface to control internet browsers like Chrome, Firefox, and Safari automatically, permitting you to connect with pages, finish up structures, click fastens, and scratch dynamic substance.
Data Analyst Interview Questions And Answers
1. What is an information investigation, and for what reason is it significant?
- • Information examination is the method involved with assessing, cleaning, changing, and displaying information to find valuable data, draw inferences, and navigate back. It's significant because it assists organisations and associations with pursuing informed choices, recognising drifts, and further developing processes.
2. What are the various sorts of information investigations?
- • unmistakable examination (summing up and imagining information), exploratory investigation (tracking down examples and connections in information), prescient examination (making expectations in light of verifiable information), and prescriptive investigation (giving suggestions for future activities).
3. What are a few normal information cleaning methods?
- • Eliminating copies, taking care of missing qualities (attribution, cancellation, or assessment), adjusting information mistakes, normalising information arrangements, and managing anomalies.
4. Make sense of the contrast between connection and causation.
- • Connection alludes to a measurable connection between two factors where changes in a single variable are related to changes in another variable. Causation suggests that adjustments to one variable straightforwardly cause changes in another variable.
5. What is the hypothesis as far as possible, and for what reason is it significant in measurements?
- • As far as possible The hypothesis expresses that the dissemination of the test implies moving towards an ordinary conveyance as the example size builds, no matter what the state of the population dispersion. It's significant because it takes into consideration the utilisation of inferential measurements, for example.
6. What is theory trying, and how can it work?
- • Theory testing is a measurable strategy used to make assumptions about population boundaries given test information. It includes figuring out an invalid speculation (no impact) and an elective theory, choosing an importance level, gathering information, and utilising factual tests to decide if to dismiss the invalid speculation.
7. What is A/B testing, and how could it be utilized in information examination?
- • A/B testing is a controlled trial where at least two variations of a site page, email, or other computerized resource are contrasted to figure out which one performs better concerning a predefined metric (e.g., change rate, active clicking factor).
8. What is the distinction between a population and an example in measurements?
- • A populace is the whole gathering of people or things that the specialist is keen on considering, while an example is a subset of the populace chosen for study.
9. What is information perception, and for what reason is it significant in information examination?
- • Information perception is the graphical portrayal of information to convey data plainly and really. It's significant because it assists experts and partners with understanding complex information examples, patterns, and connections initially.
10. What are a few normal information representation devices and procedures?
- • Tools: Scene, Power BI, Matplotlib, Seaborn, and ggplot2. Techniques: bar diagrams, line graphs, disperse plots, histograms, box plots, heat maps, and intelligent dashboards.
11. What is the contrast between organised and unstructured information?
- • Organized information is coordinated in a predefined design with a proper composition (e.g., social data sets), while unstructured information misses the mark on unambiguous configuration and is commonly text-weighty (e.g., messages, web-based entertainment posts, pictures).
12. What is SQL, and how could it be utilized in information examination?
- • SQL (Organized Question Language) is a space-explicit language utilized for overseeing and questioning social data sets. Information experts use SQL to recover, control, and break down information put away in data sets, utilising orders like SELECT, Addition, UPDATE, and Erase.
13. What are some normal SQL orders utilised in information examination?
- • SELECT (recover information), WHERE (channel information), Gathering BY (bunch information), Request BY (sort information), JOIN (consolidate information from various tables), and total capabilities (e.g., aggregate, AVG, COUNT).
14. What is an SQL join, and how can it work?
- • A SQL join is utilized to consolidate lines from at least two tables in light of a connected segment between them. Normal types of joins include Internal JOIN, LEFT JOIN, RIGHT JOIN, and FULL External JOIN.
15. What is information fighting, and for what reason is it significant in information examination?
- • Information fighting (otherwise called information munging) is the method involved with cleaning, changing, and planning crude information for examination. It's significant because crude information is frequently untidy, conflicting, and deficient and should be organised and arranged appropriately for examination.
16. Make sense of the idea of dimensionality decreasing.
- • A dimensionality decrease is the most common way of lessening the number of factors (aspects) in a dataset while protecting its significant highlights. It's utilized to work on complex datasets, work on computational effectiveness, and stay away from overfitting in AI models.
17. What is a turn table, and how could it be utilized in information examination?
- • A turn table is an information outline device in accounting sheet programming (e.g., Succeed, Google Sheets) that permits clients to redesign and sum up information from a table into another table by pivoting its lines and sections to show helpful data.
18. What is the contrast between information mining and information warehousing?
- • Information mining is the most common way of finding examples, connections, and experiences from huge datasets, while information warehousing includes gathering, putting away, and overseeing organised information from different sources in a concentrated storehouse (information stockroom) for examination and revealing.
19. What is information administration, and for what reason is it significant in information examination?
- • Information administration is the general administration of the accessibility, ease of use, trustworthiness, and security of information utilized in an association. It's significant in information examination to guarantee information quality, consistency with guidelines, and arrangement with business objectives.
20. What are a few normal measurements used to assess the presentation of an information investigation project?
- • Exactness, accuracy, review, F1 score, ROC bend, AUC-ROC, disarray lattice, mean outright blunder (MAE), mean squared mistake (MSE), root mean squared mistake (RMSE).
21. What is a measurable model, and how could it be utilized in information examination?
- • A measurable model is a numerical portrayal of connections between factors in a dataset. It's utilized in information examination to make expectations, test theories, and construe experiences from information utilizing factual procedures and calculations.
22. What is straight relapse, and how can it work?
- • Direct relapse is a factual strategy used to show the connection between a dependent variable and at least one free factor by fitting a straight condition to the observed data of interest. It's utilised for anticipating ceaseless results.
23. What is strategic relapse, and how can it differ from direct relapse?
- • Strategic relapse is a factual strategy utilised for parallel characterization undertakings, where the dependent variable is downright with two potential results (e.g., yes/no, valid/bogus). Dissimilar to straight relapse, strategic relapse models the likelihood of a paired result utilising a calculated (sigmoid) capability.
24. What is grouping, and how could it be utilized in information examination?
- Bunching is a solo AI procedure used to bunch comparative information focuses together in light of their qualities or elements. It's utilized in information examination for fragmenting clients, recognizing designs, and finding stowed-away designs in information.
25. What is characterization, and how could it be utilized in information examination?
- • Grouping is a managed AI method used to sort pieces of information into predefined classes or classifications given their elements. It's utilized in information examination for anticipating straight-out results (e.g., spam/not spam, fake/authentic).
26. What is the contrast between directed and unaided learning?
- • In directed learning, the calculation gains from marked information (input-yield coordinates) and predicts results for new, concealed information. In unaided learning, the calculation gains from unlabeled information and finds examples, designs, or connections in the information.
27. What is the scourge of dimensionality, and how can it influence information investigation?
- • The scourge of dimensionality alludes to the difficulties and constraints that emerge while working with high-layered datasets, like expanded computational intricacy, sparsity of information, and trouble in imagining and deciphering results.
28. What is cross-approval, and for what reason is it significant in information examination?
- • Cross-approval is a strategy used to survey the presentation of prescient models by dividing the dataset into various subsets (folds), preparing the model on certain folds, and assessing it on the excess folds. It's critical to forestall overfitting and acquire dependable execution gauges.
29. What is overfitting, and how might it be forestalled in AI models?
- • Overfitting happens when a model figures out how to catch commotion or irregular changes in the prepared information, bringing about unfortunate speculation about new, concealed information. It very well may be forestalled by utilizing methods like cross-approval, regularization, including choice, and utilizing more information.
30. What is underfitting, and how might it be forestalled in AI models?
- • Underfitting happens when it is excessively easy to catch the hidden construction or examples in the information, bringing about a lacklustre showing on both the preparation and test datasets. It tends to be forestalled by utilizing more perplexing models, adding more elements, or decreasing regularization.
31. What is highlight design, and for what reason is it significant in information examination?
- • Highlight design is the most common way of making new elements or changing existing highlights in a dataset to work on the exhibition of AI models. It's significant because the decision and nature of elements incredibly influence the model's prescient power.
32. What is the reason for exploratory information examination (EDA) in information investigation?
- • Exploratory information examination (EDA) is the process of outwardly and genuinely investigating and summarising information to grasp its qualities, examples, circulations, and connections. It distinguishes fascinating patterns, exceptions, and possible bits of knowledge in the information.
33. What are a few normal procedures utilised in exploratory information examination (EDA)?
- • Outline insights, histograms, box plots, dissipate plots, relationship frameworks, heatmaps, match plots, and dimensionality decrease procedures (e.g., PCA, t-SNE).
34. What is the contrast between factual deduction and prescient display?
- • Measurable deduction includes making determinations or expectations about a population in light of test information and factual strategies, while prescient demonstrating includes building models to foresee future results given verifiable information and AI calculations.
35. What is a time series examination, and how could it be utilised in an information investigation?
- • Time series investigation is the most common way of examining and estimating consecutive information gathered over ordinary time stretches. It's utilised in information examination for demonstrating and anticipating time-subordinate examples, patterns, and irregularities in information (e.g., stock costs, weather condition gauges).
36. What are some normal time series anticipating strategies?
- • Moving midpoints, outstanding smoothing, autoregressive incorporated moving normal (ARIMA) models, occasional deterioration, and AI calculations (e.g., LSTM organisations).
37. What is feeling examination, and how could it be utilized in information investigation?
- • Feeling examination is the most common way of breaking down message information to decide the opinion or assessment communicated in a piece of message (e.g., good, pessimistic, unbiased). It's utilised in information examination for grasping general assessment, client criticism, and web-based entertainment.
38. What is web scratching, and how could it be utilized in information examination?
- • Web scratching is the most common way of separating information from sites utilising mechanised scripts or apparatuses. It's utilized in information examination to gather, parse, and break down information from the web for different purposes (e.g., statistical surveying, cutthroat investigation, content accumulation).
39. What is normal language handling (NLP), and how could it be utilized in information examination?
- • Regular language handling (NLP) is a part of computerised reasoning (man-made intelligence) that arranges the connection between PCs and people utilising normal language. It's utilized in information examination for errands like message order, opinion examination, named substance acknowledgment, and machine interpretation.
40. What are a few normal difficulties or restrictions of information investigation?
- • Information quality issues, absent or fragmented information, one-sided or unrepresentative examples, information protection and security concerns, moral contemplations, and the interpretability of intricate models.
41. What is the job of area information in information examination?
- • Space information alludes to mastery or comprehension of the particular business, field, or branch of knowledge applicable to the information being investigated. It's significant in information examination for deciphering results, recognising significant examples, and producing noteworthy bits of knowledge.
42. What are a few normal information examination devices and programmes utilised in business?
- • Microsoft Succeed, SQL information bases (e.g., MySQL, PostgreSQL), Python (Pandas, NumPy, sci-kit-learn), R, Scene, Power BI, SAS, SPSS, and MATLAB.
43. What is the contrast between information investigation and information science?
- • Information examination centres around investigating and deciphering information to recognise patterns, examples, and experiences that illuminate business choices, while information science includes applying advanced strategies and calculations to remove information and bits of knowledge from information utilising factual, numerical, and computational techniques.
44. What is the Fresh DM procedure, and how could it be utilized in information examination projects?
- • The Fresh DM (Cross-Industry Standard Cycle for Information Mining) strategy is a generally involved system for directing information mining and information examination projects. It comprises six stages: business getting it, information figuring out, information planning, demonstrating, assessment, and arrangement.
45. What is the distinction between group handling and ongoing handling in information examination?
- • Clump handling includes handling information in enormous, discrete clusters or gatherings at planned stretches, while constant handling includes handling information ceaselessly or continuously as it opens up.
46. What are a few normal information representation best practices?
- • Pick suitable perception types, utilise clear and unmistakable titles and marks, keep away from mess and pointless components, keep up with consistency in plans and variety plans, give setting and explanations, and focus on clarity and availability.
47. What is the information science lifecycle, and how can it contrast with the customary programming advancement lifecycle?
- • The information science lifecycle comprises stages like issue definition, information assortment, information readiness, displaying, assessment, and arrangement. It contrasts with the conventional programming improvement lifecycle regarding the iterative and exploratory nature of information investigation, the significance of information quality and area mastery, and the attention to creating bits of knowledge and expectations from information.
48. What is information security, and for what reason is it significant in information examination?
- • Information security alludes to the insurance of delicate or individual data from unapproved access, use, or exposure. It's significant in information examination to guarantee consistency with guidelines (e.g., GDPR, HIPAA), keep up with trust with partners, and moderate dangers connected with information breaks or abuse.
49. What are a few normal moral contemplations in information examination?
- • Regard for protection and privacy, reasonableness and predisposition in information assortment and examination, straightforwardness and responsibility in direction, informed assent and information proprietorship, and mindful utilisation of information for moral purposes.
50. What are the methodologies for successfully conveying information examination discoveries to non-specialised partners?
- • Utilise clear and compact language, keep away from specialised language, centre around key bits of knowledge and noteworthy suggestions, give perceptions and guides to represent discoveries, tailor the message to the crowd's degree of understanding and needs, and energise questions and input.
51. What is the contrast between organized and unstructured information?
- • Organized information is coordinated and put away in a predefined design, frequently in data sets with fixed outlines, while unstructured information comes up short on unambiguous organization and is normally text-weighty, for example, messages, web-based entertainment posts, and pictures.
52. What is the contrast between an information investigator and an information researcher?
- • Information investigators center around dissecting and deciphering information to determine bits of knowledge and backing direction, frequently utilizing measurable and perception strategies. Information researchers, then again, utilize progressed calculations and AI methods to extricate information and experiences from information, frequently for prescient displaying and streamlining.
53. What are a few normal information designs utilized in information examination?
- • Normal information designs incorporate CSV (Comma-Isolated Values), JSON (JavaScript Item Documentation), XML (eXtensible Markup Language), Parquet, Avro, and HDF5 (Progressive Information Arrangement variant 5).
54. Make sense of the idea of information standardization and its significance.
- • Information standardization is the most common way of sorting out information in a data set to decrease overt repetitiveness and reliance by partitioning huge tables into more modest, related tables and characterizing connections between them. It's significant for further developing information uprightness, diminishing information duplication, and advancing data set execution.
55. What is information deduplication, and for what reason is it significant in information examination?
- • Information deduplication is the method involved with recognizing and eliminating copy or excess records from a dataset. It's significant in information examination to guarantee information quality, abstain from twofold counting, and work on the exactness of investigation results.
56. What is information ascription, and how could it be utilized in information examination?
- • Information ascription is the most common way of filling in missing qualities in a dataset utilizing different strategies, like mean, middle, mode attribution, forward or in reverse filling, or AI calculations. It's utilized in information examination to deal with missing information and forestall predisposition in examination results.
57. What is information mining, and how could it be not the same as information investigation?
- • Information mining is the most common way of finding examples, patterns, and experiences from enormous datasets utilizing factual, numerical, and AI methods. It's unique in information examination, which includes deciphering and extricating significant bits of knowledge from information to help direction.
58. What is the contrast between information cleaning and information preprocessing?
- • Information cleaning includes recognizing and remedying blunders, irregularities, and missing qualities in a dataset, while information preprocessing includes changing and planning information for examination, including standardization, scaling, highlight designing, and dimensionality decrease.
59. What is the scourge of dimensionality, and how can it influence information investigation?
- • The scourge of dimensionality alludes to the difficulties and constraints that emerge while working with high-layered datasets, like expanded computational intricacy, sparsity of information, and trouble in imagining and deciphering results.
60. What is the distinction between group handling and ongoing handling in information examination?
- • Clump handling includes handling information in enormous, discrete clusters or gatherings at planned stretches, while constant handling includes handling information ceaselessly or close continuously as it opens up.
61. What is the reason for exploratory information examination (EDA) in information investigation?
- • Exploratory information examination (EDA) is the course of outwardly and genuinely investigating and summing up information to grasp its qualities, examples, circulations, and connections. It distinguishes fascinating patterns, exceptions, and possible bits of knowledge in the information.
62. What are a few normal procedures utilized in exploratory information examination (EDA)?
- • Outline insights, histograms, box plots, dissipate plots, relationship frameworks, heatmaps, match plots, and dimensionality decrease procedures (e.g., PCA, t-SNE).
63. What is the contrast between factual deduction and prescient displaying?
- • Measurable deduction includes making determinations or expectations about a populace in light of test information and factual strategies, while prescient demonstrating includes building models to foresee future results given verifiable information and AI calculations.
64. What is time series examination, and how could it be utilized in information investigation?
- • Time series investigation is the most common way of examining and estimating consecutive information focuses gathered at ordinary time stretches. It's utilized in information examination for demonstrating and anticipating time-subordinate examples, patterns, and irregularity in information (e.g., stock costs, weather conditions gauges).
65. What are some normal time series anticipating strategies?
- • Moving midpoints, outstanding smoothing, autoregressive incorporated moving normal (ARIMA) models, occasional deterioration, and AI calculations (e.g., LSTM organizations).
66. What is feeling examination, and how could it be utilized in information investigation?
- • Feeling examination is the most common way of breaking down message information to decide the opinion or assessment communicated in a piece of message (e.g., good, pessimistic, unbiased). It's utilized in information examination for grasping general assessment, client criticism, and web-based entertainment feeling.
67. What is web scratching, and how could it be utilized in information examination?
- • Web scratching is the most common way of separating information from sites utilizing mechanized scripts or apparatuses. It's utilized in information examination to gather, parse, and break down information from the web for different purposes (e.g., statistical surveying, cutthroat investigation, content accumulation).
68. What is normal language handling (NLP), and how could it be utilized in information examination?
- • Regular language handling (NLP) is a part of computerized reasoning (man-made intelligence) that arrangements the connection between PCs and people utilizing normal language. It's utilized in information examination for errands like message order, opinion examination, named substance acknowledgment, and machine interpretation.
69. What are a few normal difficulties or restrictions of information investigation?
- • Information quality issues, absent or fragmented information, one-sided or unrepresentative examples, information protection and security concerns, moral contemplations, and interpretability of intricate models.
70. What is the job of area information in information examination?
- • Space information alludes to mastery or comprehension of the particular business, field, or branch of knowledge applicable to the information being investigated. It's significant in information examination for deciphering results, recognizing significant examples, and producing noteworthy bits of knowledge.
71. What are a few normal information examination devices and programming utilized in the business?
- • Microsoft Succeed, SQL information bases (e.g., MySQL, PostgreSQL), Python (pandas, NumPy, sci-kit-learn), R, Scene, Power BI, SAS, SPSS, MATLAB.
72. What is the contrast between information investigation and information science?
- • Information examination centers around investigating and deciphering information to recognize patterns, examples, and experiences that illuminate business choices, while information science includes applying progressed strategies and calculations to remove information and bits of knowledge from information utilizing factual, numerical, and computational techniques.
73. What is the Fresh DM procedure, and how could it be utilized in information examination projects?
- • The Fresh DM (Cross-Industry Standard Cycle for Information Mining) strategy is a generally involved system for directing information mining and information examination projects. It comprises of six stages: business getting it, information figuring out, information planning, demonstrating, assessment, and arrangement.
74. What are the methodologies for successfully conveying information examination discoveries to non-specialized partners?
- • Utilize clear and compact language, keep away from specialized language, center around key bits of knowledge and noteworthy suggestions, give perceptions and guides to represent discoveries, tailor the message to the crowd's degree of understanding and needs, and energize questions and input.
75. What is the reason for A/B testing, and how could it be utilized in information examination?
- • A/B testing is a controlled trial where at least two variations of a site page, email, or other computerized resource are contrasted to figure out which one performs better concerning a predefined metric (e.g., change rate, active clicking factor). It's utilized in information examination to survey the effect of changes or mediations and settle on information-driven choices.
76. What is the contrast between directed and unaided learning?
- • In directed learning, the calculation gains from marked information (input-yield coordinates) and predicts results for new, concealed information. In unaided learning, the calculation gains from unlabeled information and finds examples, designs, or connections in the information.
77. What is overfitting, and how might it be forestalled in AI models?
- • Overfitting happens when a model figures out how to catch commotion or irregular changes in the prepared information, bringing about unfortunate speculation about new, concealed information. It very well may be forestalled by utilizing methods like cross-approval, regularization, including choice, and utilizing more information.
78. What is underfitting, and how might it be forestalled in AI models?
- • Underfitting happens when it is excessively easy to catch the hidden construction or examples in the information, bringing about a lacklustre showing on both the preparation and test datasets. It tends to be forestalled by utilizing more perplexing models, adding more elements, or decreasing regularization.
79. What is highlight design, and for what reason is it significant in information examination?
- • Highlight design is the most common way of making new elements or changing existing highlights in a dataset to work on the exhibition of AI models. It's significant because the decision and nature of elements incredibly influence the model's prescient power.
80. What is cross-approval, and for what reason is it significant in information examination?
- • Cross-approval is a strategy used to survey the presentation of prescient models by dividing the dataset into various subsets (folds), preparing the model on certain folds, and assessing it on the excess folds. It's critical to forestall overfitting and acquire dependable execution gauges.
81. What are a few normal measurements used to assess the exhibition of AI models?
- • Exactness, accuracy, review, F1 score, ROC bend, AUC-ROC, disarray lattice, mean outright blunder (MAE), mean squared mistake (MSE), root mean squared mistake (RMSE).
82. What is strategic relapse, and how can it differ from direct relapse?
- • Strategic relapse is a factual strategy utilised for parallel characterization undertakings, where the dependent variable is downright with two potential results (e.g., yes/no, valid/bogus). Dissimilar to straight relapse, strategic relapse models the likelihood of a paired result utilising a calculated (sigmoid) capability.
83. What is the motivation behind dimensionality decrease, and how could it be utilized in information examination?
- • A dimensionality decrease is the most common way of lessening the number of factors (aspects) in a dataset while protecting its significant highlights. It's utilized to work on complex datasets, work on computational effectiveness, and stay away from overfitting in AI models.
84. What is grouping, and how could it be utilized in information examination?
- • Bunching is a solo AI procedure used to bunch comparative information focuses together in light of their qualities or elements. It's utilized in information examination for fragmenting clients, recognizing designs, and finding stowed-away designs in information.
85. What is affiliation rule mining, and how could it be utilized in information examination?
- • Affiliation rule mining is an information mining procedure used to find fascinating connections, examples, or relationships among factors in enormous datasets. It's utilized in information examination for market container examination, proposal frameworks, and recognizing continuous itemsets.
86. What is an anomaly location, and for what reason is it significant in information examination?
- • Anomaly recognition is the method involved with recognizing and taking care of information focuses that go amiss fundamentally from the remainder of the dataset. It's significant in information examination to guarantee information quality, distinguish blunders or oddities, and keep them from slanting examination results.
87. What is text mining, and how could it be utilized in information examination?
- • Text mining (otherwise called text investigation) is the method involved with removing significant data and bits of knowledge from unstructured text information, for example, messages, client surveys, and web-based entertainment posts. It's utilised in information examination for feeling investigation, theme display, and data extraction.
88. What is cooperative separating, and how could it be utilized in information examination?
- • Cooperative separating is a suggestion framework method that predicts the inclinations or evaluations of clients for things (e.g., films, items) in light of the inclinations or evaluations of comparative clients. It's utilized in information examination for customized suggestions and content sifting.
89. What is information administration, and for what reason is it significant in information examination?
- • Information administration is the general administration of the accessibility, ease of use, trustworthiness, and security of information utilized in an association. It's significant in information examination to guarantee information quality, consistency with guidelines, and arrangement with business objectives.
90. What are a few normal information representation best practices?
- • Pick suitable perception types, utilise clear and unmistakable titles and marks, keep away from mess and pointless components, keep up with consistency in plans and variety plans, give setting and explanations, and focus on clarity and availability.
91. What are a few normal information representation devices and libraries utilised in business?
- • Scene, Power BI, matplotlib, Seaborn, ggplot2, D3.js, Plotly, Bokeh, Highcharts, and Google Outlines.
92. What is the motivation behind narrating in information examination, and how could it be utilized?
- • Narrating in an information examination includes introducing examination results and experiences in a story organisation to draw in and convince partners, work with understanding, and drive navigation. It's utilised to impart the specific circumstances, importance, and ramifications of investigational discoveries successfully.
93. What are a few normal information examination methods utilised in monetary investigation?
- • Monetary proportions examination, pattern investigation, similar examination, income examination, risk appraisal, and valuation models (e.g., limited income, cost-profit proportion).
94. What are a few normal information examination methods utilised in showcasing examinations?
- • Client division, partner investigation, client lifetime esteem (CLV) examination, market bin examination, A/B testing, effort execution investigation, and virtual entertainment examination.
95. What are a few normal information examination procedures utilised in medical services investigations?
- • Patient results examination, illness predominance investigation, treatment adequacy investigation, clinical preliminary examination, electronic wellbeing record (EHR) investigation, and medical care cost investigation.
96. What is information security, and for what reason is it significant in information examination?
- • Information security alludes to the insurance of delicate or individual data from unapproved access, use, or exposure. It's significant in information examination to guarantee consistency with guidelines (e.g., GDPR, HIPAA), keep up with trust with partners, and moderate dangers connected with information breaks or abuse.
97. What are a few normal moral contemplations in information examination?
- • Regard for protection and privacy, reasonableness and predisposition in information assortment and examination, straightforwardness and responsibility in direction, informed assent and information proprietorship, and mindful utilisation of information for moral purposes.
98. What are a few normal information examination challenges looked at by associations?
- • Information storehouses and joining issues; unfortunate information quality and consistency; absence of talented information experts and information researchers; information protection and security concerns; and trouble interpreting examination results into noteworthy bits of knowledge.
99. What is the job of information administration in information examination projects?
- • Information administration includes laying out approaches, strategies, and controls for overseeing and guaranteeing the quality, trustworthiness, and security of information utilized in an association. In information examination projects, information administration keeps up with information consistency, consistency with guidelines, and arrangement with business objectives.
100. What are a few procedures for really overseeing and reporting information investigation projects?
- • Characterise clear venture targets and extensions; record information sources and suppositions; layout information quality norms and systems; keep up with adaptation control of datasets and investigation code; archive examination approaches and suspicions; and team up with partners for input and approval.
Machine Learning & AI Interview Questions And Answers
1. What is AI, and how can it contrast with customary programming?
- • AI is a subset of man-made reasoning that empowers frameworks to gain and improve on a fact without being unequivocally modified. Conventional programming includes composing unequivocal directions for a PC to follow.
2. What are the various kinds of AI?
- • Regulated Learning, Unaided Learning, Semi-Managed Learning, and Support Learning.
3. Make sense of the predisposition-fluctuation tradeoff in AI.
- • The Predisposition Change tradeoff alludes to the harmony between a model's capacity to catch the hidden examples in the information (low inclination) and its aversion to clamour and vacillations in the information (low fluctuation).
4. What is the contrast between directed and unaided learning?
- • Directed gaining includes gaining from marked information, where the calculation figures out how to foresee a result based on the based on the given information. Unaided gaining includes gaining from unlabeled information to find examples or designs inside the information.
5. What is the contrast between arrangement and relapse?
- • Grouping includes foreseeing clear-cut names or classes (e.g., spam/not spam), while relapse includes anticipating consistent mathematical qualities (e.g., house costs).
6. What is the motivation behind cross-approval in AI?
- • Cross-approval is a procedure used to survey the presentation of a model by partitioning the dataset into numerous subsets, preparing the model on certain subsets, and assessing it on the excess subsets. It helps gauge the model's exhibition of concealed information and forestall overfitting.
7. What is regularization, and for what reason is it utilized in AI?
- • Regularization is a procedure used to forestall overfitting by adding a punishment term to the model's expense capability, putting excessively complex models down. It further develops the model's speculation execution on inconspicuous information.
8. What is angle drop, and how could it be utilized in AI?
- • Slope plunge is an enhancement calculation used to limit the expense capability of a model by iteratively changing the model's boundaries toward the steepest plummet of the inclination.
9. Make sense of the idea of component design in AI.
- • Highlight design is the most common way of making new elements or changing existing highlights in a dataset to work on the exhibition of AI models. It includes choosing, removing, and changing applicable highlights to more readily address the fundamental examples in the information.
10. What is the scourge of dimensionality, and how can it influence AI?
- • The scourge of dimensionality alludes to the difficulties and constraints that emerge while working with high-layered datasets, like expanded computational intricacy, sparsity of information, and trouble in imagining and deciphering results.
11. What is the distinction between packing and helping with outfit learning?
- • Sacking (bootstrap amassing) includes preparing numerous autonomous models on various subsets of the information and averaging their expectations to further develop execution. Helping includes consecutively preparing numerous frail models, where each resulting model spotlights the models that the past models misclassified.
12. What is the contrast between accuracy and review in order?
- • Accuracy estimates the extent of genuine positive expectations among all certain forecasts made by the model, while review estimates the extent of genuine positive forecasts among all real-sure occasions in the information.
13. What is the ROC bend, and what does it address in AI?
- • The ROC (Beneficiary Working Trademark) bend is a graphical plot that shows the compromise between the genuine positive rate (responsiveness) and bogus positive rate (1-particularity) of a grouping model across various edge values.
14. What is the F1 score, and for what reason is it utilized in AI?
- • The F1 score is the consonant mean of accuracy and review, utilised as a solitary measurement to assess the exhibition of a characterization model. It adjusts both accuracy and review, giving a more exhaustive proportion of model execution.
15. Make sense of the idea of a choice tree in AI.
- • A choice tree is a prescient model that guides elements to target marks by recursively dividing the information into subsets in light of the worth of a picked highlight. Each inner hub addresses an element, each branch addresses a choice given that component, and each leaf hub addresses an objective mark.
16. What is the distinction between a choice tree and an irregular wood?
- • A choice tree is a solitary tree-based model that makes expectations given a succession of choices, while an irregular woodland is a troupe of various choice trees prepared on various subsets of the information, where forecasts are found in the middle value of or collected across all trees.
17. What is K-implies bunching, and how can it work?
- • K-implies grouping is a solo learning calculation used to parcel data of interest into K bunches in light of their elements. It works by iteratively allocating information focusing on the closest group centroid and refreshing the centroid given the mean of the appointed data of interest.
18. What is the contrast between accuracy and review in a grouping?
- • Accuracy in bunching estimates the immaculateness of groups, addressing the extent of information focuses doled out to a bunch that has a place with that bunch. Review in grouping estimates the fulfillment of bunches, addressing the extent of genuine information focuses doled out to a bunch.
19. Make sense of the idea of supporting learning in AI.
- • Support learning is a kind of AI where a specialist figures out how to settle on choices by communicating with a climate and getting criticism as remunerations or punishments. The specialist figures out how to expand aggregate prizes after some time by investigating various activities and gaining from the results of those activities.
20. What is profound realising, and how can it differ from conventional AI?
- • Profound learning is a subset of AI that includes preparing counterfeit brain networks with numerous layers (profound structures) to gain complex examples and portrayals from information. It differs from customary AI in its capacity to naturally learn various levelled portrayals of information without manual element design.
21. What is a brain organisation, and how can it work?
- • A brain network is a computational model roused by the design and capability of the human cerebrum, comprising interconnected hubs (neurons) coordinated into layers. Every neuron gets input signals, applies a change capability, and passes the result to the following layer. Brain networks are prepared to utilise enhancement calculations to change the associations (loads) between neurons to limit expectation mistakes.
22. What is backpropagation, and how could it be utilized in preparing brain organizations?
- • Backpropagation is a calculation used to prepare brain networks by iteratively changing loads of associations between neurons to limit the contrast between anticipated and genuine results. It works by engendering the mistake in reverse from the result layer to the information layer, refreshing the loads, and utilising slope drop.
23. What is a convolutional brain organization (CNN), and what is it utilized for?
- • A convolutional brain organization (CNN) is a kind of brain network intended to process and examine organized matrix-like information, like pictures. It comprises different layers of convolutional and pooling activities, trailed by completely associated layers for characterization or relapse errands. CNNs are generally utilized in picture acknowledgment, object discovery, and picture division errands.
24. What is a repetitive brain organization (RNN), and what is it utilized for?
- • An intermittent brain organization (RNN) is a kind of brain network intended to process and investigate successive information, like time series or regular language. It contains repetitive associations that permit data to persevere over the long run, making it appropriate for errands like succession expectation, language demonstrating, and time series determining.
25. What is the move realizing, and how could it be utilized in AI?
- • Move learning is an AI strategy where information acquired from preparing a model for one undertaking is moved and applied to an alternate yet related task. It includes adjusting pre-prepared models or involving them as element extractors to work on the exhibition of models on new undertakings with restricted information.
26. What is the job of enactment capabilities in brain organisations?
- • Actuation capabilities bring non-linearity into brain organisations, permitting them to display complex connections and make non-direct changes of information. They decide the result of individual neurons and the general organization's capacity to inexact complex capabilities.
27. What are some normal enactment capabilities utilised in brain organisations?
- • Sigmoid, Tanh (exaggerated digression), ReLU (redressed direct unit), Broken ReLU, ELU (remarkable straight unit), Softmax.
28. What is the evaporating angle issue in brain organisations?
- • The evaporating angle issue happens during preparation when the slopes of the misfortune capability regarding the model's boundaries become tiny (near nothing), prompting slow or ineffectual learning in profound brain organisations.
29. What is the contrast between clump inclination drop, stochastic slope plunge, and small bunch angle plummet?
- • Group slope plunge processes the inclination of the expense capability utilising the whole dataset; stochastic slope drop figures the inclination utilising a solitary, haphazardly chosen piece of data of interest; and little clump angle plummet registers the inclination utilising a little arbitrarily chosen subset (smaller than usual cluster) of the information.
30. What is the distinction between a generative model and a discriminative model?
- • A generative model learns the joint likelihood circulation of information elements and target names, permitting it to produce new examples from the learned dispersion. A discriminative model learns the contingent likelihood circulation of target marks given input highlights, zeroing in on recognising various classes.
31. What is the contrast among managed and unaided generative models?
- • Directed generative models figure out how to produce new examples moulded on unambiguous information marks or classes, while solo generative models figure out how to create tests without express names or classes, zeroing in on catching the hidden design of the information.
32. What is the distinction between generative antagonistic organisations (GANs) and variational autoencoders (VAEs)?
- • GANs comprise two brain organisations, a generator and a discriminator, prepared adversarially to create sensible examples from a given circulation. VAEs are probabilistic generative models that figure out how to rough out genuine information dissemination by encoding input tests into a dormant space and interpreting them back into the first space.
33. What is the motivation behind the dimensionality decrease in AI?
- • A dimensionality decrease is the most common way of diminishing the quantity of information highlights or factors in a dataset while safeguarding its significant qualities and examples. It's utilized to work on complex datasets, work on computational effectiveness, and stay away from overfitting in AI models.
34. What are some normal dimensionality decrease methods utilised in AI?
- • Head Part Examination (PCA), t-Circulated Stochastic Neighbour Installing (t-SNE), Solitary Worth Disintegration (SVD), Direct Discriminant Investigation (LDA).
35. What is the reason for normal language handling (NLP) in AI?
- • Regular language handling (NLP) is a part of man-made reasoning that deals with communication among PCs and people utilising normal language. It's utilized in AI for undertakings like message grouping, opinion examination, named element acknowledgment, and machine interpretation.
36. What are a few normal difficulties in regular language handling (NLP)?
- • Uncertainty and setting reliance in language, information sparsity and commotion, dealing with different dialects and lingos, grasping colloquial articulations and shoptalk, and safeguarding protection and security in text information.
37. What is the distinction between word implanting and one-hot encoding in regular language handling (NLP)?
- • Word implanting is a thick portrayal of words in a consistent vector space, catching semantic connections between words in view of their specific situation. One-hot encoding is a meager portrayal where each word is addressed as a parallel vector with a solitary '1' demonstrating its presence in the jargon.
38. What is move-realising in regular language handling (NLP), and how could it be utilised?
- • Move learning in NLP includes utilising pre-prepared language models (e.g., BERT, GPT) to perform downstream errands with restricted marked information. By calibrating the pre-prepared models on unambiguous errands, move learning empowers quicker and more powerful learning for different NLP applications.
39. What is called substance acknowledgment (NER) in regular language handling (NLP)?
- • Named element acknowledgment (NER) is the undertaking of recognising and arranging named substances (e.g., people, associations, areas) referenced in unstructured text information. It's utilized in data extraction, text mining, and record outline assignments.
40. What is opinion examination, and how could it be utilized in regular language handling (NLP)?
- • Feeling examination is the undertaking of breaking down message information to decide the opinion or assessment communicated in a piece of message (e.g., good, pessimistic, impartial). It's utilized in NLP for grasping popular assessment, client criticism, and web-based entertainment opinion.
41. What are some normal AI structures and libraries utilised in the business?
- • TensorFlow, PyTorch, scikit-learn, Keras, Apache Flash MLlib, Microsoft Mental Tool compartment (CNTK), Theano, and MXNet.
42. What is the motivation behind logical simulated intelligence (XAI), and for what reason is it significant?
- • Reasonable computer-based intelligence (XAI) expects to pursue AI models, and their choices are interpretable and justifiable to people. It's significant for building trust in simulated intelligence frameworks, guaranteeing responsibility, and distinguishing predispositions or mistakes in model expectations.
43. What is antagonistic AI, and how can it work?
- • Ill-disposed AI is a field of study that investigates the weaknesses of AI models to antagonistic assaults or noxious information sources intended to beguile the model and produce mistaken forecasts. Antagonistic assaults can take advantage of shortcomings in model designs or prepare calculations to control model results.
44. What is mechanised AI (AutoML), and how can it work?
- • Robotized AI (AutoML) alludes to the method involved with computerising the start-to-finish cycle of applying AI to true issues, including information preprocessing, highlight designing, model choice, hyperparameter tuning, and arrangement. AutoML apparatuses and stages expect to democratise AI by decreasing the requirement for manual mediation and mastery.
45. What is the job of area information in AI and simulated intelligence projects?
- • Space information alludes to aptitude or comprehension of the particular business, field, or branch of knowledge applicable to the information being examined. It's significant in AI and simulated intelligence projects for deciphering results, recognising significant examples, and producing noteworthy bits of knowledge that line up with space-specific objectives and requirements.
46. What is the distinction between logic and interpretability in AI and computer-based intelligence?
- • Reasonableness alludes to the capacity to give clarifications or legitimizations to demonstrate forecasts or choices in a human-justifiable configuration. Interpretability alludes to the simplicity of understanding the interior functions and systems of a model, including how sources of information are changed into yields.
47. What are a few normal moral contemplations in AI and simulated intelligence?
- • Decency and predisposition in information assortment and model forecasts; straightforwardness and responsibility in direction; security and information assurance; interpretability and reasonableness of model expectations; and dependable utilisation of simulated intelligence advancements.
48. What is the job of reasonableness and predisposition moderation strategies in AI and artificial intelligence?
- • Reasonableness and predisposition alleviation procedures plan to address inclinations and guarantee decency in AI models and simulated intelligence frameworks, especially in delicate spaces like money, medical services, and law enforcement. These procedures incorporate reasonableness, mindful calculations, predisposition discovery and amendment, and various delegated procedures for preparing information.
49. What are a few normal difficulties or limits of AI and simulated intelligence?
- • Information quality and amount; one-sided or unrepresentative datasets; interpretability and reasonableness of models; moral and cultural ramifications; arrangement and adaptability; and security and protection concerns.
50. What are a few techniques for successfully imparting AI and computer-based intelligence discoveries to non-specialised partners?
- • Utilise clear and succinct language, keep away from specialised language, give representations and guides to delineate discoveries, centre around key bits of knowledge and significant proposals, tailor the message to the crowd's degree of understanding and needs, and empower questions and criticism.
51. What is group realising, and how can it work on model execution?
- • Gathering learning includes consolidating numerous singular models to make a more grounded, more vigorous model. It further develops execution by decreasing overfitting, expanding speculation, and catching assorted designs in the information.
52. What are some normal gathering learning strategies?
- • Packing (Bootstrap Amassing), Supporting (e.g., AdaBoost, Angle Helping), Stacking, and Arbitrary Timberlands.
53. What is the contrast between sacking and helping in gathering learning?
- • Sacking includes preparing various autonomous models based on various subsets of the information and averaging their expectations. Helping includes preparing different frail models successively, where each ensuing model spotlights on the models that the past models misclassified.
54. What is the reason for hyperparameter tuning, and how could it be used in AI?
- • Hyperparameter tuning includes choosing the ideal hyperparameters for an AI model to work on its exhibition. It's performed utilizing strategies like framework search, arbitrary hunt, or Bayesian enhancement to investigate the hyperparameter space and track down the best mix.
55. What is the distinction among hyperparameters and boundaries in AI?
- • Hyperparameters are settings or designs that control the growing experience of a model, for example, the learning rate or the quantity of secret layers in a brain organization. Boundaries are the factors or loads advanced by the model during preparation, addressing the hidden examples in the information.
56. What is the job of regularisation in AI, and for what reason is it significant?
- • Regularization is a procedure used to forestall overfitting by adding a punishment term to the model's expense capability, putting excessively complex models down. It's significant for further developing the model's speculation execution on concealed information and lessening change.
57. What is the contrast between L1 regularisation and L2 regularisation?
- • L1 regularisation (Tether) adds the outright upsides of the loads as a punishment term, advancing sparsity and element determination. L2 regularisation (Edge) adds the squared upsides of the loads as a punishment term, forestalling huge loads and decreasing aversion to exceptions.
58. What is the contrast between clump inclination drop, stochastic slope plunge, and small bunch angle plummet?
- • Group slope plunge processes the inclination of the expense capability utilising the whole dataset; stochastic slope drop figures the inclination utilising a solitary, haphazardly chosen piece of data of interest; and little clump angle plummet registers the inclination utilising a little arbitrarily chosen subset (smaller than usual cluster) of the information.
59. What is the job of enactment capabilities in brain organisations, and for what reason would they say they are fundamental?
- • Actuation capabilities bring non-linearity into brain organisations, permitting them to display complex connections and make non-direct changes of information. They're fundamental for empowering brain organisations to actually learn and have inconsistent capabilities.
60. What are some normal enactment capabilities utilized in brain organizations, and when are they utilized?
- • Sigmoid and Tanh (exaggerated digression) are utilized in the secret layers of shallow brain organizations. ReLU (Corrected Direct Unit) and its variations (e.g., Cracked ReLU, ELU) are usually utilized in profound brain networks for their quicker assembly and sparsity-prompting properties.
61. What is clump standardisation, and how can it work in brain organisations?
- • Clump standardization is a procedure used to work on the soundness and execution of profound brain networks by normalizing the initiations of each layer. It works by normalising the mean and difference of the actuations inside every small-scale bunch during preparation, decreasing the inner covariate shift, and speeding up intermingling.
62. What is the disappearing angle issue in brain organizations, and how could it be relieved?
- • The disappearing slope issue happens during preparation when the angles of the misfortune capability regarding the model's boundaries become tiny (near nothing) in profound brain organisations, prompting slow or incapable learning. It's moderated by utilising methods like cautious instatement of loads, utilising actuation works that relieve evaporating inclinations (e.g., ReLU), and applying standardisation strategies (e.g., cluster standardisation).
63. What is dropout, and how can it work in brain organisations?
- • Dropout is a regularisation method used to forestall overfitting in brain networks by haphazardly dropping (setting to nothing) a negligible part of the neurons in each layer during preparation. It keeps the organisation from depending a lot on unambiguous neurons or highlights, compelling it to learn more heartfelt portrayals.
64. What is move-realising, and how could it be utilised in AI?
- • Move learning is an AI procedure where information acquired from preparing a model on one errand is moved and applied to an alternate yet related task. It includes adjusting pre-prepared models or involving them as element extractors to work on the exhibition of models on new undertakings with restricted information.
65. What are some normal exchange learning approaches utilised in profound learning?
- • Calibrating pre-prepared models (e.g., changing the model's engineering and refreshing loads), highlight extraction (e.g., involving pre-prepared models as fixed include extractors), and area variation (e.g., learning space invariant portrayals).
66. What is the job of information expansion in profound learning, and for what reason is it significant?
- • Information expansion is a strategy used to misleadingly build the size and variety of prepared information by applying irregular changes (e.g., pivot, interpretation, turning) to existing information tests. It's significant for working on model speculation, power, and execution, particularly while preparing profound brain networks with restricted marked information.
67. What are a few normal information expansion methods utilised in picture-order undertakings?
- • Revolution, interpretation, scaling, flipping (even and vertical), trimming, shearing, brilliance change, contrast change, adding clamour.
68. What is the distinction between generative and discriminative models in AI?
- • Generative models get familiar with the joint likelihood dissemination of information highlights and target marks, permitting them to produce new examples from the learned conveyance. Discriminative models become familiar with the contingent likelihood dissemination of target names given input highlights, zeroing in on recognising various classes.
69. What are a few normal instances of generative models in AI?
- • Generative Ill-disposed Organisations (GANs), Variational Autoencoders (VAEs), Generative Antagonistic Impersonation Learning (GAIL), and Markov Chain Monte Carlo (MCMC) strategies.
70. What are a few normal instances of discriminative models in AI?
- • Calculated Relapse, Backing Vector Machines (SVM), Choice Trees, Irregular Woods, Slope Supporting Machines (GBM), and Convolutional Brain Organisations (CNNs) for grouping undertakings.
71. What is the motivation behind autoencoders in profound learning, and how would they work?
- • Autoencoders are brain network structures utilised for solo learning and dimensionality reduction. They comprise an encoder network that guides input information to a dormant space portrayal and a decoder network that recreates the information from the inert space portrayal.
72. What is the contrast between a denoising autoencoder and a variational autoencoder?
- • A denoising autoencoder is prepared to reproduce clean information from boisterous or tainted input information, assisting with learning hearty portrayals. A variational autoencoder is a probabilistic generative model that figures out how to estimate the genuine information circulation by encoding input tests into an inactive space and interpreting them back into the first space, considering the age of new examples.
73. What is the contrast between managed learning and support learning?
- • Administered gaining includes gaining from named information to foresee a result in light of information yield matches. Support learning includes figuring out how to pursue consecutive choices by communicating with a climate and getting criticism as remunerations or punishments.
74. What are a few normal uses of support learning?
- • game playing (e.g., AlphaGo), advanced mechanics (e.g., automated control), independent vehicles (e.g., self-driving vehicles), proposal frameworks, and money (e.g., algorithmic exchanging).
75. What is a strategy to support learning, and how can it work?
- • Strategy slope support learning is a sort of support realisation where a specialist learns an arrangement (a planning from states to activities) by straightforwardly improving the normal combined reward. It involves slope-raising to refresh the arrangement boundaries towards the path that builds the normal prize.
76. What is the job of investigation and abuse in supporting learning?
- • Investigation includes making moves to assemble data about the climate and find possibly better approaches or techniques. Double-dealing includes making moves given ongoing information to boost transient prizes. Adjusting investigation and double-dealing is vital for successful and dynamic learning.
77. What is the distinction between esteem-based and strategy-based support learning techniques?
- • Esteem-based support learning strategies: gain proficiency with the worth capability (the normal combined reward) related to various states or state-activity coordinates and use it to choose activities. Strategy-based support learning techniques get familiar with the arrangement straightforwardly (from states to activities) without unequivocally processing the worth capability.
78. What is the Monte Carlo technique, and how could it be utilized in supporting learning?
- • The Monte Carlo strategy is a factual procedure used to gauge the normal combined price of an approach by examining directions (groupings of states and activities) from the climate and averaging their profits. It's utilized in support learning for strategy assessment and learning without a model of the climate.
79. What is the Q-realising calculation, and how can it work to support learning?
- • Q-learning is a sans-model support learning calculation that learns the activity esteem capability (the normal combined compensation of making a move in a given state) by refreshing Q-values iteratively given the noticed rewards and changes. It's utilized to track down an ideal arrangement by choosing activities with the most noteworthy Q-values.
80. What is profound Q-realising, and how can it develop customary Q-learning?
- • Profound Q-learning is a variation of Q-discovering that utilizes profound brain organizations to estimate the activity esteem capability (Q-capability) in support learning. It enhances conventional Q-advancing by empowering more complicated state-activity portrayals and dealing with high-layered input spaces, like pictures.
81. What is the contrast between on-strategy and off-approach support learning techniques?
- • On-approach support learning techniques gain proficiency with the worth or strategy capability in light of the information gathered by following the ongoing arrangement. Off-strategy supports learning techniques and becomes familiar with the worth or strategy capability given the information gathered by following an alternate strategy (e.g., an irregular arrangement or a past strategy).
82. What is the distinction between the sans model and model-based support learning strategies?
- • The Sans model supports learning techniques that straightforwardly gain the worth or strategy capability of a fact without expressly displaying the elements of the climate. Model-based support learning techniques gain proficiency with a model of the climate (change elements and prize capability) and use it to design or reproduce directions for navigation.
83. What is profound support realizing, and how can it consolidate profound learning with support learning?
- • Profound support learning is a mix of profound learning and support realizing, where profound brain networks are utilized to rough esteem capabilities, strategy capabilities, or both in support learning. It empowers gaining complex arrangements and worth capabilities from high-layered input spaces, like pictures or crude sensor information.
84. What is the job of involvement replay in profound support learning, and how can it work?
- • Experience replay is a method used to work on the proficiency and security of profound support advancing by putting away and replaying previous encounters (changes) from a specialist's memory during preparation. It helps break the worldly relationships in the information and empowers the specialist to gain from assorted encounters.
85. What are a few normal difficulties or limits of profound support learning?
- • Test failure (requiring a lot of information), investigation double-dealing compromise, versatility to high-layered and consistent activity spaces, shakiness and combination issues in preparing profound brain organizations, and trouble in taking care of fractional perceptibility and long-haul conditions.
86. What is antagonistic AI, and how can it work?
- • Ill-disposed AI is a field of study that investigates the weaknesses of AI models to antagonistic assaults or noxious information sources intended to beguile the model and produce mistaken forecasts. Antagonistic assaults can take advantage of shortcomings in model designs or prepare calculations to control model results.
87. What are antagonistic models, and how are they produced?
- • Antagonistic models are painstakingly created inputs (e.g., pictures, text) that are purposefully intended to cause misclassification or erroneous expectations by AI models. They're created by applying impalpable annoyances to genuine sources of information, taking advantage of the model's weaknesses.
88. What are a few normal kinds of ill-disposed assaults in AI?
- • white-box assaults (information on model engineering and boundaries), black-box assaults (restricted or no information on the model), avoidance assaults (bothering contributions to change forecasts), and harming assaults (controlling preparation information to think twice about execution).
89. What is the contrast between generative antagonistic organizations (GANs) and ill-disposed models?
- • Generative ill-disposed networks (GANs) are a class of generative models comprising two brain organizations (a generator and a discriminator) prepared adversarially to produce sensible examples from a given dispersion. Antagonistic models are sources of information intended to delude AI models, taking advantage of their weaknesses.
90. What are some normal protection components against antagonistic assaults in AI?
- • Ill-disposed preparing (integrating antagonistic models during model preparation), powerful enhancement (streamlining models for most pessimistic scenario situations), cautious refining (preparing models on smoothed or refined renditions of the information), and info preprocessing (distinguishing and sifting through antagonistic models).
91. What is logical artificial intelligence (XAI), and for what reason is it significant in AI and simulated intelligence?
- • Reasonable computer-based intelligence (XAI) expects to pursue AI models, and their choices are interpretable and justifiable to people. It's significant for building trust in simulated intelligence frameworks, guaranteeing responsibility, and distinguishing predispositions or mistakes in model expectations.
92. What are a few normal strategies for making sense of AI models?
- • Highlight significance examination (e.g., stage significance, SHAP values), fractional reliance plots, individual contingent assumption (ICE) plots, neighborhood interpretable model-freethinker clarifications (LIME), and model-explicit interpretability techniques (e.g., choice tree perception, consideration components).
93. What is the job of reasonableness and predisposition moderation strategies in AI and artificial intelligence?
- • Reasonableness and predisposition alleviation procedures plan to address inclinations and guarantee decency in AI models and simulated intelligence frameworks, especially in delicate spaces like money, medical services, and law enforcement. These procedures incorporate reasonableness, mindful calculations, predisposition discovery and amendment, and various delegated procedures for preparing information.
94. What are a few normal difficulties or restrictions on decency and predisposition relief in AI and man-made intelligence?
- • Definition and estimation of decency, compromises between various reasonableness models, absence of variety and portrayal in preparing information, potentially negative side-effects of predisposition moderation strategies, and moral ramifications of direction.
95. What is the distinction between logic and interpretability in AI and computer-based intelligence?
- • Reasonableness alludes to the capacity to give clarifications or legitimizations to demonstrate forecasts or choices in a human-justifiable configuration. Interpretability alludes to the simplicity of understanding the interior functions and systems of a model, including how sources of information are changed into yields.
96. What are a few normal methods for working on the interpretability of AI models?
- • Improving model structures (e.g., utilizing straight models, choice trees), highlight designing (making interpretable elements), model refining (preparing easier substitute models), and utilizing interpretable model-explicit methods (e.g., choice tree representation, rule extraction).
97. What is the job of area information in AI and simulated intelligence projects?
- • Space information alludes to aptitude or comprehension of the particular business, field, or branch of knowledge applicable to the information being examined. It's significant in AI and simulated intelligence projects for deciphering results, recognizing significant examples, and producing noteworthy bits of knowledge that line up with space-specific objectives and requirements.
98. What are a few normal procedures for coordinating space information into AI models?
- • Highlight designing (making space explicit elements), consolidating area explicit imperatives (e.g., straight requirements in improvement issues), area explicit preprocessing (e.g., taking care of missing qualities or anomalies in light of space information), and utilizing space explicit assessment measurements.
99. In machine learning, what distinguishes supervised from unsupervised domain adaptation?
- Using supervision from the source domain, supervised domain adaptation entails modifying a model from a source domain with labelled data to a target domain with unlabeled data. Unsupervised domain adaptation is modifying a model using only unsupervised learning methods in the absence of labelled data in the target domain.
100. What are some popular methods used in machine learning for domain adaptation?
- • Domain adversarial training (aligning feature distributions across domains), feature augmentation (adding domain-specific features), instance reweighting (changing sample weights based on domain differences), and transfer learning (leveraging pre-trained models or knowledge from related domains).
Machine Learning & Cloud Interview Questions And Answers
1. What is AI?
- • AI is a subset of computerized reasoning that includes the improvement of calculations and models that permit PCs to gain from information and settle on forecasts or choices without being expressly modified.
2. What are the principal kinds of AI?
- • The primary kinds of AI are directed learning, solo learning, and support learning.
3. What is Distributed computing?
- • Distributed computing alludes to the conveyance of registering administrations over the web, including capacity, handling power, and programming applications, on a pay-more-only-as-costs arise premise.
4. How can Machine Learn connected with Distributed computing?
- • Distributed computing gives the foundation and assets expected to store and handle huge volumes of information expected for preparing AI models. It offers adaptability, adaptability, and cost viability for running AI responsibilities.
5. What are some famous cloud stages for running AI responsibilities?
- • Famous cloud stages for running AI jobs incorporate Amazon Web Administrations (AWS), Microsoft Purplish Blue, Google Cloud Stage (GCP), and IBM Cloud.
6. What are the upsides of involving cloud stages for AI?
- • Benefits incorporate versatility (capacity to deal with enormous datasets and register assets on request), adaptability (admittance to a great many administrations and devices), cost-viability (pay-more only as costs arise evaluating model), and simplicity of organization and the board.
7. What is Amazon SageMaker, and how could it be utilized for AI?
- • Amazon SageMaker is a completely overseen administration given by AWS to build, prepare, and send AI models at scale. It improves the AI work process by giving coordinated instruments and foundation.
8. What is Google Cloud's artificial intelligence Stage, and how could it be utilized for AI?
- • Google Cloud man-made intelligence Stage is a set-up of cloud administrations given by Google Cloud Stage to build, prepare, and convey AI models. It offers highlights like oversaw Jupyter notepads, robotized AI, and versatile preparation and organization framework.
9. What is Sky Blue AI, and how could it be utilized for AI?
- • Purplish Blue AI is a cloud-based help given by Microsoft Sky Blue to build, prepare, and convey AI models. It offers highlights like mechanized AI, model organization to Sky Blue Kubernetes Administration (AKS), and reconciliation with other Sky Blue administrations.
10. What are some normal AI undertakings that can be performed utilizing cloud stages?
- • Normal errands incorporate information preprocessing and cleaning, including designing, model preparation and assessment, hyperparameter tuning, model sending, and observing.
11. What is AutoML, and how can it improve on AI work processes?
- • AutoML (Robotized AI) alludes to the method involved with mechanizing the start-to-finish cycle of applying AI to true issues, including information preprocessing, highlight designing, model determination, hyperparameter tuning, and arrangement. It improves AI work processes by diminishing the requirement for manual mediation and skill.
12. How truly does distributed computing work with cooperative AI projects?
- • Distributed computing gives cooperative elements, for example, shared information capacity, rendition control frameworks, cooperative coding conditions, and access control systems, permitting various clients to team up on AI projects consistently.
13. What are a few prescribed procedures for conveying AI models on cloud stages?
- • Best practices incorporate containerization (e.g., utilizing Docker compartments), forming models and conditions, checking model execution and float, setting up computerized testing and persistent reconciliation/consistent sending (CI/Album) pipelines, and guaranteeing security and consistency.
14. What is serverless figuring, and how could it be utilized in AI?
- • Serverless processing, otherwise called Capability as a Help (FaaS), permits designers to run code in light of occasions without overseeing the server framework. In AI, serverless registering can be utilized for conveying and scaling deduction endpoints, dealing with ongoing expectations, and executing cluster handling undertakings.
15. What are a few difficulties of running AI jobs on cloud stages?
- • Challenges incorporate overseeing costs and streamlining asset utilization, guaranteeing information protection and security, managing seller secure in, tending to execution and dormancy issues, and remaining refreshed with quickly developing cloud administrations and advances.
16. How truly does distributed computing uphold dispersed AI calculations?
- •Distributed computing gives appropriate registering assets and systems (e.g., Apache Flash, TensorFlow disseminated) that permit AI calculations to be parallelized and executed across numerous hubs or cases, empowering quicker preparation and handling of huge datasets.
17. What is the distinction between on-premises and cloud-based AI frameworks?
- • On-premises AI framework alludes to the foundation that is possessed, worked, and kept up with by an association inside its own server farms or offices. Cloud-based AI framework alludes to the foundation given by cloud specialist organizations over the web, open on a pay-more-only-as-costs arise premise.
18. How really does distributed computing uphold enormous information handling in AI?
- • Distributed computing gives adaptable capacity and handling arrangements (e.g., Amazon S3, Google BigQuery, Sky Blue Information Lake) that permit AI calculations to examine and get experiences from huge volumes of organized and unstructured information, usually alluded to as large information.
19. What are a few contemplations for choosing a cloud stage for AI projects?
- • Contemplations incorporate the accessibility of AI administrations and instruments, estimating and cost design, versatility and execution, security and consistency highlights, joining with existing framework and devices, and seller backing and notoriety.
20. How truly does distributed computing uphold constant AI applications?
- • Distributed computing gives constant information web-based and handling administrations (e.g., Amazon Kinesis, Google Cloud Bar/Sub, Sky blue Occasion Center points) that empower AI models to get and deal with information streams progressively, settling on immediate forecasts or choices.
21. What are a few systems for improving expenses while running AI jobs on cloud stages?
- • Procedures incorporate rightsizing register assets, utilizing spot occasions or preemptible VMs for non-basic responsibilities, utilizing held occurrences or serious use limits for unsurprising jobs, improving information stockpiling and move costs, and executing cost observing and cautioning.
22. What are some security contemplations for conveying AI models on cloud stages?
- • Security contemplations remember information encryption for travel and very still, access control and confirmation components, network seclusion and division, consistency with industry guidelines (e.g., GDPR, HIPAA), and customary security reviews and weakness appraisals.
23. How really does distributed computing uphold edge registering in AI?
- • Distributed computing gives edge figuring arrangements that permit AI models to be sent and executed nearer to the information sources or end-clients, decreasing inertness and transmission capacity utilization. Edge registering is especially valuable for applications calling for ongoing surmising or low-dormancy reactions.
24. What is the contrast between a confidential cloud, a public cloud, and a mixture cloud?
- • A confidential cloud is a cloud foundation devoted to a solitary association and facilitated on-premises or in an outsider server farm. A public cloud is a cloud foundation given by an outsider cloud specialist co-op and available over the web to different clients. A half-and-half cloud is a blend of private and public cloud conditions, permitting responsibilities to be sent across the two conditions.
25. How truly does distributed computing uphold model arrangement and serving in AI?
- • Distributed computing offers oversaw types of assistance and stages (e.g., Amazon SageMaker, Google Cloud computer-based intelligence Stage, Purplish blue AI) for conveying, serving, and overseeing AI models at scale. These administrations handle foundation provisioning, model sending, checking, and scaling consequently.
26. What is serverless machine learning, and how does it differ from traditional machine learning deployment?
- • Serverless machine learning refers to the deployment of machine learning models using serverless computing platforms, where the infrastructure management is abstracted away from the user. It differs from traditional machine learning deployment by eliminating the need to provision and manage servers, enabling automatic scaling and cost optimization.
27. What are some best practices for securing machine learning models deployed on cloud platforms?
- • Best practices include encrypting model artifacts and data at rest and in transit, implementing access controls and authentication mechanisms, monitoring model usage and access patterns, regularly updating and patching models, and conducting security audits and penetration testing.
28. What is Kubernetes, and how is it used in machine learning deployments on cloud platforms?
- • Kubernetes is an open-source container orchestration platform used for automating deployment, scaling, and management of containerized applications. In machine learning deployments on cloud platforms, Kubernetes is used to deploy and manage scalable and reliable inference endpoints for serving machine learning models.
29. What is the difference between batch processing and real-time processing in machine learning workflows?
- • Batch processing involves processing data in large, discrete batches or chunks, typically collected over a period of time, and performing computations on them. Real-time processing, on the other hand, involves processing data as soon as it becomes available, usually with low latency and near-instantaneous response times.
30. What is the role of cloud-based data storage solutions in machine learning workflows?
- • Cloud-based data storage solutions (e.g., Amazon S3, Google Cloud Storage, Azure Blob Storage) provide scalable and durable storage for storing large volumes of structured and unstructured data used in machine learning workflows, including datasets, model artifacts, and log files.
31. What are some considerations for selecting cloud-based data storage solutions for machine learning projects?
- • Considerations include scalability and performance, durability and availability, data transfer and access costs, integration with machine learning tools and platforms, security features (e.g., encryption, access controls), and compliance with regulatory requirements.
32. What is data preprocessing, and why is it important in machine learning?
- • Data preprocessing refers to the process of cleaning, transforming, and preparing raw data for machine learning tasks. It's important for improving the quality of data, reducing noise and inconsistencies, handling missing values and outliers, and creating features that are informative for machine learning models.
33. How does cloud computing support distribute data preprocessing in machine learning workflows?
- • Cloud computing provides distributed computing frameworks and services (e.g., Apache Spark, Google Dataflow, Azure Databricks) that allow data preprocessing tasks to be parallelized and executed across multiple nodes or instances, enabling faster processing of large datasets.
34. What is model serving, and how does it differ from model training in machine learning workflows?
- • Model serving refers to the process of deploying and making trained machine learning models available for making predictions or serving requests from applications or users. It differs from model training, which involves the process of training machine learning models using labeled data to learn patterns and make predictions.
35. What are some techniques for optimizing machine learning models for deployment on cloud platforms?
- • Techniques include model quantization (reducing the precision of model weights), model pruning (removing redundant or less important model parameters), model compression (reducing model size), and model distillation (training simpler surrogate models).
36. What is the role of monitoring and logging in machine learning deployments on cloud platforms?
- • Monitoring and logging provide visibility into the performance, behavior, and health of machine learning models deployed on cloud platforms. They help detect anomalies, track usage patterns, diagnose performance issues, and ensure reliability and availability.
37. What are some common metrics for evaluating the performance of machine learning models deployed on cloud platforms?
- • Common metrics include inference latency (time taken to process a single inference request), throughput (number of inference requests processed per unit of time), accuracy (correctness of predictions), availability (uptime of inference endpoints), and cost (operational costs associated with model serving).
38. What are some techniques for scaling machine learning models deployed on cloud platforms to handle varying workloads?
- • Techniques include vertical scaling (increasing the resources allocated to individual instances), horizontal scaling (adding more instances to distribute the workload), auto-scaling (automatically adjusting resources based on demand), and serverless computing (automatically scaling based on request volume).
39. What is continuous integration and continuous deployment (CI/CD) in the context of machine learning workflows?
- • Continuous integration and continuous deployment (CI/CD) refer to the practice of automating the process of building, testing, and deploying machine learning models in a consistent and reproducible manner. It helps streamline development workflows, reduce manual errors, and improve collaboration among team members.
40. How does cloud computing support CI/CD pipelines for machine learning projects?
- • Cloud computing provides infrastructure and services for setting up and managing CI/CD pipelines for machine learning projects, including version control systems, automated testing frameworks, containerization platforms, and orchestration tools.
41. What are some best practices for designing CI/CD pipelines for machine learning projects on cloud platforms?
- • Best practices include versioning data, code, and model artifacts, automating testing and validation of models, containerizing models for reproducibility, automating deployment and rollback procedures, and integrating monitoring and logging for continuous feedback.
42. What is model drift, and how is it monitored in machine learning deployments on cloud platforms?
- • Model drift refers to the phenomenon where the performance of a deployed machine learning model degrades over time due to changes in the underlying data distribution or environment. It's monitored by comparing model predictions against ground truth labels or expected outcomes over time and triggering alerts when significant deviations occur.
43. What are some techniques for mitigating model drift in machine learning deployments?
- • Techniques include regular retraining of models with fresh data, implementing feedback loops for updating models based on new observations, monitoring feature distributions, and concept drift, using anomaly detection algorithms to detect drift, and implementing automated rollback mechanisms.
44. What is the role of feature engineering in machine learning, and how does it impact model performance?
- • Feature engineering involves selecting, transforming, and creating features from raw data to improve the performance of machine learning models. It impacts model performance by influencing the model's ability to learn relevant patterns and relationships from the data.
45. How does cloud computing support feature engineering in machine learning workflows?
- • Cloud computing provides scalable computing resources and distributed data processing frameworks (e.g., Apache Spark, Google Dataflow, Azure Databricks) that allow feature engineering tasks to be parallelized and executed efficiently on large datasets.
46. What are some techniques for feature selection in machine learning, and why is it important?
- • Techniques include univariate feature selection, recursive feature elimination, feature importance ranking, and model-based feature selection. Feature selection is important for reducing model complexity, improving model interpretability, and preventing overfitting.
47. What is model explainability, and why is it important in machine learning?
- • Model explainability refers to the ability to understand and interpret the decisions made by machine learning models, particularly complex or black-box models. It's important for building trust in models, understanding model behavior, and identifying biases or errors in model predictions.
48. How does cloud computing support model explainability in machine learning workflows?
- • Cloud computing provides tools and services for model explainability, including feature importance analysis, partial dependence plots, individual conditional expectation (ICE) plots, and model-specific interpretability methods (e.g., decision tree visualization, and attention mechanisms).
49. What are some techniques for improving the interpretability of machine learning models deployed on cloud platforms?
- • Techniques include using simpler and more interpretable model architectures (e.g., linear models, decision trees), feature engineering to create interpretable features, model distillation to train simpler surrogate models, and using model-specific interpretability methods.
50. What is the difference between model explainability and model transparency in machine learning?
- • Model explainability refers to the ability to understand and interpret the decisions made by machine learning models in a human-understandable format. Model transparency refers to the visibility and openness of the model's internal workings and mechanisms, including its architecture, parameters, and training process.
51. What is data preprocessing, and why is it important in machine learning?
- • Data preprocessing involves cleaning, transforming, and preparing raw data for machine learning tasks. It's important for improving data quality, reducing noise, handling missing values and outliers, and creating features that are informative for machine learning models.
52. How does cloud computing facilitate distributed data preprocessing in machine learning workflows?
- • Cloud computing provides distributed computing frameworks (e.g., Apache Spark, Google Dataflow, Azure Databricks) that allow data preprocessing tasks to be parallelized and executed across multiple nodes or instances, enabling faster processing of large datasets.
53. What is feature engineering and how does it affect the performance of ML models?
- • Feature engineering is the process of choosing, modifying, and producing features from unprocessed data in order to enhance the functionality of machine learning models. By affecting the model's capacity to extract pertinent patterns and correlations from the data, it affects model performance.
54. How does cloud computing support feature engineering in machine learning workflows?
- • Cloud computing provides scalable computing resources and distributed data processing frameworks that allow feature engineering tasks to be efficiently executed on large datasets, enabling the creation of informative features for machine learning models.
55. What is AutoML (Automated Machine Learning), and how does it simplify machine learning workflows?
- • AutoML refers to the process of automating the end-to-end process of applying machine learning to real-world problems, including data preprocessing, feature engineering, model selection, hyperparameter tuning, and deployment. It simplifies machine learning workflows by reducing the need for manual intervention and expertise.
56. How does AutoML in machine learning projects become supported by cloud computing?
- • Managed services and tools, such as Google AutoML, Azure Automated Machine Learning, and Amazon SageMaker Autopilot, are available on cloud computing platforms. These tools and services automate different steps in the machine learning workflow, such as feature engineering, model selection, data preprocessing, and hyperparameter tuning.
57. What are some advantages of using cloud-based AutoML services in machine learning projects?
- • Advantages include reduced time-to-market for machine learning solutions, lower barriers to entry for non-experts, automated optimization of machine learning pipelines, scalability for handling large datasets, and integration with existing cloud infrastructure and services.
58. What is model deployment, and why is it important in machine learning workflows?
- • Model deployment refers to the process of deploying trained machine learning models into production environments where they can make predictions or serve requests from applications or users. It's important to realize the value of machine learning models and make them accessible for real-world use cases.
59. How does cloud computing support model deployment in machine learning projects?
- • Cloud computing platforms offer managed services and platforms (e.g., Google Cloud AI Platform, Azure Machine Learning, Amazon SageMaker) for deploying, serving, and managing machine learning models at scale. These services handle infrastructure provisioning, model deployment, monitoring, and scaling automatically.
60. What are some common challenges of deploying machine learning models on cloud platforms?
- • Challenges include managing model dependencies and versioning, ensuring consistency across development and production environments, monitoring and logging model performance, handling security and compliance requirements, and integrating models with existing applications and workflows.
61. What is serverless computing, and how is it used in machine learning deployments on cloud platforms?
- • Serverless computing, also known as Function as a Service (FaaS), allows developers to run code in response to events without managing server infrastructure. In machine learning deployments, serverless computing can be used for deploying and scaling inference endpoints, handling real-time predictions, and executing batch processing tasks.
62. What are some advantages of using serverless computing for machine learning deployments?
- • Advantages include automatic scaling based on demand, cost-effectiveness (pay-per-use pricing model), reduced operational overhead (no server management), rapid deployment and iteration, and seamless integration with other cloud services and event-driven architectures.
63. What is containerization, and how is it used in machine learning deployments on cloud platforms?
- • Containerization is a method of packaging software applications and their dependencies into lightweight, portable containers that can run consistently across different environments. In machine learning deployments, containerization is used to package machine learning models and their dependencies for easy deployment and scalability.
64. What is Docker, and how is it applied to machine learning deployments using containerization?
- • Docker is an open-source platform for creating, distributing, and operating containers. Docker is used in machine learning deployments to build containers that contain libraries, dependencies, and machine learning models, allowing for consistent execution in various contexts.
65. What is Kubernetes and how is it used to machine learning deployments using container orchestration?
- • The deployment, scaling, and maintenance of containerized applications may be automated with the help of Kubernetes, an open-source container orchestration platform. Scalable and dependable inference endpoints for servicing machine learning models are deployed and managed using Kubernetes in machine learning deployments.
66. What are some advantages of using Kubernetes for container orchestration in machine learning deployments?
- • Advantages include automatic scaling based on resource usage, high availability and reliability, efficient resource utilization, simplified deployment and management of containerized applications, and support for rolling updates and self-healing capabilities.
67. What is model explainability, and why is it important in machine learning?
- • Model explainability refers to the ability to understand and interpret the decisions made by machine learning models, particularly complex or black-box models. It's important for building trust in models, understanding model behavior, and identifying biases or errors in model predictions.
68. How does cloud computing support model explainability in machine learning workflows?
- • Cloud computing provides tools and services for model explainability, including feature importance analysis, partial dependence plots, individual conditional expectation (ICE) plots, and model-specific interpretability methods (e.g., decision tree visualization, and attention mechanisms).
69. What are some techniques for improving the interpretability of machine learning models deployed on cloud platforms?
- • Techniques include using simpler and more interpretable model architectures (e.g., linear models, decision trees), feature engineering to create interpretable features, model distillation to train simpler surrogate models, and using model-specific interpretability methods.
70. What is model monitoring, and why is it important in machine learning deployments?
- • Model monitoring refers to the process of tracking and evaluating the performance and behavior of machine learning models deployed in production environments. It's important for detecting model drift, performance degradation, and anomalies, and ensuring that models continue to meet business objectives.
71. How does cloud computing support model monitoring in machine learning deployments?
- • Cloud computing platforms offer monitoring and logging services that allow developers to track model performance metrics, collect logs and events, set up alerts and notifications, and visualize performance trends over time, facilitating proactive management of machine learning models.
72. What is model drift and how is it tracked in cloud-based machine learning deployments?
- Model drift is the term used to describe the phenomena wherein a deployed machine learning model's performance deteriorates over time as a result of modifications to the environment or underlying data distribution. It is tracked over time by comparing model predictions to expected results or ground truth labels, and when notable variations from the model's predictions occur, alarms are raised.
73. What are some techniques for mitigating model drift in machine learning deployments?
- • Techniques include regular retraining of models with fresh data, implementing feedback loops for updating models based on new observations, monitoring feature distributions, and concept drift, using anomaly detection algorithms to detect drift, and implementing automated rollback mechanisms.
74. What are some security considerations for deploying machine learning models on cloud platforms?
- • Security considerations include data encryption in transit and at rest, access control and authentication mechanisms, network isolation and segmentation, compliance with industry regulations (e.g., GDPR, HIPAA), and regular security audits and vulnerability assessments.
75. How does cloud computing support security in machine learning deployments?
- • Cloud computing platforms offer a range of security features and services, including encryption, identity and access management (IAM), network security controls, threat detection and prevention, compliance certifications, and security monitoring and logging, to ensure the confidentiality, integrity, and availability of machine learning deployments.
76. What is data privacy, and why is it important in machine learning deployments?
- • Data privacy refers to the protection of sensitive and personal information collected and processed by machine learning models. It's important for ensuring compliance with privacy regulations, maintaining trust with users, and preventing unauthorized access or misuse of data.
77. How does cloud computing support data privacy in machine learning deployments?
- • Cloud computing platforms offer features and services for data encryption, access controls, data anonymization, and compliance certifications (e.g., GDPR, HIPAA) to help organizations protect the privacy of data used in machine learning deployments.
78. What are some best practices for securing machine learning models deployed on cloud platforms?
- • Best practices include encrypting model artifacts and data at rest and in transit, implementing access controls and authentication mechanisms, monitoring model usage and access patterns, regularly updating and patching models, and conducting security audits and penetration testing.
79. What is data governance, and why is it important in machine learning deployments?
- • Data governance refers to the management framework and processes for ensuring the availability, integrity, and security of data used in machine learning deployments. It's important for maintaining data quality, compliance with regulations, and accountability in machine learning projects.
80. How does cloud computing support data governance in machine learning deployments?
- • Cloud computing platforms offer tools and services for data governance, including data cataloging, metadata management, access controls, data lineage tracking, and compliance reporting, to help organizations establish and enforce data governance policies in machine learning deployments.
81. What is model governance, and why is it important in machine learning deployments?
- • Model governance refers to the management framework and processes for overseeing the development, deployment, and monitoring of machine learning models in production environments. It's important for ensuring model reliability, accountability, and compliance with regulatory requirements.
82. How does cloud computing support model governance in machine learning deployments?
- • Cloud computing platforms offer tools and services for model governance, including version control systems, model repositories, model deployment pipelines, model performance monitoring, and audit trails, to help organizations establish and enforce model governance policies in machine learning deployments.
83. What are some techniques for optimizing machine learning models for deployment on cloud platforms?
- • Techniques include model quantization (reducing the precision of model weights), model pruning (removing redundant or less important model parameters), model compression (reducing model size), and model distillation (training simpler surrogate models).
84. What are some considerations for selecting a cloud platform for machine learning projects?
- • Considerations include the availability of machine learning services and tools, pricing and cost structure, scalability and performance, security and compliance features, integration with existing infrastructure and tools, and vendor support and reputation.
85. What is model serving, and how does it differ from model training in machine learning workflows?
- • Model serving refers to the process of deploying trained machine learning models into production environments where they can make predictions or serve requests from applications or users. It differs from model training, which involves the process of training machine learning models using labeled data to learn patterns and make predictions.
86. What is model monitoring, and why is it important in machine learning deployments?
- • Model monitoring refers to the process of tracking and evaluating the performance and behavior of machine learning models deployed in production environments. It's important for detecting model drift, performance degradation, and anomalies, and ensuring that models continue to meet business objectives.
87. How does cloud computing support model monitoring in machine learning deployments?
- • Cloud computing platforms offer monitoring and logging services that allow developers to track model performance metrics, collect logs and events, set up alerts and notifications, and visualize performance trends over time, facilitating proactive management of machine learning models.
88. What is continuous integration and continuous deployment (CI/CD) in the context of machine learning workflows?
- • Continuous integration and continuous deployment (CI/CD) refers to the practice of automating the process of building, testing, and deploying machine learning models in a consistent and reproducible manner. It helps streamline development workflows, reduce manual errors, and improve collaboration among team members.
89. How does cloud computing support CI/CD pipelines for machine learning projects?
- • Cloud computing provides infrastructure and services for setting up and managing CI/CD pipelines for machine learning projects, including version control systems, automated testing frameworks, containerization platforms, and orchestration tools.
90. What are some best practices for designing CI/CD pipelines for machine learning projects on cloud platforms?
- • Best practices include versioning data, code, and model artifacts, automating testing and validation of models, containerizing models for reproducibility, automating deployment and rollback procedures, and integrating monitoring and logging for continuous feedback.
91. What is model explainability, and why is it important in machine learning?
- • Model explainability refers to the ability to understand and interpret the decisions made by machine learning models, particularly complex or black-box models. It's important for building trust in models, understanding model behavior, and identifying biases or errors in model predictions.
92. How does cloud computing support model explainability in machine learning workflows?
- • Cloud computing provides tools and services for model explainability, including feature importance analysis, partial dependence plots, individual conditional expectation (ICE) plots, and model-specific interpretability methods (e.g., decision tree visualization, and attention mechanisms).
93. What are some techniques for improving the interpretability of machine learning models deployed on cloud platforms?
- • Techniques include using simpler and more interpretable model architectures (e.g., linear models, decision trees), feature engineering to create interpretable features, model distillation to train simpler surrogate models, and using model-specific interpretability methods.
94. What is model fairness, and why is it important in machine learning?
- • Model fairness refers to the fairness and impartiality of machine learning models in their predictions and decisions, particularly with regard to sensitive attributes such as race, gender, or ethnicity. It's important for ensuring ethical and unbiased outcomes and avoiding discrimination or harm to individuals or groups.
95. How does cloud computing support model fairness in machine learning workflows?
- • Cloud computing provides tools and services for assessing and mitigating biases in machine learning models, including fairness-aware machine learning algorithms, bias detection and mitigation techniques, and model evaluation frameworks that measure fairness across different demographic groups.
96. What are some challenges of achieving model fairness in machine learning deployments?
- • Challenges include identifying and measuring biases in training data, mitigating biases without sacrificing model performance, ensuring transparency and accountability in model decisions, and addressing ethical and legal implications of biased predictions or decisions.
97. What is edge computing, and how is it used in machine learning deployments?
- • Edge computing refers to the practice of processing data closer to its source or destination, typically at the network edge or on end-user devices, rather than in centralized data centers or cloud environments. In machine learning deployments, edge computing is used for deploying and executing machine learning models on devices with limited connectivity or latency requirements.
98. How does cloud computing support edge computing in machine learning deployments?
- • Cloud computing platforms offer edge computing solutions that allow machine learning models to be deployed and executed closer to the data sources or end-users, reducing latency and bandwidth usage. Edge computing is particularly useful for applications requiring real-time inference or low-latency responses.
99. What are some advantages of using edge computing for machine learning deployments?
- • Advantages include reduced latency for real-time inference, improved privacy and security by processing data locally, reduced bandwidth usage by filtering and preprocessing data at the edge, and increased resilience against network failures or disruptions.
100. What is federated learning, and how is it used in machine learning deployments?
- • Federated learning is a distributed machine learning approach where multiple devices or edge nodes collaboratively train a shared machine learning model while keeping data decentralized and localized. It's used in scenarios where data privacy, security, or bandwidth constraints prevent centralized model training.
Certified Ethical Hacker CEH v11 Interview Questions And Answers
1. What is hacking that is ethical?
- • In order to increase security, systems are tested for vulnerabilities through ethical hacking.
2. Explain the difference between White Hat, Black Hat, and Gray Hat Hackers.
- • White Hat: Ethical hackers who work legally to improve security.
- • Black Hat: Hackers who exploit systems for malicious purposes.
- • Gray Hat: Hackers who may use their skills for both ethical and unethical purposes.
3. What is Footprinting in Ethical Hacking?
- • Information about a target system or network is gathered as part of the footprinting process.
4. List the stages involved in ethical hacking.
- • Track clearing, scanning, gaining and retaining access, and reconnaissance.
5. What is Social Engineering in the context of Ethical Hacking?
- • Social Engineering involves manipulating individuals to divulge confidential information.
6. Explain the concept of Vulnerability Assessment.
- • Vulnerability assessment entails locating and measuring weak points in a network or system.
7. Define a firewall.
- • A firewall is a tool for network security that keeps an eye on and regulates both inbound and outbound network traffic.
8. Name a common port used for SSH.
- • Port 22.
9. What is SQL Injection?
- • SQL Injection is a type of code injection when malicious SQL statements are inserted into input fields to exploit data-driven systems.
10. Explain the concept of Phishing.
- • Phishing is a cyber-attack where attackers pose as legitimate entities to deceive individuals into providing sensitive information.
11. What is the purpose of a Proxy Server?
- • A Proxy Server acts as an intermediary between clients and servers, providing various security and performance benefits.
12. What does Cross-Site Scripting (XSS) mean?
- • Cross-Site Scripting is a flaw that lets hackers insert dangerous scripts onto other users' web sites.
13. What is a Brute Force Attack?
- • A Brute Force Attack is a trial-and-error method used by attackers to guess login credentials or encryption keys.
14. Explain the concept of Network Sniffing
- • Network Sniffing involves capturing and analyzing data packets transmitted over a network.
15. What is ARP Spoofing?
- • ARP Spoofing is a technique used by attackers to associate their MAC address with the IP address of another device on a local network.
16. What is the purpose of a Honeypot?
- •A Honeypot is a decoy system designed to lure attackers and gather information about their techniques and motivations.
17. Define Denial of Service (DoS) Attack.
- • A Denial of Service Attack is an attempt to make a machine or network resource unavailable to its intended users.
18. What is the difference between Penetration Testing and Vulnerability Assessment?
- • Penetration Testing attempts to exploit vulnerabilities to determine the effectiveness of security measures, while Vulnerability Assessment focuses on identifying and quantifying vulnerabilities.
19. What is the purpose of an Intrusion Detection System (IDS)?
- • An intrusion detection system keeps an eye out for hostile activity and policy infractions on a network or system.
20. Describe the idea behind cryptography.
- • The application of secure communication methods to prevent unauthorised access to data is known as cryptography.
21. What distinguishes symmetric encryption from asymmetric encryption?
- • While Asymmetric Encryption employs separate keys for encryption and decryption, Symmetric Encryption uses the same key for both processes.
22. What is a Man-in-the-Middle (MitM) Attack?
- • An attacker can intercept and perhaps modify communication between two parties without the victims' awareness in a Man-in-the-Middle Attack.
23. What is a rootkit?
- • A rootkit is a group of malicious programmes that, while remaining undetected, allow unauthorised users to access a computer or network.
24. What is the purpose of Hashing?
- • Hashing is the process of converting data into a fixed-size string of characters, typically for secure storage or verification.
25. Define Keylogger.
- • A Keylogger is a type of malware or hardware device that records keystrokes entered by a user.
26. Explain the concept of Steganography.
- • Steganography is the practice of concealing messages or data within non-secret data or media.
27. What is the purpose of Network Access Control (NAC)?
- • Network Access Control restricts access to a network based on policies and the security status of devices attempting to connect.
28. Define DNS Spoofing.
- • DNS Spoofing is a type of attack where DNS records are altered to redirect traffic to a malicious website.
29. What is a Reverse Proxy?
- • A Reverse Proxy is a server that accesses one or more servers to retrieve resources on behalf of a client.
30. Explain the concept of Zero-Day Exploit.
- • A Zero-Day Exploit is a vulnerability in software or hardware that is exploited by attackers before the vendor releases a patch or update.
31. What is a Certificate Authority (CA)?
- • A Certificate Authority is an entity that issues digital certificates used to verify the identity of individuals, organizations, or devices.
32. Define Data Encryption Standard (DES).
- • Data Encryption Standard is a symmetric-key algorithm used for encrypting and decrypting electronic data.
33. What is a WAF (Web Application Firewall)?
- • A Web Application Firewall is a security device or service that monitors and filters HTTP requests between a web application and the internet.
34. Explain the concept of Packet Filtering.
- • Packet Filtering is the process of selectively blocking or allowing network traffic based on predetermined criteria.
35. What is the purpose of Multi-Factor Authentication (MFA)?
- • Multi-Factor Authentication adds an extra layer of security by requiring users to provide multiple forms of identification to access a system or application.
36. Describe buffer overflow.
- • A programme can create a buffer overflow vulnerability by writing more data to a buffer than it can handle. This can result in system crashes or unauthorised access.
37. What is the purpose of Digital Forensics?
- • Digital Forensics involves collecting, preserving, and analyzing digital evidence for use in investigations or legal proceedings.
38. Describe the idea behind network segmentation.
- • Network segmentation: To increase security and performance, a network is divided into smaller, more isolated pieces.
39. How do you distinguish between authorization and authentication?
- • Authorization establishes the access rights and privileges provided to that identity, whereas authentication confirms the identity of a user or system.
40. Define VPN (Virtual Private Network).
- • A Virtual Private Network extends a private network across a public network, allowing users to securely transmit data as if they were directly connected to the private network.
41. What is the purpose of a SIEM (Security Information and Event Management) system?
- • A SIEM system collects, analyzes, and correlates security event data from various sources to identify and respond to security threats.
42. Explain the concept of Endpoint Security.
- • Endpoint Security refers to the protection of endpoints (devices such as computers, smartphones, and tablets) from cybersecurity threats.
43. What is the purpose of Data Masking?
- • Data Masking is a data protection technique that replaces sensitive information with fictitious data or symbols to prevent unauthorized access.
44. Give a definition of Network Address Translation (NAT).
- • The process of changing network address information in packet headers while they are being transmitted over a network is known as "network address translation."
45. What is Security Information and Event Management's (SIEM) objective?
- • SIEM systems offer instantaneous security warning analysis derived from both network hardware and applications.
46. Explain the concept of Secure Coding Practices.
- • Secure Coding Practices involve writing code in a way that minimizes security vulnerabilities and weaknesses.
47. What is the purpose of a Secure Shell (SSH)?
- • Secure Shell provides secure remote access and communication over an unsecured network.
48. Define the Principle of Least Privilege.
- • The Principle of Least Privilege states that users or systems should only be granted the minimum level of access or permissions necessary to perform their tasks.
49. What is the difference between Vulnerability and Exploit?
- • Vulnerability is a weakness in a system that could be exploited, while an exploit is a piece of code or technique used to take advantage of a vulnerability.
50. Define Port Scanning.
- • Port Scanning is the process of identifying open ports on a target system to determine potential vulnerabilities.
51. Describe the idea behind packet sniffing.
- • Packet sniffing is the process of recording and examining data packets that are sent over a network.
52. What is a Session Hijacking attack?
- • Session Hijacking is a type of attack where an attacker takes over a session between a client and a server to gain unauthorized access.
53. Define Wireless Encryption Protocol (WEP).
- • WEP is a security protocol used to secure wireless networks, but it is known to have significant vulnerabilities.
54. What is a Proxy Server and how does it work?
- • A Proxy Server acts as an intermediary between clients and servers, forwarding requests on behalf of the client and returning responses from the server.
55. Describe the idea of a Trojan Horse.
- • A Trojan Horse is a kind of malware that appears as trustworthy software but, when run, does bad things.
56. What is the purpose of a Password Cracking Tool?
- • A Password Cracking Tool is used to recover or bypass passwords by attempting various methods such as brute force or dictionary attacks.
57. Define Cross-Site Request Forgery (CSRF).
- • • CSRF is a type of attack where an attacker tricks a user into performing actions on a web application without their knowledge or consent.
58. Explain the concept of Network Address Translation (NAT).
- • NAT is a process that modifies network address information in packet headers while they are in transit across a network to enable communication between devices with different IP addresses.
59. What distinguishes passive reconnaissance from active reconnaissance?
- • While passive reconnaissance gathers data without directly dealing with the target, active reconnaissance uses direct interaction with the target system to obtain information.
60. Define Cryptanalysis.
- • • Cryptanalysis is the study of cryptographic systems with the goal of breaking or circumventing their security measures.
61. What is a Rogue Access Point?
- • A Rogue Access Point is an unauthorized wireless access point connected to a network without the network administrator's knowledge or consent.
62. Explain the concept of a Rainbow Table.
- • A Rainbow Table is a precomputed table used in password cracking to reverse cryptographic hash functions and find plaintext passwords.
63. What is the purpose of Network Forensics?
- • Network Forensics involves analyzing network traffic and logs to investigate security incidents or breaches.
64. Define Secure Sockets Layer (SSL) Stripping.
- • SSL Stripping is a technique used by attackers to downgrade a secure HTTPS connection to an insecure HTTP connection, allowing them to intercept sensitive information.
65. What is a Distributed Denial of Service (DDoS) Attack?
- • A DDoS Attack is an attempt to make a network or website unavailable to its intended users by overwhelming it with a large volume of traffic from multiple sources.
66. Explain the concept of a Firewall and how it works.
- • A Firewall is a network security device that monitors and controls incoming and outgoing traffic based on predetermined security rules to prevent unauthorized access.
67. Explain Key Exchange.
- • Establishing a secure communication route between parties through the safe exchange of cryptographic keys is known as Key Exchange.
68. What is a SQL Injection attack?
- • A SQL Injection attack is a type of code injection where an attacker manipulates SQL queries executed by a web application to access or modify sensitive data.
69. Explain the concept of a Web Application Firewall (WAF).
- • A Web Application Firewall is a security device or service that monitors and filters HTTP requests to protect web applications from various attacks.
70. What is the purpose of Network Access Control (NAC)?
- • Network Access Control restricts access to a network based on policies and the security status of devices attempting to connect.
71. Define Vulnerability Scanning.
- • Vulnerability Scanning is the process of identifying and assessing vulnerabilities in a system or network to prioritize and remediate them.
72. What is the difference between Penetration Testing and Red Teaming?
- • Penetration Testing simulates attacks to identify and exploit vulnerabilities, while Red Teaming involves more comprehensive and realistic simulations of adversary behavior to test an organization's overall security posture.
73. Explain the concept of a Reverse Proxy.
- • A Reverse Proxy is a server that retrieves resources on behalf of clients from one or more servers, acting as an intermediary between clients and backend servers.
74. What does Digital Rights Management (DRM) aim to achieve?
- • Digital rights management is a technique that guards against unauthorised use, distribution, and access to digital content.
75. Define Data Masking.
- • Data Masking is a data protection technique that replaces sensitive information with fictitious data or symbols to prevent unauthorized access.
76. What is the purpose of Data Loss Prevention (DLP)?
- • Data Loss Prevention is a strategy for preventing sensitive information from being accessed, used, or shared inappropriately.
77. Explain the concept of Hashing.
- • Hashing is the process of converting data into a fixed-size string of characters using a cryptographic hash function, typically for secure storage or verification.
78. Define Malware Analysis.
- • Malware Analysis is the process of dissecting and understanding malicious software to identify its functionality, origins, and potential impact.
79. What is the difference between Asymmetric and Symmetric Encryption?
- • Asymmetric Encryption uses different keys for encryption and decryption, while Symmetric Encryption uses the same key for both encryption and decryption.
80. Describe the idea of a buffer overflow.
- • A buffer overflow is a vulnerability that can result in system crashes or unwanted access when a programme writes more data to a buffer than it can handle.
81. What is the purpose of Endpoint Detection and Response (EDR)?
- • Endpoint Detection and Response is a cybersecurity technology that continuously monitors and responds to threats on endpoint devices such as computers, smartphones, and tablets.
82. What is a rootkit?
- • A rootkit is a group of malicious programmes that covertly gain unauthorised access to a computer or network.
83. What is a Honeypot and how does it work?
- • A Honeypot is a decoy system designed to lure attackers and gather information about their techniques and motivations by mimicking vulnerable systems or services.
84. Explain the concept of Brute Force Attack.
- • A Brute Force Attack is a trial-and-error method used by attackers to guess login credentials or encryption keys by systematically trying all possible combinations.
85. What is the purpose of a Certificate Authority (CA)?
- • A Certificate Authority is an entity that issues digital certificates used to verify the identity of individuals, organizations, or devices in a public key infrastructure (PKI).
86. Define Data Encryption Standard (DES).
- • Data Encryption Standard is a symmetric-key encryption algorithm used for encrypting and decrypting electronic data.
87. Describe and explain the operation of a keylogger.
- • A keylogger is a kind of virus or hardware that keeps track of user keystrokes; it is usually used to obtain private data, including credit card numbers and passwords.
88. Explain the concept of Social Engineering.
- • Social Engineering is the psychological manipulation of individuals to trick them into divulging confidential information or performing actions that compromise security.
89. What is the purpose of Security Information and Event Management (SIEM)?
- • SIEM systems provide real-time analysis of security alerts generated by network hardware and applications to identify and respond to security threats.
90. Define Zero-Day Exploit.
- • A Zero-Day Exploit is a vulnerability in software or hardware that is exploited by attackers before the vendor releases a patch or update, leaving no time for defenders to mitigate the threat.
91. What is the difference between Authentication and Authorization?
- • Authentication verifies the identity of a user or system, while Authorization determines the access rights and privileges granted to that identity.
92. Explain the concept of Network Segmentation.
- • Network Segmentation involves dividing a network into smaller, isolated segments to improve security and performance by controlling the flow of traffic between segments.
93. What is a Secure Shell (SSH) and how does it work?
- • Secure Shell is a cryptographic network protocol used for secure remote access and communication between two computers, typically used for remote administration or file transfers.
94. Define Data Loss Prevention (DLP).
- • Data Loss Prevention is a strategy for preventing sensitive information from being accessed, used, or shared inappropriately by monitoring and controlling data in motion, at rest, and in use.
95. What is the purpose of Multi-Factor Authentication (MFA)?
- • Multi-Factor Authentication adds an extra layer of security by requiring users to provide multiple forms of identification, such as passwords, biometrics, or security tokens, to access a system or application.
96. Explain the concept of Network Access Control (NAC).
- • Network Access Control restricts access to a network based on policies and the security status of devices attempting to connect, ensuring only authorized devices are granted access.
97. What is a Man-in-the-Middle (MitM) Attack?
- • A Man-in-the-Middle Attack occurs when an attacker intercepts and possibly alters communication between two parties without their knowledge or consent, allowing the attacker to eavesdrop or manipulate data.
98. Define Secure Coding Practices.
- • Secure Coding Practices involve writing code in a way that minimizes security vulnerabilities and weaknesses, following industry best practices and guidelines to mitigate common security risks.
Web Server Hacking & Network Pentesting Interview Questions And Answers
1. What is a web server?
- • A web server is software that serves web pages to users upon request over the internet.
2. What is the most common web server software?
- • Apache HTTP Server and Nginx are the most common web server software.
3. What is a vulnerability scanner?
- • A vulnerability scanner is a tool used to identify weaknesses and vulnerabilities in a system or network.
4. What is a brute force attack?
- • A brute force attack is a trial-and-error method used to gain unauthorized access to a system by trying all possible combinations of usernames and passwords.
5. Explain the concept of cross-site scripting (XSS).
- • Cross-site scripting is a type of vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users.
6. What is an attack known as a buffer overflow?
- • A buffer overflow attack happens when a programme writes more data to a buffer than it is designed to retain. This can cause the buffer to overwrite neighbouring memory addresses, which could give the attacker access to run arbitrary code.
7. What is SQL injection?
- • SQL injection is a code injection technique used to attack data-driven applications by inserting malicious SQL statements into input fields.
8. What is directory traversal?
- • Directory traversal is a vulnerability that allows attackers to access files and directories outside the web root directory.
9. What is a reverse shell?
- • A reverse shell is a shell session established by an attacker from a target machine back to the attacker's machine, allowing remote control and execution of commands.
10. Explain the concept of port scanning.
- • Port scanning is the process of identifying open ports on a target system to determine potential vulnerabilities.
11. What is a denial-of-service (DoS) attack?
- • A denial-of-service attack is an attempt to make a machine or network resource unavailable to its intended users by overwhelming it with a flood of traffic or requests.
12.What is the definition of a man-in-the-middle (MitM) attack?
- • When someone intercepts and potentially modifies communication between two parties without the other party's knowledge or approval, it's known as a man-in-the-middle attack.
13. What is network sniffing?
- • Network sniffing involves capturing and analyzing data packets transmitted over a network.
14. What is ARP poisoning?
- • ARP poisoning is a technique used by attackers to associate their MAC address with the IP address of another device on a local network, allowing them to intercept traffic intended for that device.
15. What is a vulnerability assessment?
- • Vulnerability assessment involves identifying and quantifying vulnerabilities in a system or network to prioritize and remediate them.
16. What is the purpose of a proxy server?
- • A proxy server acts as an intermediary between clients and servers, providing various security and performance benefits.
17. What is a web application firewall (WAF)?
- • A web application firewall is a security device or service that monitors and filters HTTP requests to protect web applications from various attacks.
18. What is a network intrusion detection system (NIDS)?
- • A network intrusion detection system monitors network traffic for signs of suspicious activity or potential security threats.
19. What is the difference between a vulnerability assessment and penetration testing?
- • A vulnerability assessment identifies and quantifies vulnerabilities, while penetration testing attempts to exploit those vulnerabilities to determine the effectiveness of security measures.
20. What is the purpose of banner grabbing?
- • Banner grabbing is the process of gathering information about a target system or network, often used to identify the type and version of software running on a server.
21. What is a rainbow table?
- • A rainbow table is a precomputed table used in password cracking to reverse cryptographic hash functions and find plaintext passwords.
22. What is session fixation?
- • Session fixation is a vulnerability that allows an attacker to hijack a user's session by fixing or forcing the session ID, typically through phishing or other social engineering techniques.
23. What is a SYN flood attack?
- • A SYN flood attack is a type of denial-of-service attack where an attacker sends a flood of TCP SYN packets to a target system, overwhelming its resources and making it unable to respond to legitimate requests.
24. What is a vulnerability exploit?
- • A vulnerability exploit is a piece of code or technique used to take advantage of a vulnerability in a system or application to gain unauthorized access or perform malicious actions.
25. What is the purpose of network segmentation?
- • Network segmentation involves dividing a network into smaller, isolated segments to improve security and performance by controlling the flow of traffic between segments.
26. What is a firewall and how does it work?
- • A firewall is a network security device that monitors and controls incoming and outgoing traffic based on predetermined security rules to prevent unauthorized access.
27. What is a covert channel?
- • A covert channel is a communication channel that is not intended to be detected or monitored, often used by attackers to bypass security controls and exfiltrate data.
28. What distinguishes white-box testing from black-box testing?
- • White-box testing is a testing technique in which the tester has complete knowledge of the internal operations of the system, in contrast to black-box testing, which involves the tester knowing nothing about the internal operations of the system.
29. What is port knocking?
- • Port knocking is a security technique used to hide the existence of services on a server by requiring a sequence of connection attempts on specific ports before granting access to a service.
30. What is the purpose of network access control (NAC)?
- • Network access control restricts access to a network based on policies and the security status of devices attempting to connect, ensuring only authorized devices are granted access.
31. What is a zero-day exploit?
- • A zero-day exploit is a vulnerability in software or hardware that is exploited by attackers before the vendor releases a patch or update, leaving no time for defenders to mitigate the threat.
32. How do you define packet filtering?
- • Packet filtering is the process of limiting or permitting network traffic according to preset standards, usually based on protocols, ports, and IP addresses.
33. What is the difference between encryption and hashing?
- • Encryption is the process of converting plaintext data into ciphertext using a cryptographic algorithm and a key, while hashing is the process of converting data into a fixed-size string of characters using a hash function.
34. What is a web shell?
- • A web shell is a malicious script or program uploaded to a web server to provide remote access and control to an attacker.
35. What is a honey pot?
- • A honey pot is a decoy system designed to lure attackers and gather information about their techniques and motivations by mimicking vulnerable systems or services.
36. What is a rootkit?
- • A rootkit is a collection of malicious software that provides unauthorized access to a computer or network while concealing its presence.
37. What is the purpose of network forensics?
- • Network forensics involves analyzing network traffic and logs to investigate security incidents or breaches and gather evidence for legal proceedings.
38. What is a DNS amplification attack?
- • A DNS amplification attack is a type of denial-of-service attack where an attacker exploits vulnerabilities in DNS servers to flood a target system with a large volume of DNS response traffic.
39. What is a virtual private network (VPN)?
- • A virtual private network extends a private network across a public network, allowing users to securely transmit data as if they were directly connected to the private network.
40. What is session hijacking?
- • Session hijacking is a type of attack where an attacker takes over a session between a client and a server to gain unauthorized access.
41. What is the purpose of network segmentation?
- • Network segmentation involves dividing a network into smaller, isolated segments to improve security and performance by controlling the flow of traffic between segments.
42. What is a reverse proxy?
- • A reverse proxy is a server that retrieves resources on behalf of clients from one or more servers, acting as an intermediary between clients and backend servers.
43. What distinguishes white-box testing from black-box testing?
- • White-box testing is a testing technique in which the tester has complete knowledge of the internal operations of the system, in contrast to black-box testing, which involves the tester knowing nothing about the internal operations of the system.
44. What is a zero-day exploit?
- • A zero-day exploit is a vulnerability in software or hardware that is exploited by attackers before the vendor releases a patch or update, leaving no time for defenders to mitigate the threat.
45. How does packet filtering work?
- • Packet filtering is the process of limiting or permitting network traffic according to preset standards, usually based on protocols, ports, and IP addresses.
46. How does hashing vary from encryption?
- • Hashing is the process of transforming data into a fixed-length string of characters using a hash function, whereas encryption is the process of transforming plaintext data into ciphertext using a cryptographic method and a key.
47. What is a web shell?
- • A web shell is a malicious script or program uploaded to a web server to provide remote access and control to an attacker.
48. What is a honey pot?
- • A honey pot is a decoy system designed to lure attackers and gather information about their techniques and motivations by mimicking vulnerable systems or services.
49. What is a rootkit?
- • A rootkit is a collection of malicious software that provides unauthorized access to a computer or network while concealing its presence.
50. What is the purpose of network forensics?
- • Network forensics involves analyzing network traffic and logs to investigate security incidents or breaches and gather evidence for legal proceedings.
51. How is a web application firewall (WAF) used?
- • By filtering and keeping an eye on HTTP traffic travelling between a web application and the internet, a WAF safeguards web applications.
52. Explain the concept of network segmentation.
- • Network segmentation involves dividing a network into smaller, isolated segments to improve security and performance by controlling the flow of traffic between segments.
53. What is the difference between active and passive reconnaissance?
- • Active reconnaissance involves directly interacting with the target system to gather information, while passive reconnaissance involves collecting information without directly interacting with the target.
54. What is DNS spoofing?
- • DNS spoofing is a type of attack where attackers manipulate DNS records to redirect traffic to a malicious website.
55. Define banner grabbing.
- • Banner grabbing is the process of gathering information about a target system or network, often used to identify the type and version of software running on a server.
56. What is a vulnerability exploit?
- • A vulnerability exploit is a piece of code or technique used to take advantage of a vulnerability in a system or application to gain unauthorized access or perform malicious actions.
57. What is session fixation?
- • Session fixation is a vulnerability that allows an attacker to hijack a user's session by fixing or forcing the session ID, typically through phishing or other social engineering techniques.
58. Explain the concept of ARP poisoning.
- • ARP poisoning is a technique used by attackers to associate their MAC address with the IP address of another device on a local network, allowing them to intercept traffic intended for that device.
59. What is a brute force attack?
- • A brute force attack is a trial-and-error method used to gain unauthorized access to a system by trying all possible combinations of usernames and passwords.
60. What is a distributed denial-of-service (DDoS) attack?
- • A DDoS attack is an attempt to make a machine or network resource unavailable to its intended users by overwhelming it with a flood of traffic or requests from multiple sources.
61. What is the purpose of network intrusion detection systems (NIDS)?
- • NIDS monitors network traffic for signs of suspicious activity or potential security threats.
62. Define port knocking.
- • Port knocking is a security technique used to hide the existence of services on a server by requiring a sequence of connection attempts on specific ports before granting access to a service.
63. What is a reverse shell?
- • A reverse shell is a shell session established by an attacker from a target machine back to the attacker's machine, allowing remote control and execution of commands.
64. Explain the concept of covert channels.
- • Covert channels are communication channels that are not intended to be detected or monitored, often used by attackers to bypass security controls and exfiltrate data.
65. Explain packet filtering.
- • Packet filtering is the process of limiting or permitting network traffic according to preset standards, usually based on protocols, ports, and IP addresses.
66. What is a honey pot?
- • A honey pot is a decoy system designed to lure attackers and gather information about their techniques and motivations by mimicking vulnerable systems or services.
67. How do white-box and black-box testing differ from one another?
- • White-box testing is a testing technique in which the tester has complete knowledge of the internal operations of the system, in contrast to black-box testing, which involves the tester knowing nothing about the internal operations of the system.
68. What is a zero-day exploit?
- • A zero-day exploit is a vulnerability in software or hardware that is exploited by attackers before the vendor releases a patch or update, leaving no time for defenders to mitigate the threat.
69. What is session hijacking?
- • Session hijacking is a type of attack where an attacker takes over a session between a client and a server to gain unauthorized access.
70. What is the purpose of network segmentation?
- • Network segmentation involves dividing a network into smaller, isolated segments to improve security and performance by controlling the flow of traffic between segments.
71. What is a reverse proxy?
- • A reverse proxy is a server that retrieves resources on behalf of clients from one or more servers, acting as an intermediary between clients and backend servers.
72. What is an attack known as a buffer overflow?
- • A buffer overflow attack happens when a programme writes more data to a buffer than it is designed to retain. This can cause the buffer to overwrite neighbouring memory addresses, which could give the attacker access to run arbitrary code.
73. Explain the concept of a rainbow table.
- • A rainbow table is a precomputed table used in password cracking to reverse cryptographic hash functions and find plaintext passwords.
74. What is a virtual private network (VPN)?
- • A VPN extends a private network across a public network, allowing users to securely transmit data as if they were directly connected to the private network.
75. What is a web shell?
- • A web shell is a malicious script or program uploaded to a web server to provide remote access and control to an attacker.
76. What is the purpose of network forensics?
- • Network forensics involves analyzing network traffic and logs to investigate security incidents or breaches and gather evidence for legal proceedings.
77. What is the difference between encryption and hashing?
- • Encryption is the process of converting plaintext data into ciphertext using a cryptographic algorithm and a key, while hashing is the process of converting data into a fixed-size string of characters using a hash function.
78. What is a rogue access point?
- • An unapproved wireless access point that joins a network without the network administrator's knowledge or approval is known as a rogue access point.
79. What is a rootkit?
- • A rootkit is a collection of malicious software that provides unauthorized access to a computer or network while concealing its presence.
80. What is the purpose of data loss prevention (DLP)?
- • DLP is a strategy for preventing sensitive information from being accessed, used, or shared inappropriately by monitoring and controlling data in motion, at rest, and in use.
81. What is network access control (NAC)?
- • NAC restricts access to a network based on policies and the security status of devices attempting to connect, ensuring only authorized devices are granted access.
82. What distinguishes white-box testing from black-box testing?
- • White-box testing is a testing technique in which the tester fully understands the internal workings of the system, in contrast to black-box testing, which involves the tester having no knowledge of the system's internal workings.
83. What is a zero-day exploit?
- • A zero-day exploit is a vulnerability in software or hardware that is exploited by attackers before the vendor releases a patch or update, leaving no time for defenders to mitigate the threat.
84. How does packet filtering work?
- • Packet filtering is the process of limiting or permitting network traffic according to preset standards, usually based on protocols, ports, and IP addresses.
85. What is the difference between encryption and hashing?
- • Encryption is the process of converting plaintext data into ciphertext using a cryptographic algorithm and a key, while hashing is the process of converting data into a fixed-size string of characters using a hash function.
86. What is a web shell?
- • A web shell is a malicious script or program uploaded to a web server to provide remote access and control to an attacker.
87. What is a honey pot?
- • A honey pot is a decoy system designed to lure attackers and gather information about their techniques and motivations by mimicking vulnerable systems or services.
88. What is a rootkit?
- • A rootkit is a collection of malicious software that provides unauthorized access to a computer or network while concealing its presence.
89. What is the purpose of network forensics?
- • Network forensics involves analyzing network traffic and logs to investigate security incidents or breaches and gather evidence for legal proceedings.
90. What is session hijacking?
- • Session hijacking is a type of attack where an attacker takes over a session between a client and a server to gain unauthorized access.
91. What is the purpose of network segmentation?
- • Network segmentation involves dividing a network into smaller, isolated segments to improve security and performance by controlling the flow of traffic between segments.
92. What is a reverse proxy?
- • A reverse proxy is a server that retrieves resources on behalf of clients from one or more servers, acting as an intermediary between clients and backend servers
93. Describe a buffer overflow attack.
- • A buffer overflow attack happens when a programme writes more data to a buffer than it is designed to retain. This can cause the buffer to overwrite neighbouring memory addresses, which could give the attacker access to run arbitrary code.
94. Explain the concept of a rainbow table.
- • A rainbow table is a precomputed table used in password cracking to reverse cryptographic hash functions and find plaintext passwords.
95. What is a virtual private network (VPN)?
- • A VPN extends a private network across a public network, allowing users to securely transmit data as if they were directly connected to the private network.
96. What is a web shell?
- • A web shell is a malicious script or program uploaded to a web server to provide remote access and control to an attacker.
97. What is the purpose of network forensics?
- • Network forensics involves analyzing network traffic and logs to investigate security incidents or breaches and gather evidence for legal proceedings.
98. What is the difference between encryption and hashing?
- • Encryption is the process of converting plaintext data into ciphertext using a cryptographic algorithm and a key, while hashing is the process of converting data into a fixed-size string of characters using a hash function.
99. What is an unauthorised access point?
- • An unapproved wireless access point that joins a network without the network administrator's knowledge or approval is known as a rogue access point.
100. What is the purpose of data loss prevention (DLP)?
- • DLP is a strategy for preventing sensitive information from being accessed, used, or shared inappropriately by monitoring and controlling data in motion, at rest, and in use.
Search here
categories
- FullStack Java Developer
- FullStack Python Developer
- Full Stack MEAN Developer
- Full Stack MERN Developer
- UI UX Development
- SEO Training
- Social Media Marketing
- Google Ads and PPC
- Email Marketing
- Whatsapp Marketing
- DevOps
- Linux Admin
- AWS
- Data Science with Python
- Data Analyst
- Machine Learning & AI
- Machine Learning & Cloud
- Certified Ethical Hacker CEH v11
- Web Server Hacking & Network Pentesting
About us
Welcome to Ariyath Academy, where knowledge meets innovation, and skills shape the future. As a leading software training institute, we take pride in our commitment to fostering excellence, empowering individuals, and transforming aspirations into achievements.
Resources
Work with us
×